Common Pitfalls When Deploying Akka HTTP Apps on Cloud Foundry
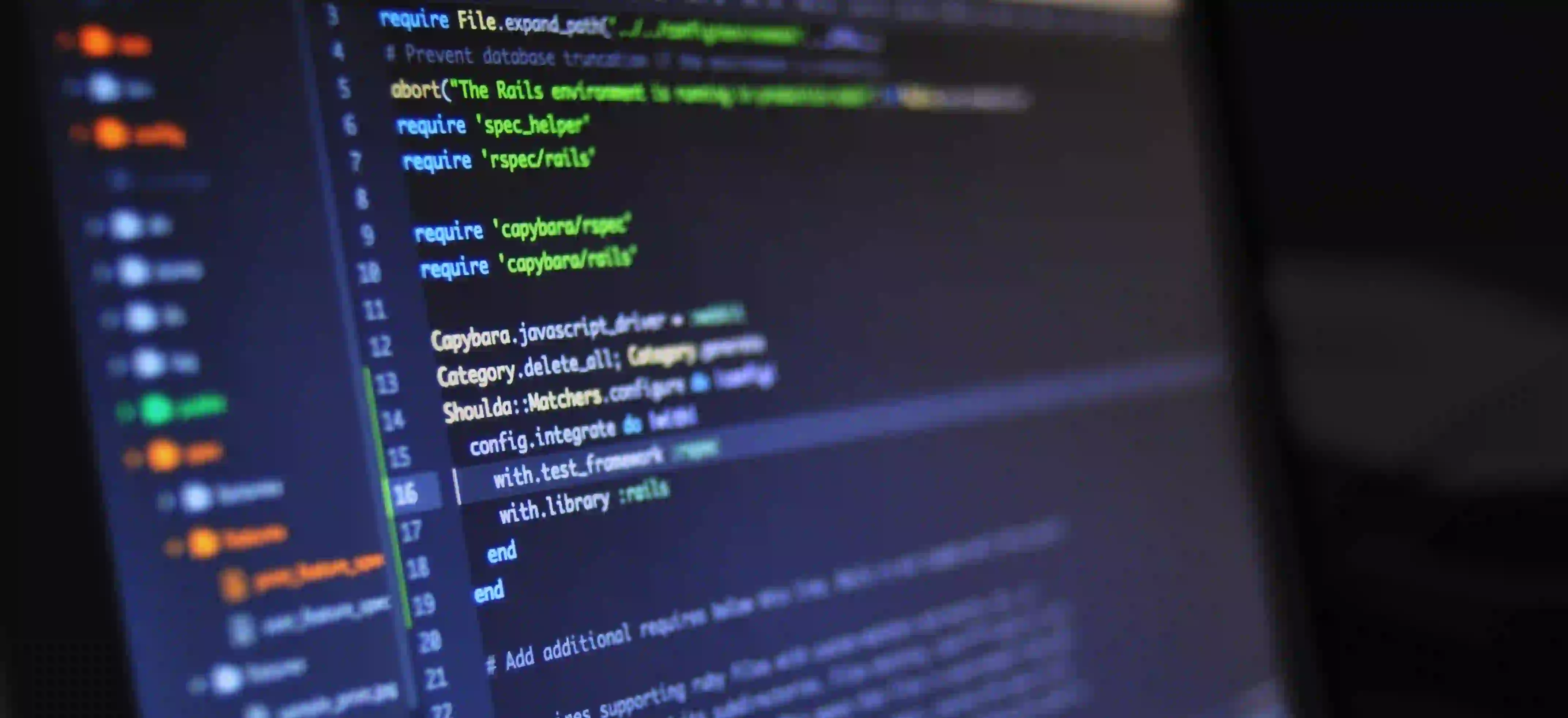
Common Pitfalls When Deploying Akka HTTP Apps on Cloud Foundry
As modern software development evolves, the demand for robust, resilient web applications grows. One powerful tool for building these applications is Akka HTTP. It provides a flexible and efficient toolkit for building RESTful APIs and is widely used in Scala and Java environments.
Moreover, deploying these applications on platforms like Cloud Foundry has become a popular choice due to its cloud-native advantages. However, during deployment, developers often encounter common pitfalls that can hinder performance and availability. In this blog post, we will explore these pitfalls, how to avoid them, and provide actionable insights for a successful deployment experience.
Understanding the Akka HTTP Ecosystem
What is Akka HTTP?
Akka HTTP is built as a lightweight and easy-to-use framework for building web services. It utilizes the actor model (part of the larger Akka toolkit) to provide non-blocking, asynchronous operations. This is crucial for handling concurrent connections efficiently, especially suited for microservices architecture.
Why Deploy on Cloud Foundry?
Cloud Foundry simplifies deployment with easy-to-use abstractions and automated scaling. Whether you are deploying a simple REST API or a complex service orchestrated with multiple APIs, Cloud Foundry optimizes the management of resources, giving you more time to focus on business logic.
Common Pitfalls During Deployment and How to Avoid Them
1. Misconfigured Routing
Routing is one of the core functionalities in any HTTP application. Akka HTTP uses routes to define how incoming requests are handled.
import akka.http.scaladsl.server.Directives._
val route =
path("hello") {
get {
complete("Hello, Akka HTTP!")
}
}
What to Avoid:
- Ensure your routes are correctly defined. A common issue occurs when the path variable or HTTP method (GET, POST, etc.) is mistakenly configured, leading to 404 errors during requests.
Best Practice: Using prefixes for versioning your APIs can help future-proof your routing scheme. For example:
val versionedRoute =
pathPrefix("api" / "v1") {
// Your routes here
}
2. Poor Resource Management
Deploying your Akka HTTP app without considering resource limits can lead to performance bottlenecks. Cloud Foundry allows you to specify resource limits for memory and CPU allocation.
What to Avoid:
- Ignoring application logs to monitor memory usage.
Best Practice:
Configure your manifest.yml
file appropriately. Here’s an example of how to set memory limits:
applications:
- name: my-akka-http-app
memory: 512M
instances: 2
You can adjust these values based on application requirements and expected load.
3. Inefficient Error Handling
Not using proper error handling mechanisms can lead to more drastic issues such as application crashes or unresponsive services.
import akka.http.scaladsl.marshallers.sprayjson.SprayJsonSupport._
import spray.json._
case class ErrorResponse(message: String)
implicit val errorResponseFormat = jsonFormat1(ErrorResponse)
val route =
path("user" / IntNumber) { id =>
get {
complete {
if (findUserById(id).isDefined) {
// Return user details
} else {
(StatusCodes.NotFound, ErrorResponse("User not found"))
}
}
}
}
What to Avoid:
- Neglecting to handle errors can lead to 500 errors or uninformative client responses.
Best Practice: Centralize error handling by creating a partial function for managing exceptions globally. This improves readability and management across your routes.
4. Lack of Health Checks
Deployed applications should have a mechanism to assess their health. Without it, scaling operations become blind, exposing your applications to available service risks.
What to Avoid:
- Forgetting to implement health checks can lead to downtime without alerts.
Best Practice: Define a route for health checks that returns a 200 response when your application is alive:
val healthCheckRoute =
path("health") {
get {
complete(StatusCodes.OK)
}
}
Cloud Foundry also provides mechanisms to check application health, and integrating these with your health endpoint ensures better monitoring.
5. Ignoring Cloud Foundry Environment Variables
In Cloud Foundry, environment variables manage application settings. Ignoring them may lead to hard-coded configurations and make your app less flexible and secure.
What to Avoid:
- Relying solely on static configuration files. This can lead to deployment issues and inconsistencies.
Best Practice: Use environment variables for configuration. For instance:
val dbUrl = sys.env.getOrElse("DATABASE_URL", "jdbc:sqlite:sample.db")
Modify your manifest.yml
to set environment variables:
env:
DATABASE_URL: "jdbc:mysql://user:password@host:3306/db"
6. Insufficient Logging
Logging is essential for monitoring applications and troubleshooting issues. Akka provides multiple logging utilities through its logging module.
What to Avoid:
- Setting up logging inconsistently can lead to missed insights and obscure debugging.
Best Practice: Choose a logging framework (like SLF4J) that integrates well with Akka. Ensure each component logs meaningful events:
import org.slf4j.LoggerFactory
val logger = LoggerFactory.getLogger(this.getClass)
logger.info("User route accessed")
Inline comments and structured log messages will enhance your ability to detect and respond to problems effectively.
The Last Word
Deploying Akka HTTP applications on Cloud Foundry can be a seamless experience if common pitfalls are avoided. Prioritizing proper routing, resource management, error handling, health checks, dynamic configurations, and effective logging ensures that your application remains resilient and efficient in the cloud environment.
For further information on effectively using Akka HTTP, check out the Akka HTTP Documentation or explore Cloud Foundry's deployment guides.
By implementing these best practices, you’ll set a solid foundation for your application's success in the cloud, ultimately leading to a more reliable service for your users. Happy coding!
This blog post emphasizes bringing clarity and actionable insights into deploying Akka HTTP applications on Cloud Foundry, combining detailed explanations with code snippets to highlight best practices and common mistakes.