Streamline Your Java Methods: Simplifying Parameter Overload
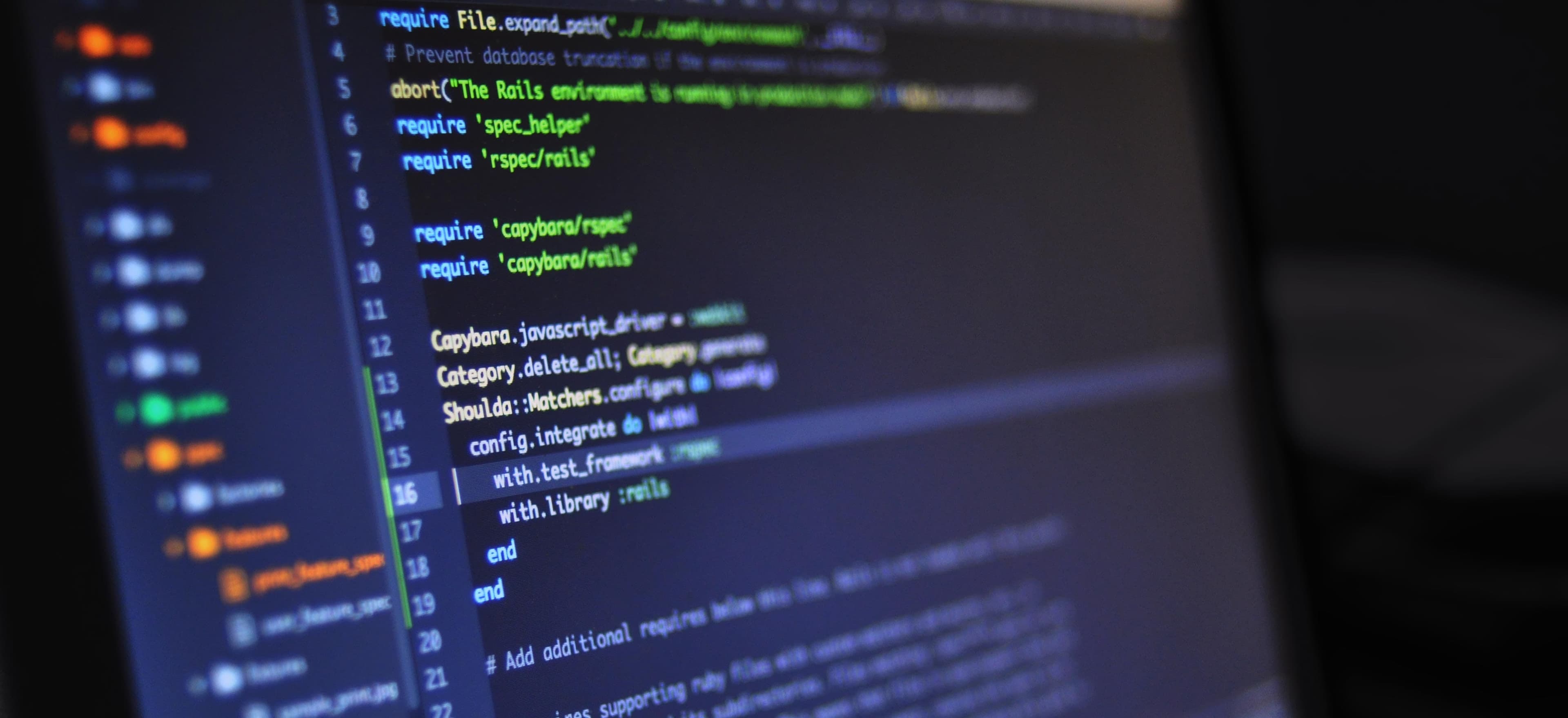
- Published on
Streamline Your Java Methods: Simplifying Parameter Overload
Java is a robust language favored for its object-oriented programming paradigm, but it can sometimes lead to complex method signatures that can hinder readability and maintainability. Lack of clarity from overloaded methods can infuriate developers and cause bugs. In this blog post, we'll explore strategies to simplify parameter overload, thus making your Java methods clearer and more efficient.
Understanding Parameter Overload
Parameter overloading occurs when two or more methods in the same class have the same name but different parameters. This allows developers to use the same method name but with different data types or numbers of arguments. While the concept offers flexibility, it can lead to confusion, especially for others reviewing your code or even for you after some time has passed.
Example of Parameter Overload
Consider the following overloaded methods:
public void drawCircle(int radius) {
// Draw a circle with the specified radius
}
public void drawCircle(int x, int y, int radius) {
// Draw a circle at the specified coordinates with the specified radius
}
public void drawCircle(double radius) {
// Draw a circle with a double precision radius
}
At first glance, this implementation seems efficient. However, when someone reads this code without context, understanding which drawCircle
method to call can be tricky.
The Problem with Parameter Overloading
While overloaded methods can reduce the number of unique method names, they can also lead to ambiguity. If the method signatures are not distinct enough, it can produce unexpected behaviors.
To demonstrate, let's look at a case when a double value could be interpreted as either an integer radius or a double radius. This can lead to unintentional method calls that could break the code, especially if later versions alter method signatures.
Streamlining Your Java Methods
1. Use Builder Pattern
One effective way to avoid parameter overload is to utilize the Builder Pattern. This design pattern involves creating a separate Builder class for constructing objects with a large number of optional parameters. This way, each object can be configured in a clear, readable manner.
Here is a simple implementation of the Builder Pattern:
public class Circle {
private int x;
private int y;
private double radius;
private Circle(Builder builder) {
this.x = builder.x;
this.y = builder.y;
this.radius = builder.radius;
}
public static class Builder {
private int x = 0; // default value
private int y = 0; // default value
private double radius = 1.0; // default value
public Builder setX(int x) {
this.x = x;
return this;
}
public Builder setY(int y) {
this.y = y;
return this;
}
public Builder setRadius(double radius) {
this.radius = radius;
return this;
}
public Circle build() {
return new Circle(this);
}
}
}
Why Use Builder Pattern?
The primary advantage of using the Builder Pattern is the increase in code readability. Each method in the Builder class clearly states its purpose, allowing you to create Circle
objects dynamically with only the parameters you need.
Circle circle1 = new Circle.Builder().setRadius(5.0).build();
Circle circle2 = new Circle.Builder().setX(10).setY(10).setRadius(3.5).build();
2. Method Overloading with Varargs
In Java, you can use variable-length arguments (varargs) as a way to simplify method overloads. Varargs allow you to pass an arbitrary number of arguments to a method.
public void drawShapes(String shapeType, double... dimensions) {
if ("Circle".equals(shapeType)) {
// Expecting dimensions[0] as the radius
drawCircle(dimensions[0]);
} else if ("Rectangle".equals(shapeType)) {
// Expecting dimensions[0] as width, dimensions[1] as height
drawRectangle(dimensions[0], dimensions[1]);
}
}
Why Use Varargs?
Varargs improve your method's flexibility without needing multiple overloaded versions. The code becomes more straightforward because you're capturing all necessary parameters in a single method.
3. Use Optional Parameters with Java 8+
In Java 8 and later, you can also use the Optional
class to define parameters that may or may not be provided. This can effectively reduce the need for overloaded methods.
import java.util.Optional;
public void drawCircle(Optional<Integer> x, Optional<Integer> y, int radius) {
int finalX = x.orElse(0); // default to 0
int finalY = y.orElse(0); // default to 0
// Draw circle using finalX, finalY, and radius
}
Why Use Optional?
The use of Optional
clarifies which parameters are optional, thus making your method easier to understand and use correctly. It reduces ambiguity, allowing for defaults without cluttering your code with multiple overloaded methods.
4. Use Intuitive Naming for Methods
If you have multiple methods that require different parameters, using descriptive names can greatly enhance clarity. Instead of overloading a method based solely on its parameters, consider breaking down functionality:
public void drawCircle(int radius) {
// implementation
}
public void drawCircleAt(int x, int y, int radius) {
// implementation
}
public void drawCircleWithPrecision(double radius) {
// implementation
}
By using distinct method names, you make it instantly clear what each method does, and this can help avoid confusion when reading or maintaining code.
Key Takeaways
Simplifying parameter overload in Java methods doesn't merely improve code readability—it enhances maintainability and reduces the likelihood of bugs. By implementing design patterns like the Builder Pattern, utilizing varargs, employing Optional parameters, and adopting intuitive naming conventions, you can streamline your methods effectively.
For additional context on design patterns, you can check out this comprehensive guide that delves deeper into how various patterns can optimize your code structure.
By understanding the principles of method simplification, we pave the way for cleaner, more efficient code that is easier to read and maintain. Embrace these strategies today and elevate your Java programming skills to new heights.