Optimize Memory Usage: Efficient File Reading in Java
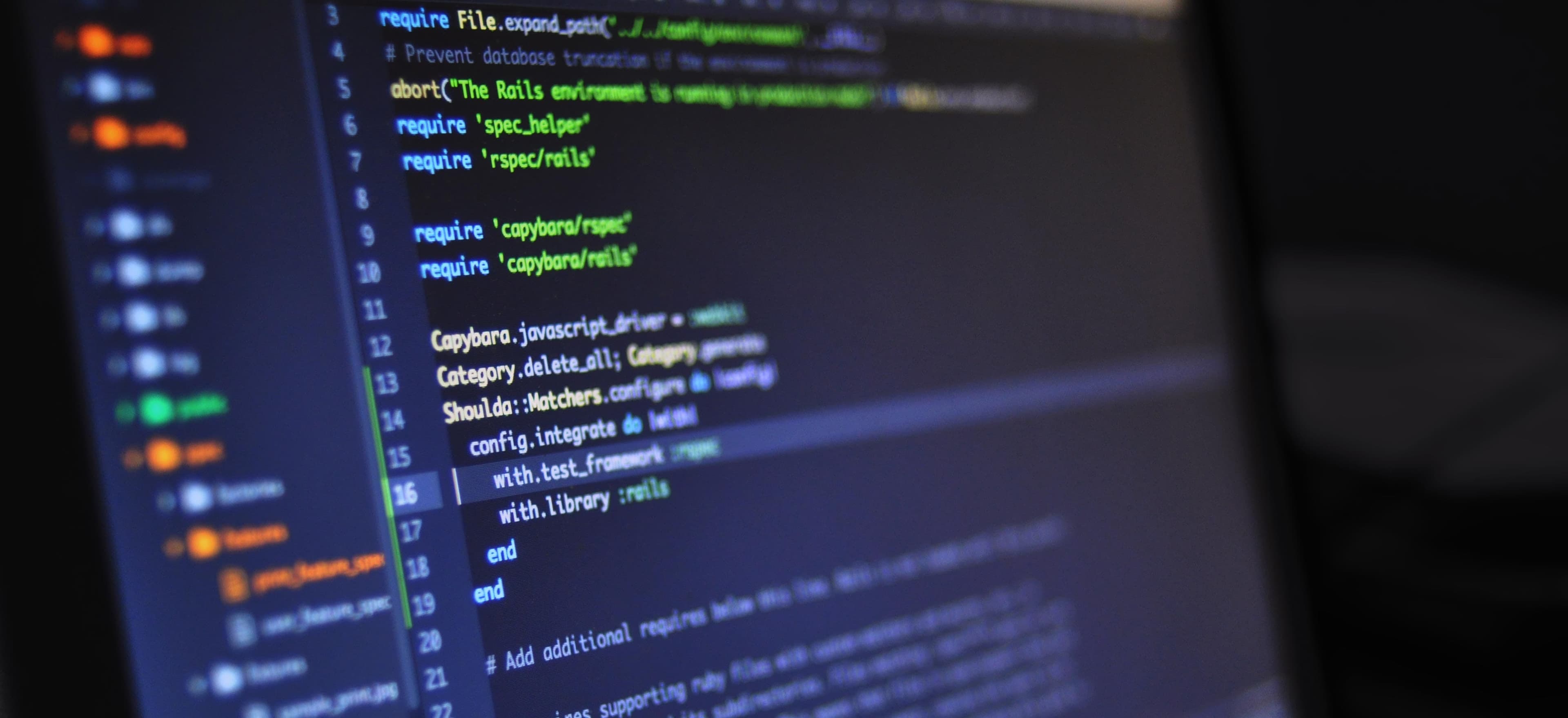
- Published on
Optimize Memory Usage: Efficient File Reading in Java
Efficient file reading in Java is crucial for applications that handle large datasets or files. Many programmers fall into the trap of using straightforward methods that may consume unnecessary memory resources. In this blog post, we’ll explore various techniques to optimize memory usage during file reading in Java, highlight practical code snippets, and discuss when to use each method.
Why Optimize File Reading?
When an application reads files, it often has to handle large amounts of data. If the reading method is inefficient, it can lead to excessive memory consumption, increased garbage collection activity, and ultimately a degraded user experience. Memory management plays a significant role in the performance and scalability of applications, making it essential to adopt best practices.
Understanding Basic File Reading
Before we dive into optimization techniques, let’s look at a basic file-reading example in Java. We’ll use the BufferedReader
class, which is known for its efficiency in reading text files.
Example of Basic File Reading
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class BasicFileReader {
public static void main(String[] args) {
String filePath = "example.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- BufferedReader: This class wraps a
FileReader
and improves efficiency by buffering characters, thus reducing the number of I/O operations. - try-with-resources: This ensures that the
BufferedReader
is closed automatically, preventing memory leaks. - Reading Line by Line: This method reads files line by line, which is memory efficient for large files.
While the basic approach is effective, there are several ways to further optimize file reading.
1. Avoid Loading Entire Files into Memory
One of the fastest ways to use up memory is by loading entire files into memory. Instead, read files in chunks or lines. For example, when dealing with large CSV files, read them line by line or in blocks.
Example of Chunk-Based Reading
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ChunkFileReader {
private static final int CHUNK_SIZE = 1024; // 1KB
public static void main(String[] args) {
String filePath = "largefile.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
char[] buffer = new char[CHUNK_SIZE];
int bytesRead;
while ((bytesRead = br.read(buffer, 0, CHUNK_SIZE)) != -1) {
System.out.println(new String(buffer, 0, bytesRead));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- Buffer Size: We define a buffer size of 1KB, allowing us to read and process data in manageable chunks rather than loading the entire file.
- Adaptive Memory Usage: This method adapts to the available memory, allowing better scalability.
2. Use NIO for Better Performance
Java's New I/O (NIO) package provides advanced file input/output capabilities and can significantly improve performance for large files.
Example Using NIO
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class NioFileReader {
public static void main(String[] args) {
String filePath = "largefile.txt";
try {
List<String> lines = Files.readAllLines(Paths.get(filePath));
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- Files.readAllLines: This method reads all lines at once into a list, which is practical for files that can fit in memory, but less efficient for larger ones.
- NIO Advantages: It employs memory-mapped files and buffers, resulting in improved performance and resource management.
3. Stream Processing with Java 8+
Java 8 introduced the Stream API, enabling functional-style processing of sequences of elements, including reading files as streams.
Example Using Stream API
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
public class StreamFileReader {
public static void main(String[] args) {
String filePath = "largefile.txt";
try (Stream<String> lines = Files.lines(Paths.get(filePath))) {
lines.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- Files.lines: This method returns a Stream that lazily reads lines from a file, minimizing memory usage since it doesn’t load the entire file into memory.
- ForEach: Functional programming style encourages clearer code and can simplify operations like filtering or mapping.
4. Consider Memory-Mapping Files
For extremely large files, memory-mapped files can be an effective technique. This allows a program to read a portion of the file directly into memory as if it were an array.
Example Using Memory-Mapped Files
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
public class MappedFileReader {
public static void main(String[] args) {
String filePath = "largefile.txt";
try (RandomAccessFile file = new RandomAccessFile(filePath, "r");
FileChannel channel = file.getChannel()) {
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_ONLY, 0, channel.size());
while (buffer.hasRemaining()) {
System.out.print((char) buffer.get());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- MappedByteBuffer: This allows for direct file access without loading it entirely into memory, optimizing performance.
- Use Case: Ideal for very large files where only specific sections need to be accessed.
Additional Resources
For progressive file reading techniques, check out the official Java documentation on Java NIO and Stream API.
My Closing Thoughts on the Matter
Optimizing file reading in Java is essential for efficient memory usage and performance. By adopting methods like chunk-based reading, NIO, stream processing, and memory-mapping, programmers can effectively handle even the largest datasets while ensuring that their applications remain responsive and resource-efficient.
Incorporate these techniques to refine your file reading practices, and contribute to developing scalable Java applications. Happy coding!