When to Use Mocking in Software Testing
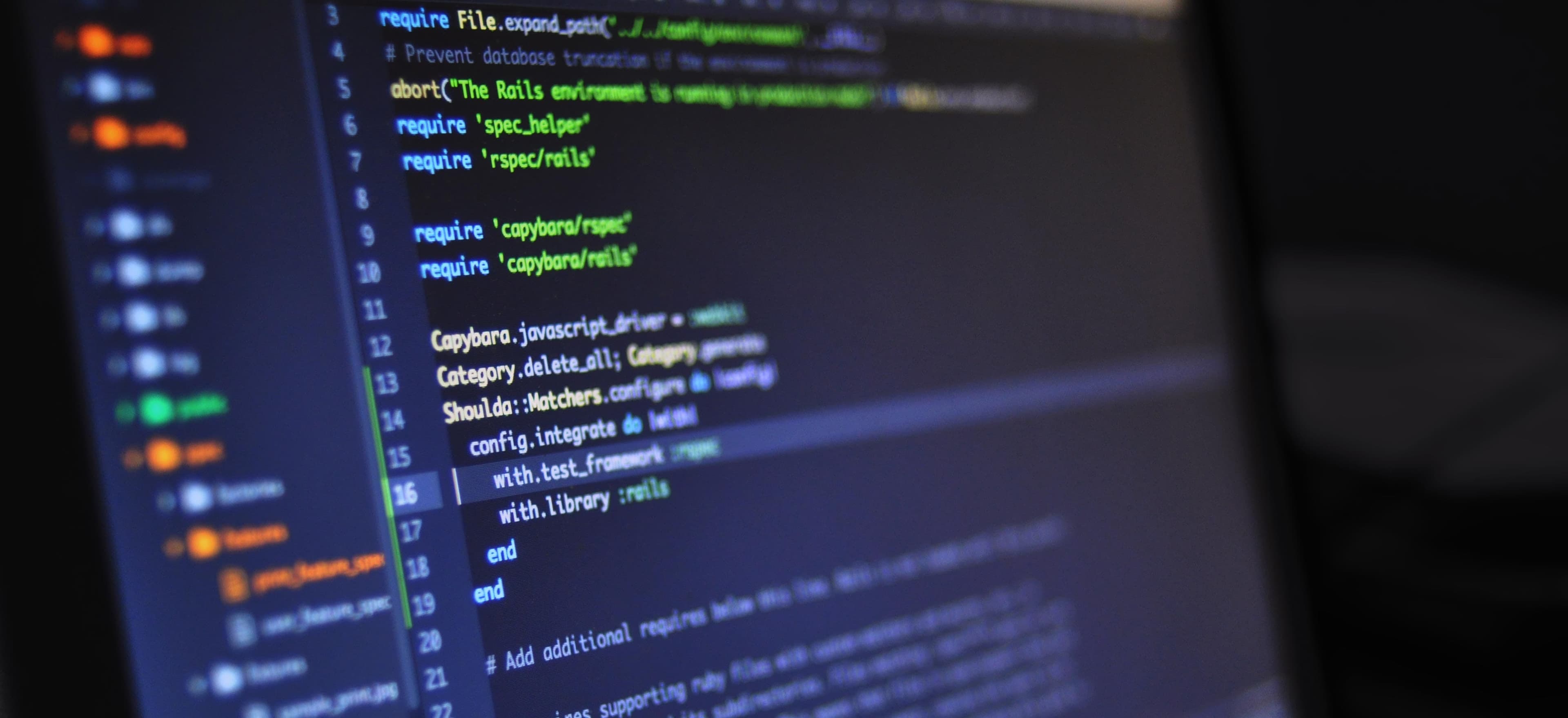
- Published on
Understanding the Importance of Mocking in Java Software Testing
In the world of software development, testing plays a crucial role in ensuring the quality and reliability of a product. Java, as one of the most popular programming languages, offers a variety of testing frameworks and tools to support developers in writing effective tests for their applications. One important concept in Java testing is mocking, which is particularly useful when testing code that depends on external dependencies such as databases, web services, or other components.
What is Mocking?
Mocking is a technique used in testing to create simulated objects that mimic the behavior of real objects. These simulated objects, known as mocks, are designed to imitate the responses and interactions of actual components or dependencies that a piece of code relies on. By using mocks, developers can isolate the code under test and verify its behavior without depending on the actual implementations of external dependencies.
When to Use Mocking?
1. Testing External Dependencies
Mocking is especially valuable when testing code that interacts with external dependencies, such as databases, network services, or third-party libraries. By using mocks to simulate the behavior of these dependencies, tests can focus exclusively on the logic and functionality of the code being tested, without being affected by the availability or state of the actual external resources.
2. Improving Test Performance
In scenarios where real external resources involve time-consuming operations or are not easily accessible in the testing environment, using mocks can significantly improve the performance and efficiency of tests. Mocks provide predictable and fast responses, allowing tests to be executed more rapidly and frequently without relying on the actual external resources.
3. Isolating Code for Unit Testing
When testing individual units of code, it's essential to isolate the code under test from its dependencies. Mocking enables developers to create controlled environments for unit testing, where the focus is solely on the behavior of the unit being tested, rather than on the behavior of its dependencies.
The Mockito Framework for Mocking in Java
Essentials at a Glance to Mockito
Mockito is a popular Java framework that provides support for creating and using mocks in testing. It offers a simple and expressive API for setting up mock objects, defining their behavior, and verifying interactions with them. Let's take a look at some essential features of Mockito and how it can be used effectively for mocking in Java testing.
Setting Up Mocks with Mockito
To demonstrate the basic usage of Mockito for mocking in Java testing, let's consider a simple example where we have a UserService
interface and a UserController
class that depends on this interface.
public interface UserService {
String getUserRole(String username);
}
public class UserController {
private UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
public String getUserRole(String username) {
return userService.getUserRole(username);
}
}
In our test class, we can use Mockito to create a mock UserService
and define its behavior as shown below:
import static org.mockito.Mockito.*;
public class UserControllerTest {
@Test
public void testGetUserRole() {
UserService mockUserService = mock(UserService.class);
when(mockUserService.getUserRole("john")).thenReturn("admin");
UserController userController = new UserController(mockUserService);
String userRole = userController.getUserRole("john");
assertEquals("admin", userRole);
verify(mockUserService).getUserRole("john");
}
}
In this example, we use Mockito's mock
method to create a mock object for the UserService
interface. We then use the when
method to define the behavior of the mock, specifying that when the getUserRole
method is invoked with the argument "john", the mock should return "admin". Finally, we verify that the getUserRole
method of the mock was called exactly once with the argument "john" using Mockito's verify
method.
Why Use Mockito for Mocking?
The example above illustrates how Mockito simplifies the process of creating and using mocks in Java testing. Mockito's fluent and readable syntax, along with its powerful features for defining mock behavior and verifying interactions, makes it a valuable tool for achieving effective mocking in test scenarios.
The Last Word
Mocking plays a critical role in Java software testing, particularly when dealing with external dependencies, improving test performance, and enabling focused unit testing. Mockito, with its user-friendly API and robust capabilities, provides a solid foundation for incorporating mocking into Java test suites. By mastering the art of mocking, developers can enhance the reliability and effectiveness of their tests, ultimately contributing to the delivery of high-quality, dependable software products.
To delve deeper into Mockito and its advanced features, check out the official Mockito documentation. Additionally, for a comprehensive guide on Java testing best practices, explore the resources provided by JUnit. Happy testing!