Why Sticking to JPA and JDBC Can Hurt Your Project
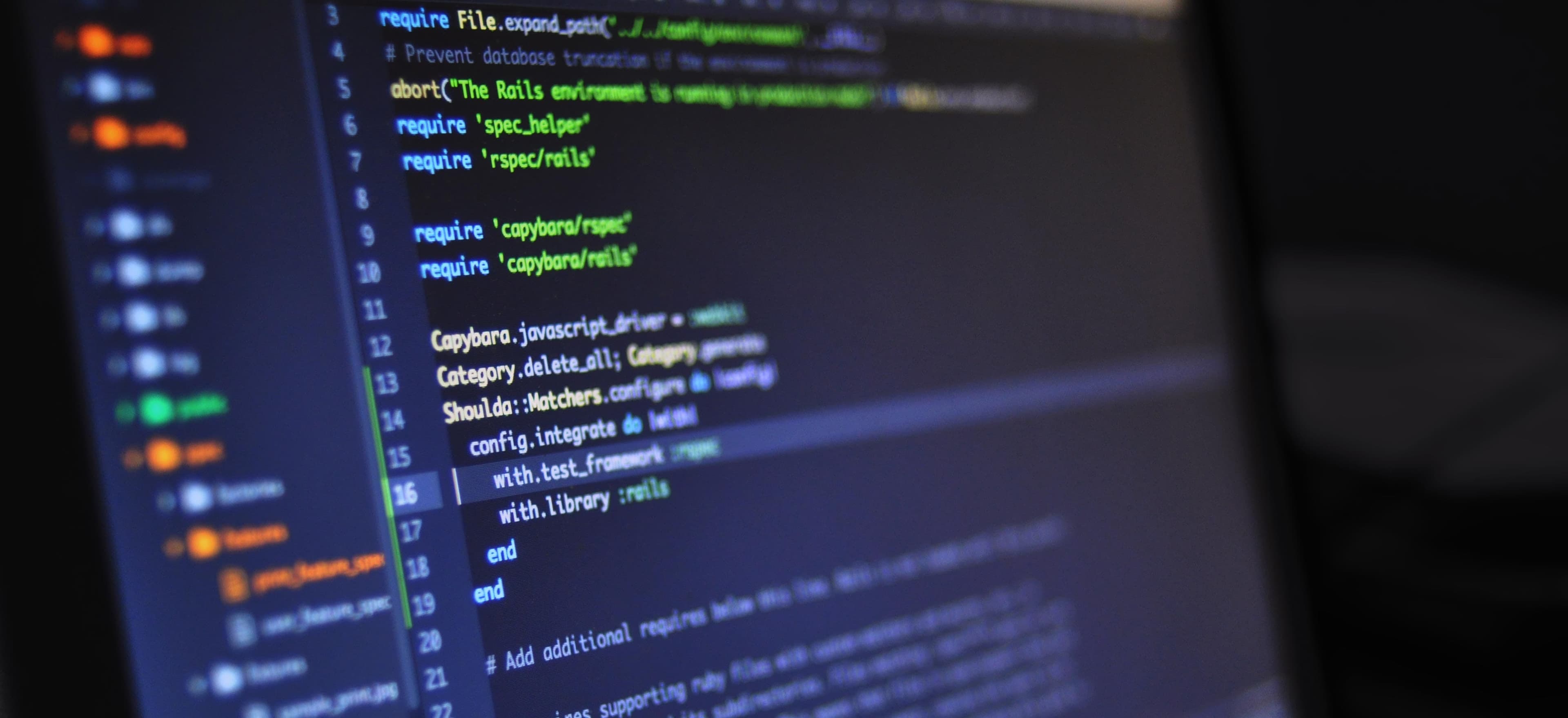
- Published on
Why Sticking to JPA and JDBC Can Hurt Your Project
Java Persistence API (JPA) and Java Database Connectivity (JDBC) have been integral parts of Java development for years. They provide robust frameworks for interacting with databases. However, while many developers stick to these technologies by default, it’s essential to evaluate whether they are the best fit for every project. In this blog post, we will explore the disadvantages of relying solely on JPA and JDBC, and discuss alternative approaches that could result in a more efficient, maintainable, and scalable project.
Understanding JPA and JDBC
What is JDBC?
JDBC is a Java-based API that allows Java applications to interact with a variety of databases through SQL. It provides the basic functionality to connect to databases, execute SQL statements, and retrieve results.
Example of JDBC connection:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "username";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password)) {
String sql = "SELECT * FROM users";
PreparedStatement ps = conn.prepareStatement(sql);
ResultSet rs = ps.executeQuery();
while (rs.next()) {
System.out.println("User: " + rs.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
What is JPA?
JPA is a specification that provides an API for object-relational mapping (ORM). It allows developers to map Java objects to database tables, making it easier to manage data persistence.
Example of JPA entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String name;
// Getters and setters
public Long getId() { return id; }
public void setId(Long id) { this.id = id; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
}
Why Sticking to JPA and JDBC Can Be Detrimental
While JPA and JDBC can be effective, there are several reasons why over-reliance on these technologies can harm a project:
1. Performance Overhead
JPA, by its nature, can introduce a layer of abstraction that may impact performance. The ORM translations, lazy loading strategies, and additional caching mechanisms can lead to performance issues.
- Implication: For high-performance applications requiring micro-optimizations, the overhead from JPA may not be acceptable.
Tip: Always assess whether your application needs the complete ORM capabilities. In cases where speed is crucial, you might find a lighter solution using simple JDBC.
2. Complexity of Use Cases
JPA can handle various database operations but can become overly complex with intricate relationships or advanced querying. SQL's simplicity and direct control can be more effective when performance matters.
Example: Complex Queries in JPA
public List<User> findUserByCriteria(String name, boolean active) {
return entityManager.createQuery(
"SELECT u FROM User u WHERE u.name = :name AND u.active = :active", User.class)
.setParameter("name", name)
.setParameter("active", active)
.getResultList();
}
- Why: This code snippet shows how JPQL can abstract SQL, but adding multiple filters may complicate things. Maintaining and debugging these queries often becomes more cumbersome than using plain SQL.
3. Lack of Control
Both JPA and JDBC manage connection pools, transactions, and mappings. This added layer may reduce visibility over what actually happens in your database. When errors occur, pinpointing issues requires understanding both the API and the database system.
- Implication: When performance degradation happens, developers spend time diagnosing the ORM rather than the underlying database.
4. Limited Flexibility
Sticking to a single technology can lead to limitations when it comes to deploying different databases or working with multiple data sources.
-
Example: Integrating a NoSQL database, like MongoDB or Cassandra, into a project using JPA, can pose significant challenges. Developers might need to refactor their entire data layer.
-
Solution: Embrace polyglot persistence. Understand your data needs and pick the right tool for each job.
5. Learning Overhead for Team Members
JPA has a steeper learning curve compared to traditional JDBC. This can be challenging for new team members who are not familiar with ORM concepts.
- Implication: The more complex the underlying framework, the longer it may take new developers to become productive. This can slow down your project's growth.
6. Version Control and Migration Problems
Maintaining JPA with evolving project requirements can lead to versioning headaches. Changing an entity structure often fractures the underlying database schema.
- Why It’s a Problem: The direct correlation between your entities and database schema means any changes require simultaneous updates in both places, leading to an increased risk of issues.
Alternatives to Consider
While JPA and JDBC are staples in the Java ecosystem, developers are adopting various methodologies and frameworks that can deliver better performance, flexibility, and maintainability:
1. Spring Data JDBC
Spring Data JDBC provides an alternative way of working with databases that adheres to a simpler, and more straightforward mapping model. It doesn’t impose the complex relationship management of JPA ORM.
Example of Repository Pattern:
import org.springframework.data.jdbc.repository.Query;
import org.springframework.data.repository.CrudRepository;
public interface UserRepository extends CrudRepository<User, Long> {
@Query("SELECT * FROM users WHERE name = :name")
List<User> findByName(String name);
}
- Why Use It: Offers less complexity and better performance while retaining a familiar Spring environment.
2. JOOQ
JOOQ (Java Object Oriented Querying) is another powerful tool that allows you to express SQL in a type-safe manner. It maps your database tables to Java classes, enabling intuitive SQL-like queries.
Example JOOQ Query:
DSLContext create = DSL.using(connection, SQLDialect.MYSQL);
Result<Record> result = create.select()
.from("users")
.where(field("name").eq("John"))
.fetch();
- Why Use JOOQ? It allows for writing SQL statements directly, offering the flexibility and control that JPA abstracts away.
3. Native Queries
In situations where JPA is still needed due to complex domain models, consider using native queries for optimization. This approach allows for SQL to be embedded directly within your JPA code.
Example of Native Query in JPA:
public List<User> findAllUsers() {
return entityManager.createNativeQuery("SELECT * FROM users", User.class)
.getResultList();
}
- Why Use This: It provides maximum flexibility when specific performance requirements and optimizations are needed that JPA integrations cannot achieve.
Final Thoughts
While JPA and JDBC are robust tools for Java developers, relying solely on them can lead to performance problems, complexity, and a lack of flexibility. It’s advisable to explore alternatives like Spring Data JDBC or JOOQ that can better serve specific project needs. Remember, the goal should be to choose the right tool for the job rather than forcing every project into a one-size-fits-all solution.
Embrace flexibility and adaptability in your tech stack, and you'll set your projects up for scalable success. For further insights into efficient data management in Java, consider checking out Spring Framework Documentation and JOOQ User Guide.
By staying informed and adaptable within the evolving landscape of database management, you'll ensure that your projects not only perform efficiently but are also maintainable as they grow.