Mastering Java: Equals, HashCode & ToString in Guavas
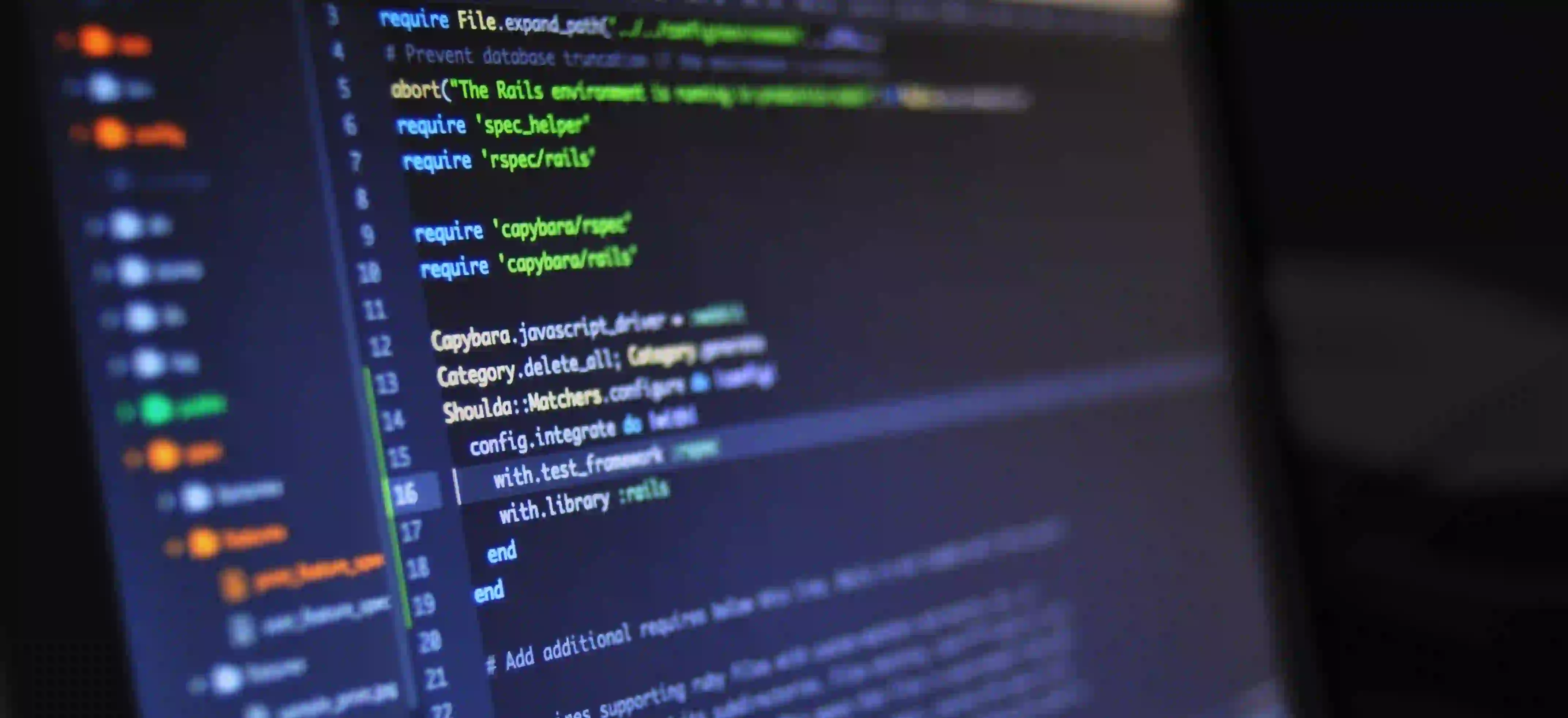
Mastering Java: Equals, HashCode & ToString in Guavas
When working with Java, especially in the context of collections, the methods equals()
, hashCode()
, and toString()
play a pivotal role in how objects are compared, stored, and represented. These methods are not only fundamental in Java but also crucial for maximizing the use of libraries such as Guava. In this blog post, we will explore these methods, their importance, and how to leverage Guava's utilities to simplify their implementation.
Understanding Equals and HashCode
Let's break down the concepts of equals()
and hashCode()
.
The Equals Method
In Java, the equals()
method is used to compare two objects for equality. The default implementation provided by Object
checks for reference equality, which means that it only returns true if both references point to the same object. However, in many cases, we need a more semantic equality, for which we need to override this method.
Here is a basic example of how to implement equals()
:
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyClass myClass = (MyClass) obj;
return Objects.equals(fieldOne, myClass.fieldOne) &&
Objects.equals(fieldTwo, myClass.fieldTwo);
}
Why This Matters
- Semantic Equality: In the above implementation, we compare the fields of our class (
fieldOne
andfieldTwo
) instead of the object references. This ensures that two distinct instances with the same values can be considered equal.
The HashCode Method
The hashCode()
method is crucial for hash-based collections like HashMap
and HashSet
. Whenever you override equals()
, you must also override hashCode()
. The general contract is that equal objects must have the same hash code.
Here's a simple hashCode()
implementation:
@Override
public int hashCode() {
return Objects.hash(fieldOne, fieldTwo);
}
Why This Matters
- Hash-based Collection Integrity: A consistent hash code allows for the effective storage and retrieval of objects in collections. If two objects are equal according to
equals()
, they must return the same hash code.
Using Guava for Equals and HashCode
The Google Guava library simplifies the implementation of equals()
and hashCode()
. For instance, you can use Objects.equal()
and Objects.hashCode()
methods from Guava.
Here’s how you can do it:
import com.google.common.base.Objects;
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyClass myClass = (MyClass) obj;
return Objects.equal(fieldOne, myClass.fieldOne) &&
Objects.equal(fieldTwo, myClass.fieldTwo);
}
@Override
public int hashCode() {
return Objects.hashCode(fieldOne, fieldTwo);
}
Why Guava?
-
Readability: Guava's methods enhance the readability of your code, making it clear what you are trying to achieve with equality checks and hash codes.
-
Reliable Implementations: With Guava, a well-tested codebase backs up the equality and hash code functionalities, minimizing bugs in edge cases.
For further understanding of Guava's capabilities, you can visit Guava's official documentation.
The ToString Method
The toString()
method also holds substantial importance. This method is used to represent your object as a String, which is particularly useful for debugging and logging.
Basic Implementation
Here’s a simple toString()
implementation:
@Override
public String toString() {
return "MyClass{" +
"fieldOne='" + fieldOne + '\'' +
", fieldTwo='" + fieldTwo + '\'' +
'}';
}
Why This Matters
- Debugging: A well-implemented
toString()
method makes it easier to log and troubleshoot your application.
Simplifying with Guava
Just like equals()
and hashCode()
, Guava provides a fluent way to implement toString()
.
import com.google.common.base.MoreObjects;
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("fieldOne", fieldOne)
.add("fieldTwo", fieldTwo)
.toString();
}
Advantages of Using Guava for toString
-
Fluency: The
MoreObjects.toStringHelper
method allows for a clear and concise string representation of the object. -
Maintainability: Adding new fields over time becomes easier; just add another
.add()
call, and your string representation is automatically updated.
Best Practices for Equals, HashCode, and ToString
-
Always Override Together: When you override
equals()
, always overridehashCode()
andtoString()
. -
Consistency Across Implementations: Make sure that your
equals()
andhashCode()
methods reflect the same set of fields. -
Use the Java Utility Classes: Leverage
java.util.Objects
and Guava’s utilities to simplify your implementation. -
Unit Testing: Don’t forget to unit test your implementations to verify that
equals()
,hashCode()
, andtoString()
behave as expected.
The Closing Argument
Mastering the equals()
, hashCode()
, and toString()
methods in Java is integral to creating robust, reliable, and maintainable code. With the help of libraries like Guava, implementing these methods becomes not only easier but also more reliable and easier to read. By following best practices and leveraging the tools available, you can ensure that your Java classes perform optimally in diverse contexts.
For more detailed exploration, consider checking out the Java documentation and the Guava library for advanced features.
Happy coding!