Getting Started with Hazelcast: Common Setup Mistakes to Avoid
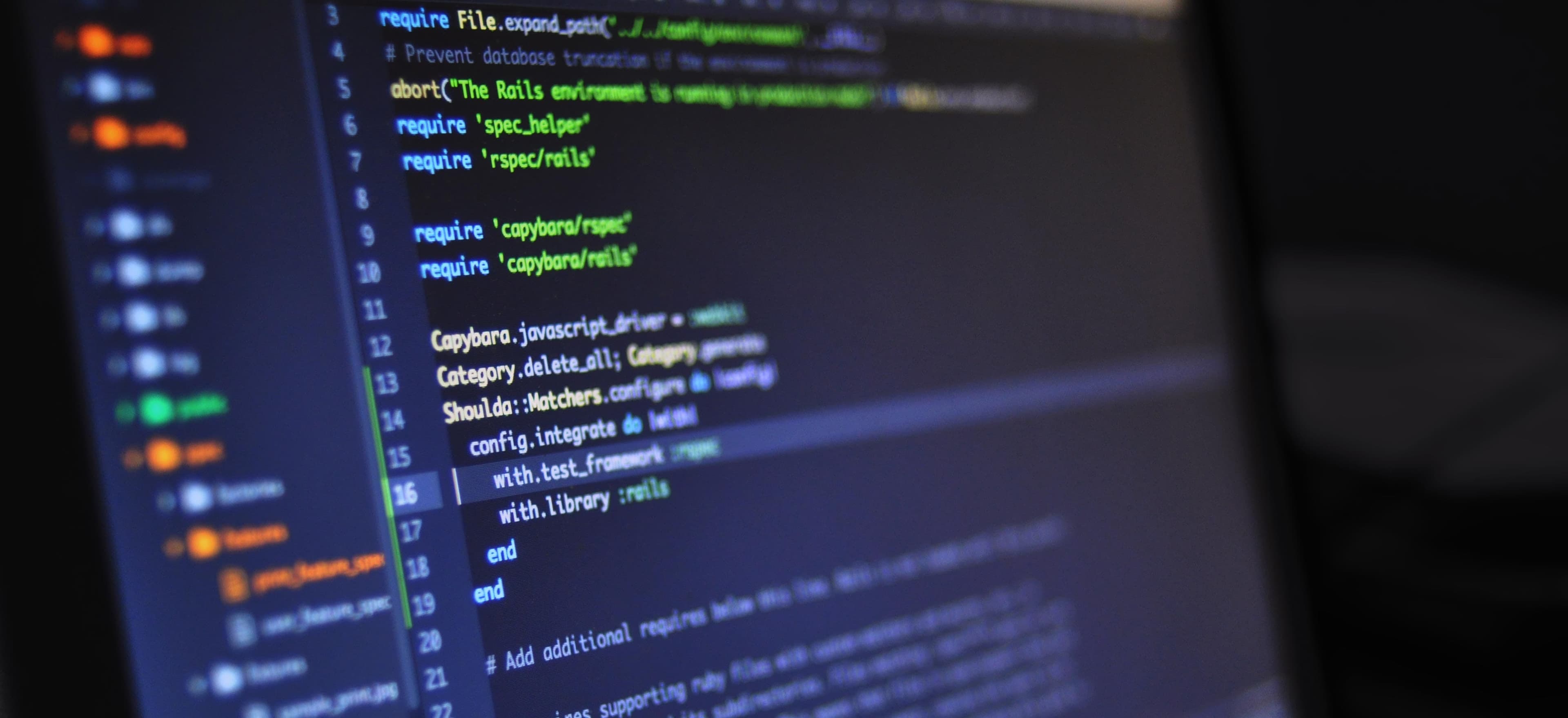
- Published on
Getting Started with Hazelcast: Common Setup Mistakes to Avoid
Hazelcast is a powerful in-memory data grid designed for high availability and scalability in Java applications. Utilizing Hazelcast effectively can significantly improve your application's performance. However, like any technology, there are common pitfalls that developers encounter during setup.
In this guide, we’ll walk through some frequent mistakes made when integrating Hazelcast into projects and how to avoid them.
Table of Contents
- What is Hazelcast?
- Common Setup Mistakes
- Ignoring Configuration Options
- Using Default Ports
- Neglecting Data Serialization
- Not Setting Up Clustering Correctly
- Failing to Monitor the Cluster
- Best Practices for Hazelcast Setup
- Conclusion
What is Hazelcast?
Hazelcast is an open-source in-memory data grid that allows organizations to store and process data in a decentralized manner. It combines the benefits of distributed computing with a simplified API for Java. This makes it easy to scale applications horizontally, manage distributed state, and enable real-time data processing.
For more foundational knowledge, refer to the official Hazelcast documentation.
Common Setup Mistakes
1. Ignoring Configuration Options
One of the most significant mistakes is overlooking the wide array of configuration options that Hazelcast provides. By default, Hazelcast operates with basic settings that may not be adequate for your specific use case.
Example Configuration:
<hazelcast>
<network>
<port>5701</port>
<join>
<multicast enabled="false"/>
<tcp-ip enabled="true">
<member>192.168.1.100:5701</member>
<member>192.168.1.101:5701</member>
</tcp-ip>
</join>
</network>
</hazelcast>
Why this matters: Customizing your configuration according to your application's needs enhances performance, security, and manageability. Hazards like insufficient timeout settings can lead to dropped connections and data inconsistency.
2. Using Default Ports
Many developers utilize default ports and IP addresses. While convenient, this practice can lead to conflicts and security vulnerabilities.
To Change Ports:
Configure your hazelcast.xml
file to use a different port:
<port>5702</port>
Why this matters: Setting custom ports based on your networking infrastructure can prevent service interruptions, allowing your application to run smoothly. It also adds a layer of security, as attackers tend to target default ports.
3. Neglecting Data Serialization
Serialization is crucial when it comes to distributed computing. If your data types are not serialized correctly, you may encounter compatibility issues.
Java Serialization Example:
import java.io.Serializable;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters
}
Why this matters:
Using Java’s built-in serialization can work, but if you want to optimize performance and maintain control over versioning, consider using Hazelcast’s IdentifiedDataSerializable
interface and implementing its methods.
4. Not Setting Up Clustering Correctly
Hazelcast is designed for distributed applications, and its clustering features are critical. Misconfiguring clustering can lead to data loss or unresponsiveness.
Joining Clusters:
Config config = new Config();
config.getNetworkConfig().getJoin().getMulticastConfig().setEnabled(false);
config.getNetworkConfig().getJoin().getTcpIpConfig().setEnabled(true)
.addMember("192.168.1.100:5701");
HazelcastInstance hz = Hazelcast.newHazelcastInstance(config);
Why this matters: By correctly setting up the cluster, including the TCP/IP settings, you ensure that all instances communicate efficiently, promoting data availability and reduced latency.
5. Failing to Monitor the Cluster
After setup, developers often neglect monitoring their Hazelcast cluster. Without monitoring, you cannot effectively manage performance or troubleshoot issues.
Using Hazelcast Management Center:
Deploying the Management Center provides real-time insights into cluster performance:
- Monitor CPU and memory usage.
- Track data movement and partition states.
- Check for security issues.
Why this matters: Monitoring helps you make informed decisions about scaling your cluster and addressing issues before they impact users.
Best Practices for Hazelcast Setup
To avoid the pitfalls mentioned above, let's discuss best practices.
- Customize Configuration: Leverage Hazelcast's configuration options extensively to suit your environment.
- Implement Custom Serialization: Optimize data serialization for performance; consider Portable Serialization for efficiency.
- Establish Robust Monitoring: Use the Hazelcast Management Center for continuous monitoring and better insights.
- Regularly Backup Data: Implement a backup strategy for critical data to prevent loss during configuration changes or updates.
- Test Clustering: Conduct thorough tests in a staging environment to ensure that your clustering setup performs as expected.
The Bottom Line
Hazelcast offers incredible benefits for distributed systems, but like any tool, it requires attention to configuration and management. By avoiding common setup mistakes, you enhance the reliability, performance, and security of your applications.
Implement the suggested configurations, practices, and continuous monitoring to make your Hazelcast integration smooth and efficient. For further reading, delve into the official Hazelcast documentation for an in-depth understanding.
Now, go ahead and harness the power of Hazelcast successfully! Happy coding!