5 Common Mistakes That Make Java Code Vulnerable
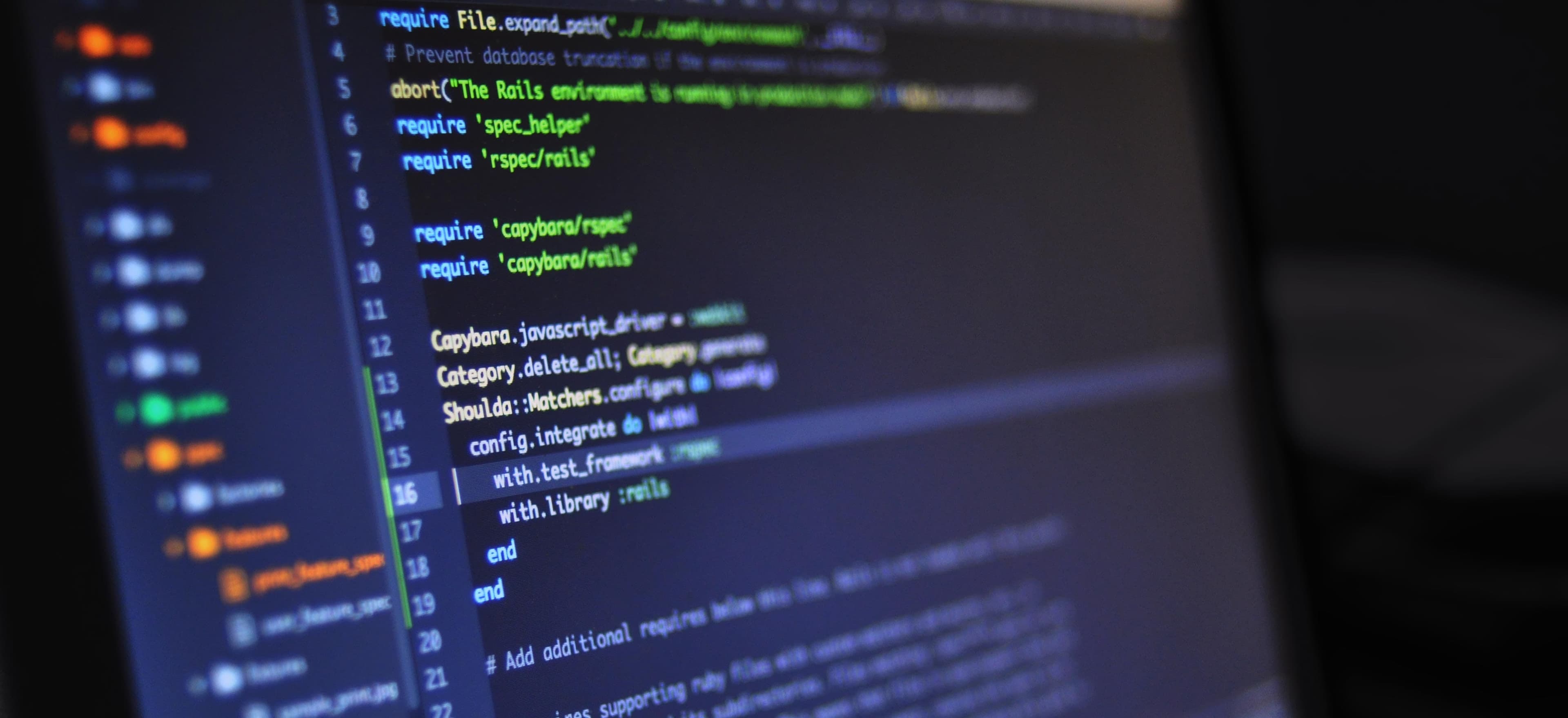
- Published on
5 Common Mistakes That Make Java Code Vulnerable
Java is a powerful and versatile programming language that is widely used in enterprise environments. But with great power comes great responsibility. Developers must be vigilant, as overlooking security practices can expose their applications to vulnerabilities. In this blog post, we will discuss five common mistakes that can make Java code vulnerable and provide actionable insights to enhance security.
1. Not Using Prepared Statements for Database Queries
One of the most prevalent vulnerabilities in web applications is SQL Injection. This happens when an attacker can inject malicious SQL code into a system’s database query. A common mistake that leads to this vulnerability is using concatenated strings to build SQL queries.
Bad Example
String userInput = request.getParameter("userInput");
String query = "SELECT * FROM Users WHERE username = '" + userInput + "'";
In this example, if userInput
contains admin' OR '1' = '1
, the query becomes valid SQL that grants unauthorized access.
Why Prepared Statements?
To protect against SQL Injection, utilize Prepared Statements. They separate SQL code from the data, effectively eliminating the injection risk.
Good Example
String query = "SELECT * FROM Users WHERE username = ?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, userInput);
By using PreparedStatement
, the database treats the userInput
as data and not SQL code, safeguarding your application’s integrity.
For further reading about prepared statements and security in SQL, check out Oracle's official documentation.
2. Ignoring Exception Handling
Another common mistake is neglecting proper exception handling. Catching exceptions allows developers to understand what went wrong and can prevent the application from crashing unexpectedly.
Bad Example
try {
// Critical operations
} catch (Exception e) {
// Do nothing
}
Ignoring exceptions can lead to unhandled situations that hackers may exploit.
Why Handle Exceptions?
A better approach is to catch specific exceptions and provide a meaningful error message. Logging errors can also give you vital insights for debugging and monitoring.
Good Example
try {
// Critical operations
} catch (SQLException e) {
logger.error("Database error occurred: " + e.getMessage());
// Handle gracefully
} catch (IOException e) {
logger.error("IO error: " + e.getMessage());
// Handle gracefully
}
By logging and handling exceptions appropriately, you can improve your application's resilience.
For guidance on best practices in exception handling, you might want to explore Java Best Practices.
3. Hardcoding Sensitive Information
Hardcoding sensitive information such as database credentials, API keys, or encryption keys in source code is a risky mistake. If your source code becomes public, anyone can access these sensitive parts of your application.
Bad Example
public class DatabaseConfig {
public static final String USERNAME = "admin";
public static final String PASSWORD = "password123";
}
Why Avoid Hardcoding?
Exposing credentials can lead to data breaches and loss of sensitive information.
Better Practices
Instead of hardcoding, consider using configuration files or environment variables. Here's a quick example using environment variables to manage configurations.
Good Example
public class DatabaseConfig {
public static final String USERNAME = System.getenv("DB_USERNAME");
public static final String PASSWORD = System.getenv("DB_PASSWORD");
}
By using environment variables, you can keep sensitive information out of your codebase and minimize the risk of exposure.
4. Lack of Input Validation
Input validation is essential for ensuring that your application behaves correctly. Failing to validate user input can expose your application to various attacks.
Bad Example
String userInput = request.getParameter("age");
int age = Integer.parseInt(userInput); // No validation
If a user inputs a string like "twenty" or "3; DROP TABLE Users", the program will throw an exception or worse, it can lead to SQL injection.
Why Validate Inputs?
Validating inputs can prevent a range of vulnerabilities including XSS (Cross-Site Scripting) and buffer overflow attacks.
Good Example
String userInput = request.getParameter("age");
try {
int age = Integer.parseInt(userInput);
if(age < 0 || age > 120) {
throw new IllegalArgumentException("Invalid age input");
}
} catch (NumberFormatException e) {
// Handle invalid input gracefully
}
By checking that the input falls within a reasonable range, you maintain application integrity and security.
5. Not Using Security Libraries
Many developers reinvent the wheel by developing their own security solutions rather than leveraging existing, robust libraries. This practice can lead to vulnerabilities due to improper implementation of security measures.
Bad Example
public class PasswordUtils {
public static String hashPassword(String password) {
// Custom hashing implementation (risky)
return encodeToSomeHash(password);
}
}
Why Use Security Libraries?
Custom implementations are often flawed. Instead, use tried-and-true libraries like Bouncy Castle or Java Cryptography Architecture (JCA). These libraries are continuously tested and updated by the community.
Good Example
import java.security.MessageDigest;
public class PasswordUtils {
public static String hashPassword(String password) throws Exception {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hashedBytes = md.digest(password.getBytes("UTF-8"));
// Convert byte array to hex format
return bytesToHex(hashedBytes);
}
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
In this example, we utilize JCA for hashing passwords which is a more secure approach than creating a custom algorithm.
My Closing Thoughts on the Matter
Preventing vulnerabilities in Java applications is crucial for protecting your code and your users. By avoiding these common mistakes—using prepared statements, implementing proper exception handling, managing sensitive information correctly, validating inputs, and leveraging security libraries—you can significantly enhance the security of your Java code.
Take the time to review your code for these vulnerabilities and apply the solutions discussed here. A proactive approach will save you from potential headaches down the road.
Remember, security is not an afterthought—it's a key component of software development.
For more resources on secure coding practices in Java, you can check out the OWASP Java Encoder Project or the Java Security Documentation.