Handling Large Fibonacci Numbers: The BigInteger Solution
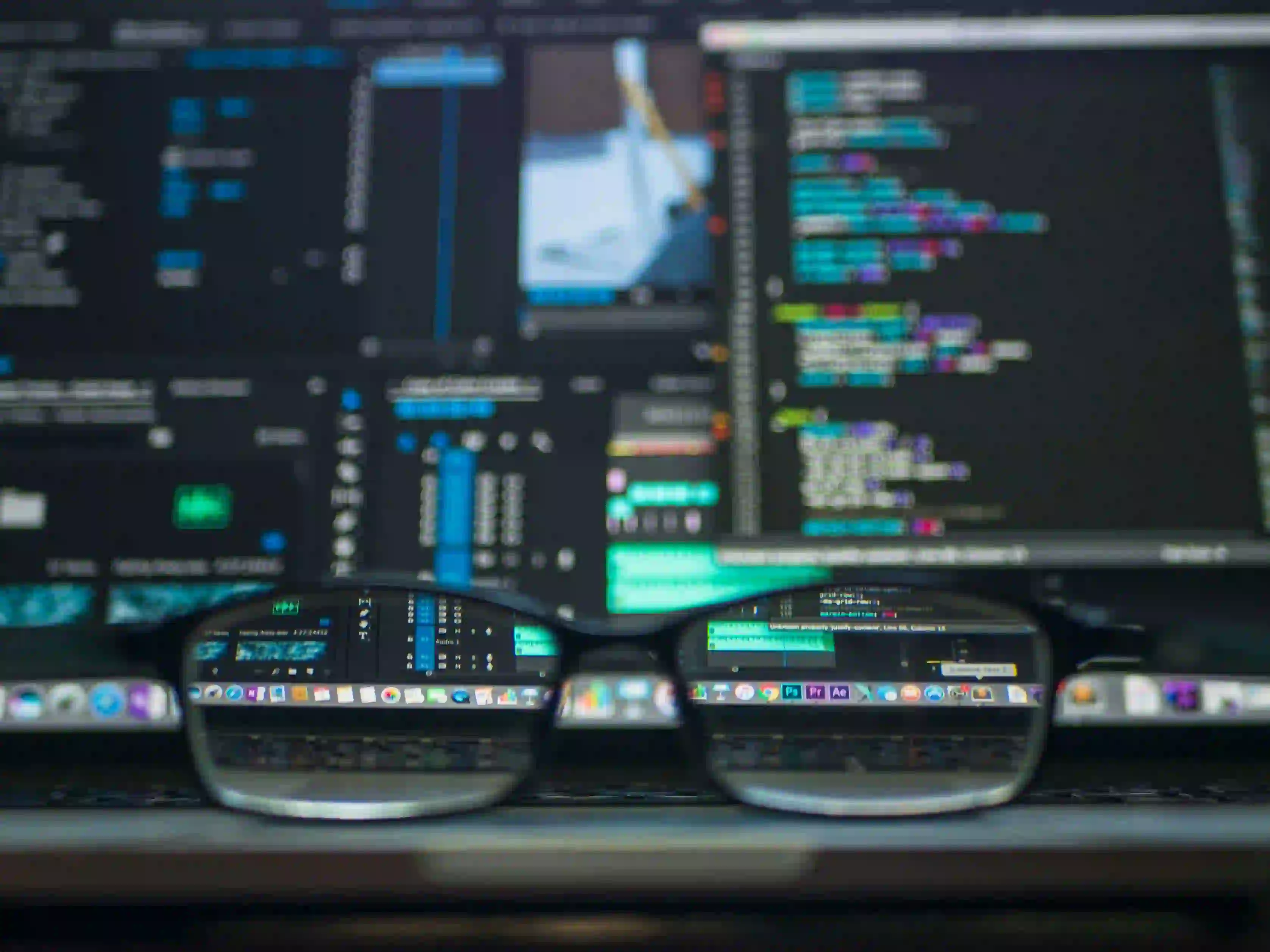
Handling Large Fibonacci Numbers: The BigInteger Solution
When programming, calculating Fibonacci numbers is a common exercise to understand algorithm efficiency and recursion. However, the challenge arises when dealing with large Fibonacci numbers, especially when they exceed the storage capacity of standard data types. In Java, this is where the BigInteger
class comes into play.
In this blog post, we will explore how to use BigInteger
to calculate large Fibonacci numbers effectively and efficiently.
Understanding Fibonacci Numbers
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. It starts as follows:
- F(0) = 0
- F(1) = 1
- F(2) = F(0) + F(1) = 0 + 1 = 1
- F(3) = F(1) + F(2) = 1 + 1 = 2
- F(4) = F(2) + F(3) = 1 + 2 = 3
- F(5) = F(3) + F(4) = 2 + 3 = 5
- And so on...
The Fibonacci sequence grows exponentially, which leads to quickly increasing values for large indexes. For instance, F(100) is already 354224848179261915075. Standard data types in Java, such as int
or long
, are inadequate for such large numbers.
Why Use BigInteger?
BigInteger
is part of the java.math
package and allows you to work with integers of arbitrary size. The main advantages of using BigInteger
are:
- Arbitrary Precision: You are limited only by the memory of your system.
- Rich API: It provides various methods for mathematical operations.
- Easy Integration: You can seamlessly incorporate it into your existing Java applications.
Installing BigInteger
Before we proceed, ensure that your Java environment is set up. If you are using any IDE like IntelliJ IDEA or Eclipse, they automatically include the java.math
package, and no further installation is needed.
Implementing Fibonacci with BigInteger
Now, let's dive into the code. Below are multiple approaches shown to calculate Fibonacci numbers, demonstrating both recursion and iteration.
Iterative Approach
The iterative method is generally more efficient than the recursive approach as it runs in O(n) time and uses constant space O(1). Here’s how you can implement it using BigInteger
.
import java.math.BigInteger;
public class Fibonacci {
public static BigInteger fibonacci(int n) {
if (n < 0) {
throw new IllegalArgumentException("Index cannot be negative.");
}
if (n == 0) return BigInteger.ZERO;
if (n == 1) return BigInteger.ONE;
BigInteger a = BigInteger.ZERO;
BigInteger b = BigInteger.ONE;
BigInteger fib = BigInteger.ZERO;
for (int i = 2; i <= n; i++) {
fib = a.add(b); // Calculate the next Fibonacci number
a = b; // Move to the next position
b = fib; // Update b as the latest Fibonacci number
}
return fib;
}
public static void main(String[] args) {
int index = 100;
System.out.println("Fibonacci number at index " + index + " is: " + fibonacci(index));
}
}
Code Commentary
- Parameters: The method
fibonacci(int n)
takes an integer index and returns the nth Fibonacci number as aBigInteger
. - Error Handling: We handle negative indices by throwing an
IllegalArgumentException
. - Logic: Using a for loop, we iteratively calculate Fibonacci numbers. Each iteration computes the next Fibonacci number by adding the previous two.
Recursive Approach
While recursion is a popular method to define Fibonacci due to its simplicity, it is not efficient for large values due to exponential time complexity. Nonetheless, here is how you can implement it with memoization to optimize performance:
import java.math.BigInteger;
import java.util.HashMap;
public class FibonacciMemo {
private static HashMap<Integer, BigInteger> memo = new HashMap<>();
public static BigInteger fibonacci(int n) {
if (n < 0) {
throw new IllegalArgumentException("Index cannot be negative.");
}
if (n == 0) return BigInteger.ZERO;
if (n == 1) return BigInteger.ONE;
// Check if result already computed
if (memo.containsKey(n)) {
return memo.get(n);
}
// Recur and store the computed Fibonacci number
BigInteger result = fibonacci(n - 1).add(fibonacci(n - 2));
memo.put(n, result); // Memoization step
return result;
}
public static void main(String[] args) {
int index = 100;
System.out.println("Fibonacci number at index " + index + " is: " + fibonacci(index));
}
}
Code Commentary
- Memoization: We use a
HashMap
to store previously computed Fibonacci values, significantly reducing repeated calculations. - Recursion: The recursion remains simple; base cases are checked first before proceeding to recursive calls.
Performance Considerations
When working with large Fibonacci values, performance becomes critical. The iterative method is often preferred in production code due to its efficiency and lower memory overhead.
Comparing Both Methods
-
Iterative:
- Time Complexity: O(n)
- Space Complexity: O(1)
-
Recursive with Memoization:
- Time Complexity: O(n)
- Space Complexity: O(n) due to the call stack and memoization storage.
My Closing Thoughts on the Matter
Calculating large Fibonacci numbers in Java requires special consideration for data types, particularly when values exceed standard limits. The BigInteger
class allows for easy manipulation of very large integers that would otherwise cause overflow issues.
In this post, we have explored two different approaches for calculating Fibonacci numbers using BigInteger
. The iterative method is generally more efficient in terms of space and time, while the recursive method showcases elegance and the power of memoization.
If you would like to learn more about BigInteger
, check out the official Java documentation.
Happy coding!