Mastering JDK 8 Streams: Overcoming Grouping Challenges
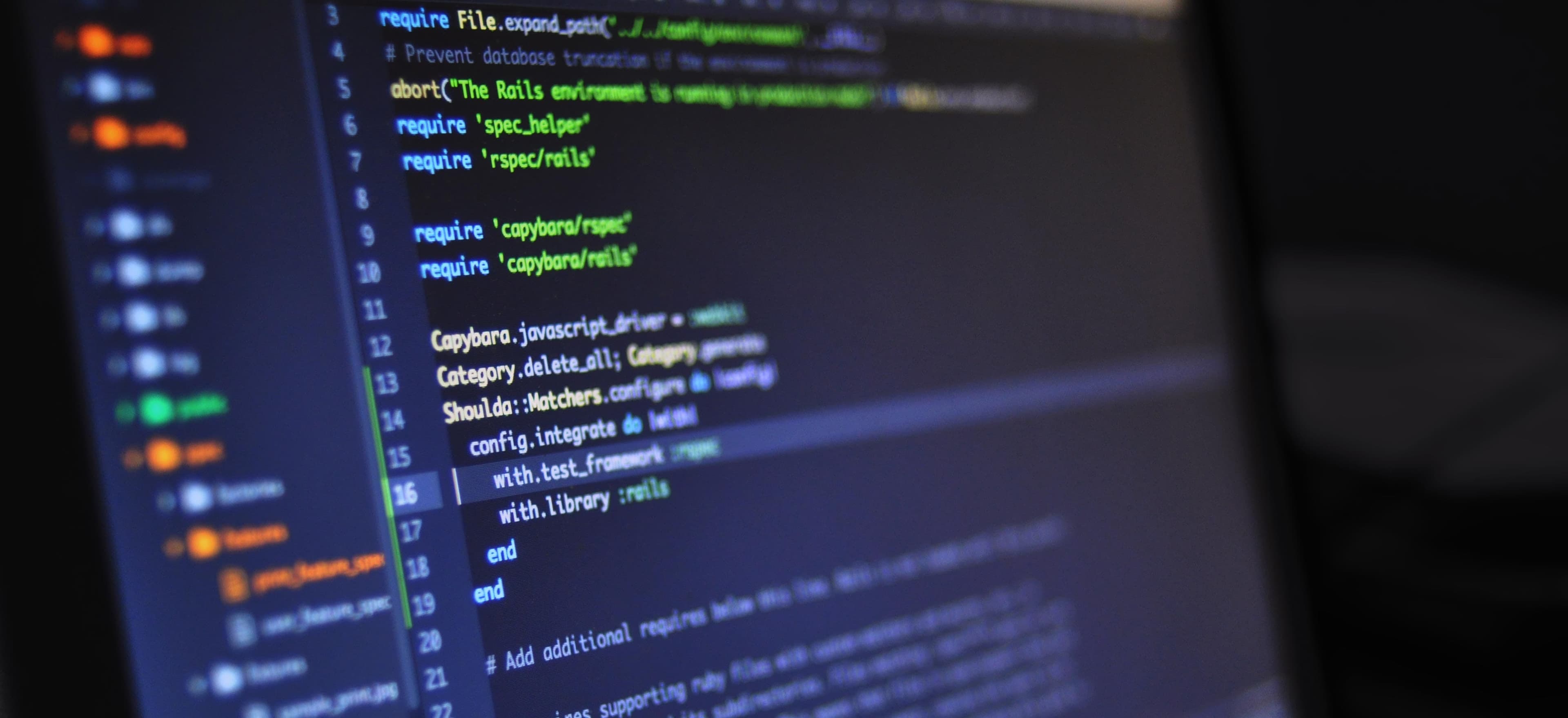
- Published on
Mastering JDK 8 Streams: Overcoming Grouping Challenges
Java 8 has transformed how we work with collections through a powerful feature called Streams. Initially introduced as a way to process sequences of elements in a functional style, Streams can greatly simplify complex data manipulations. One common challenge many developers encounter is grouping elements with Streams. In this blog, we'll explore how to master grouping in Streams, tackle associated challenges, and streamline your data-processing tasks.
Understanding the Stream API
Before delving into grouping, it's essential to understand what the Stream API is. The Stream API allows you to process data in a declarative way, enabling developers to write more concise and readable code. Here's a quick overview of how to create a Stream:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
Stream<String> stream = names.stream();
The Power of Grouping
Grouping is a fundamental operation when working with collections of objects. For instance, you may want to group a list of employees by their departments. This is where the Collectors.groupingBy
method comes into play.
Example: Grouping Employees by Department
Let’s consider we have a simple Employee
class as follows:
public class Employee {
private String name;
private String department;
public Employee(String name, String department) {
this.name = name;
this.department = department;
}
public String getName() {
return name;
}
public String getDepartment() {
return department;
}
}
Given this class, suppose we have a list of employees:
List<Employee> employees = Arrays.asList(
new Employee("Alice", "Finance"),
new Employee("Bob", "HR"),
new Employee("Charlie", "Finance"),
new Employee("David", "IT")
);
To group these employees by department, we can leverage the Stream API like so:
Map<String, List<Employee>> groupedByDepartment = employees.stream()
.collect(Collectors.groupingBy(Employee::getDepartment));
Breaking Down the Code
- Stream Creation: We start by converting the list of employees into a Stream.
- Collect Method: The
collect
method is a terminal operation that transforms the Stream into a different form, in this case, aMap
. - Grouping: Using
Collectors.groupingBy
, we specify that we want to group employees based on the result ofEmployee::getDepartment
.
This results in a map where each key is a department, and the value is a list of corresponding employees.
Output Example
To visualize the result, you might want to print it out:
groupedByDepartment.forEach((department, empList) -> {
System.out.println("Department: " + department);
empList.forEach(emp -> System.out.println(" - " + emp.getName()));
});
This will output:
Department: Finance
- Alice
- Charlie
Department: HR
- Bob
Department: IT
- David
Challenges in Grouping
Though straightforward, grouping isn't always easy. Let's cover a few common challenges and their solutions.
1. Grouping by Multiple Criteria
There are situations where you may need to group by multiple fields. For example, let's say we also want to group employees by their names' first letter within the departments.
This can be accomplished by creating a composite key using a custom class or Map
. Here's an example using a simple string concatenation as a key:
Map<String, List<Employee>> groupedByCompositeKey = employees.stream()
.collect(Collectors.groupingBy(emp -> emp.getDepartment() + "-" + emp.getName().charAt(0)));
2. Handling Null Values
Often, your dataset may contain null values. These could lead to issues during grouping. To mitigate this, you can filter the nulls out before processing:
Map<String, List<Employee>> groupedFiltered = employees.stream()
.filter(Objects::nonNull)
.collect(Collectors.groupingBy(Employee::getDepartment));
3. Grouping and Counting
Sometimes, you may not need the actual items in each group, but rather a count of items. You can do this easily with Collectors.counting()
:
Map<String, Long> departmentCount = employees.stream()
.collect(Collectors.groupingBy(Employee::getDepartment, Collectors.counting()));
4. Grouping with Custom Result Types
You don't have to stick to basic collection types like List
or Long
for your groups. You can create your custom result type:
public class DepartmentSummary {
private String department;
private long employeeCount;
public DepartmentSummary(String department, long employeeCount) {
this.department = department;
this.employeeCount = employeeCount;
}
// Getters and toString method...
}
Now, you can group and map directly into this custom type:
List<DepartmentSummary> summaries = employees.stream()
.collect(Collectors.groupingBy(Employee::getDepartment, Collectors.counting()))
.entrySet()
.stream()
.map(entry -> new DepartmentSummary(entry.getKey(), entry.getValue()))
.collect(Collectors.toList());
Best Practices in Grouping
To maximize the effectiveness of grouping using Streams, consider these tips:
-
Always Validate Inputs: Check for null or unexpected values to prevent runtime exceptions.
-
Use Immutability: Favor immutable collections to avoid side effects and maintain thread safety.
-
Leverage Parallel Streams: For large datasets, consider using parallel streams for better performance.
List<DepartmentSummary> summaries = employees.parallelStream() .collect(Collectors.groupingBy(Employee::getDepartment, Collectors.counting())) .entrySet() .stream() .map(entry -> new DepartmentSummary(entry.getKey(), entry.getValue())) .collect(Collectors.toList());
-
Profiling: Test the boundaries of your code with varying dataset sizes to identify performance bottlenecks.
In Conclusion, Here is What Matters
Mastering grouping with JDK 8 Streams is a powerful skill that enhances your ability to manipulate and summarize data. As we've explored, challenges can arise, but with the right approaches, these obstacles become opportunities for cleaner, more efficient code. By harnessing the full capabilities of the Streams API, you can streamline your data processing tasks, making your Java applications more robust and maintainable.
For further reading, consider checking the Java 8 Stream documentation for more insights into how to effectively utilize Streams in your projects.
Now it's your turn—experiment with grouping in your applications and share your experiences in the comments below! Happy coding!