Dealing with Parsing Errors in Java CLI Tools
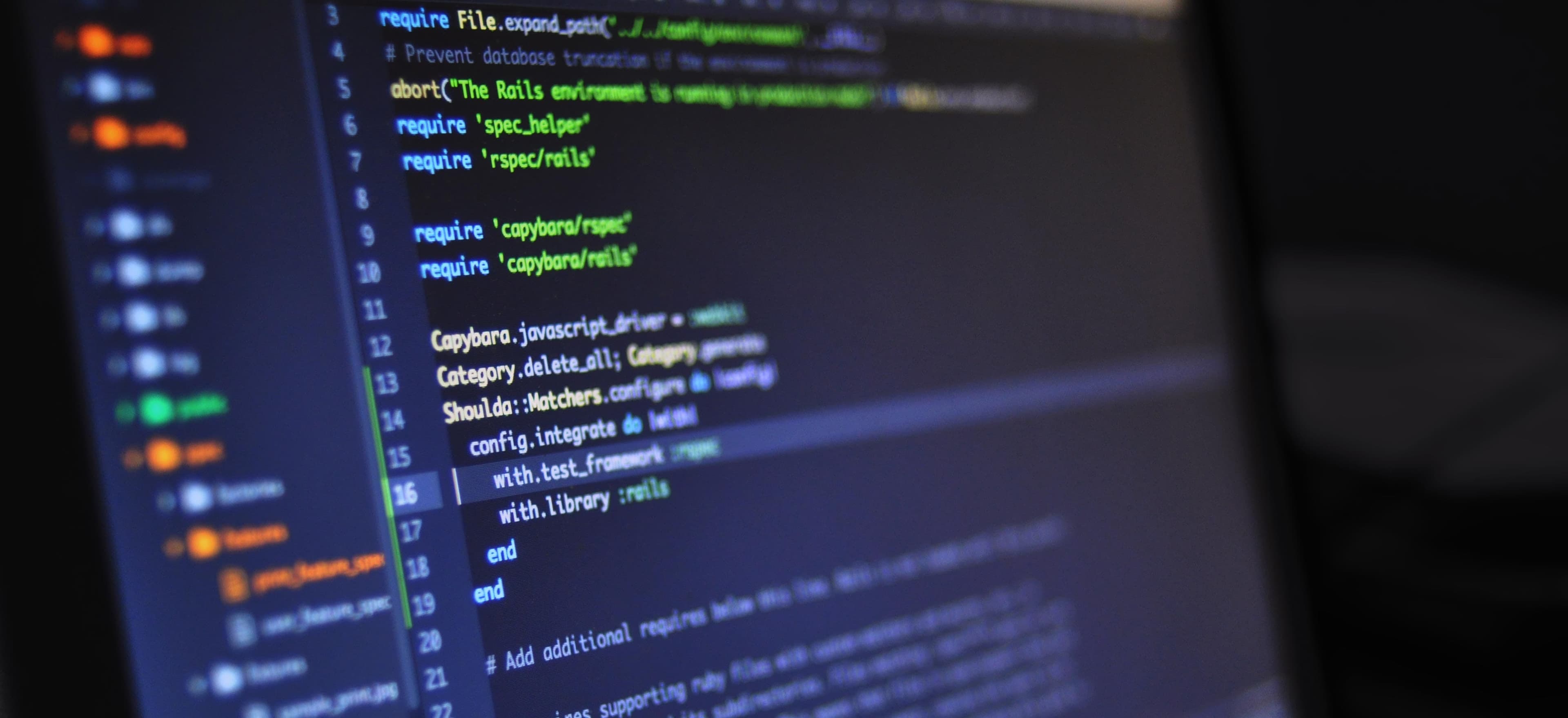
- Published on
When developing a Command Line Interface (CLI) tool in Java, ensuring that the tool can effectively parse user input is crucial for its functionality. Parsing errors can lead to unexpected behavior and make the tool difficult to use. In this blog post, we'll delve into common parsing errors in Java CLI tools and discuss strategies for handling them effectively.
Understanding Parsing Errors
Parsing errors occur when the input provided by the user does not conform to the expected format or data type. In the context of a CLI tool, parsing errors can arise when command-line arguments are incorrectly provided or when the user input cannot be interpreted as expected.
Using Apache Commons CLI
One popular library for creating command line interfaces in Java is Apache Commons CLI. It provides a powerful framework for parsing command line options and arguments. Let's consider an example where we want to create a simple CLI tool that accepts an integer value as input.
Options options = new Options();
options.addOption(Option.builder("num")
.longOpt("number")
.desc("Specify a number")
.hasArg()
.argName("number")
.required()
.build());
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
int number = Integer.parseInt(cmd.getOptionValue("num"));
// Make use of the parsed integer value
} catch (ParseException | NumberFormatException e) {
System.err.println("Parsing error: " + e.getMessage());
// Handle parsing errors
}
In this example, we define a CLI option 'num' that accepts a number as its value. The DefaultParser
attempts to parse the command line arguments, and we handle any parsing errors using the ParseException
and NumberFormatException
classes.
Handling Parsing Errors
When it comes to handling parsing errors in a Java CLI tool, it's essential to provide meaningful feedback to the user about the nature of the error. This can be achieved by displaying clear error messages and offering guidance on how to correct the issue.
try {
// Parse command line arguments
} catch (ParseException e) {
System.err.println("Parsing error: " + e.getMessage());
// Display usage help or specific error details
}
Additionally, considering the use of custom exceptions or error codes to categorize different types of parsing errors can offer more granular control over error handling.
Validating User Input
In some cases, it's beneficial to preprocess and validate user input before attempting to parse it. This can help catch potential issues early on and provide a better user experience.
String userInput = cmd.getOptionValue("input");
if (!isValidInput(userInput)) {
System.err.println("Invalid input: " + userInput);
// Handle invalid input
}
// ...
private static boolean isValidInput(String input) {
// Validate the input using custom logic
}
Providing Usage Help
Another fundamental aspect of handling parsing errors is to provide comprehensive usage help to the user. This should include information on how to use the CLI tool, the expected format of the input, and examples of valid usage.
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("mycli", options);
By utilizing the HelpFormatter
class from Apache Commons CLI, you can generate and display a formatted help message that outlines the usage of the CLI tool and its available options.
Testing for Robustness
To ensure the robustness of the parsing and error handling mechanisms in a Java CLI tool, writing comprehensive unit tests is paramount. By crafting test cases that cover various input scenarios, including valid, invalid, and edge cases, you can verify that the parsing and error handling functionality behaves as intended.
Bringing It All Together
In the realm of Java CLI tools, dealing with parsing errors is an integral part of creating a user-friendly and robust application. By leveraging libraries such as Apache Commons CLI and implementing best practices for handling parsing errors, developers can enhance the quality and usability of their CLI tools. Effective error handling, input validation, and providing clear usage guidance are pivotal elements in delivering a seamless user experience.
When parsing errors are effectively managed, users can interact with the CLI tool confidently, knowing that any input issues will be promptly identified and communicated. With thorough testing and attention to detail, Java CLI tools can offer a reliable and intuitive interface for users to engage with the underlying functionality.