Mastering Java Streams: Common Pitfalls to Avoid
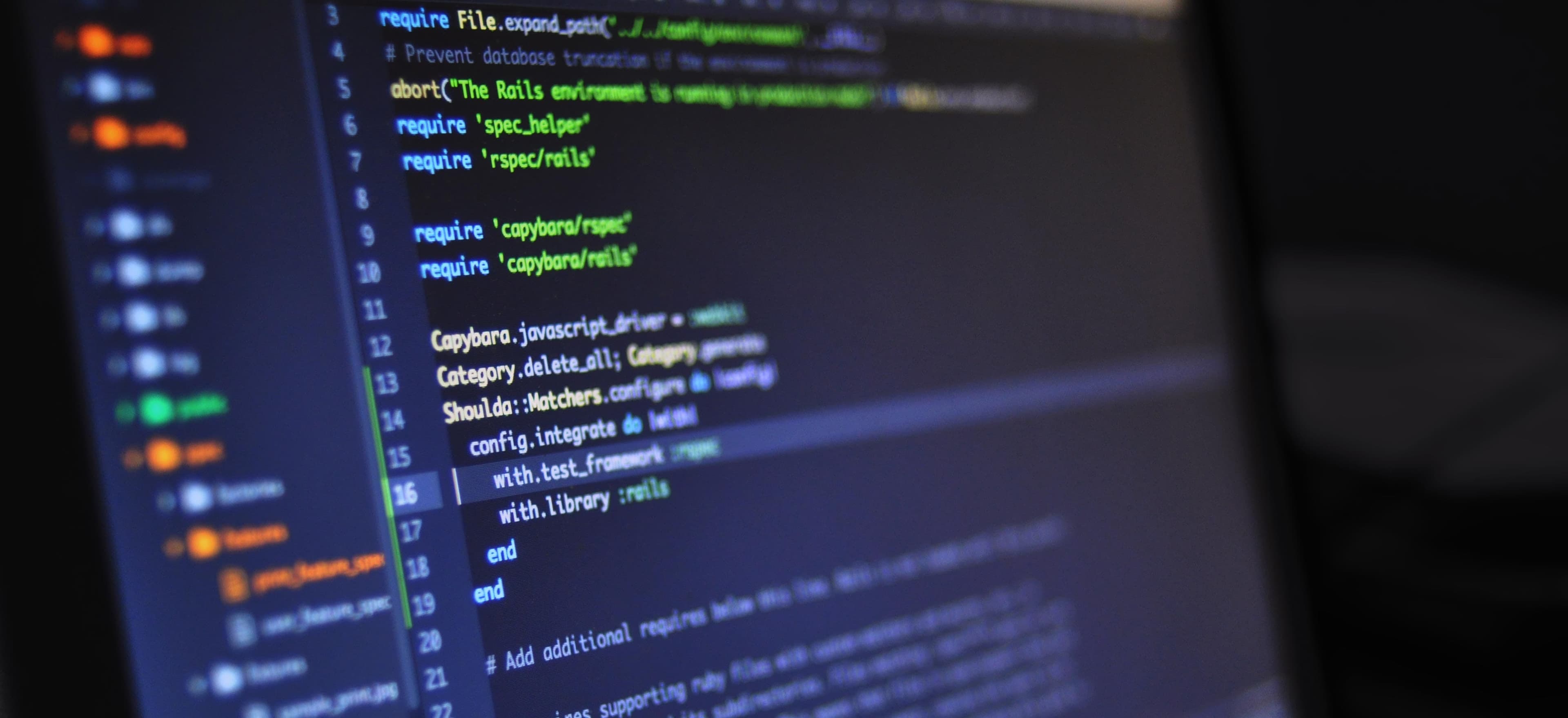
- Published on
Mastering Java Streams: Common Pitfalls to Avoid
Java introduced the Stream API in version 8, revolutionizing the way we process collections. With streams, we can write clean and readable code by using a functional approach. However, like any powerful feature, streams can lead to pitfalls if not used correctly. In this post, we will explore common mistakes programmers make when working with Java Streams and how to avoid them.
Understanding Streams
Before we dive into the pitfalls, let's briefly summarize what Java Streams are. A stream is a sequence of elements that can be processed in parallel or sequentially. Streams support functional-style operations like map, filter, and reduce.
Here's a basic example of a stream that processes a list of integers:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.filter(n -> n % 2 == 0) // filters even numbers
.forEach(System.out::println); // prints each even number
}
}
In the example above, we create a stream from a list of integers, filter the even numbers, and print them. This showcases the power of streams in creating concise code that expresses intentions clearly.
Common Pitfalls in Java Streams
While streams can streamline our code, there are a few common pitfalls to avoid.
1. Not Understanding Lazy Evaluation
One of the critical features of streams is lazy evaluation. Operations on streams are not executed until a terminal operation is invoked. This design improves performance, but it can lead to confusion.
Pitfall: Developers may expect that intermediate operations are executed immediately.
Best Practice: Familiarize yourself with the difference between intermediate and terminal operations. Intermediate operations (like filter
or map
) return a new stream and do not consume the source until a terminal operation (like forEach
or collect
) is called.
Here's how we can clarify this:
import java.util.Arrays;
import java.util.List;
public class LazyEvaluationExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Intermediate operation (does not execute)
names.stream()
.filter(name -> {
System.out.println("Filtering: " + name); // Will not print until terminal operation
return name.length() > 3;
})
.collect(Collectors.toList()); // Terminal operation - now the code is executed
}
}
2. Using Streams for Side Effects
Streams are designed to favor functional programming principles, where functions should ideally be pure — meaning they should not have side effects.
Pitfall: Developers may introduce side effects within the stream operations, causing unexpected behavior.
Best Practice: Avoid modifying external state within the stream's pipeline. Instead, collect or transform data and process it afterward.
Example of a side effect that should be avoided:
import java.util.Arrays;
import java.util.List;
public class SideEffectExample {
private static int counter = 0;
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Side effect: modifying external variable
numbers.stream()
.forEach(n -> counter++);
System.out.println("Count of numbers processed: " + counter); // Can lead to bugs
}
}
Instead, aim to return results instead of modifying external values.
3. Not Using Parallel Streams Appropriately
Java streams can be executed in parallel using parallelStream()
, which may improve performance in some cases.
Pitfall: Misusing parallel streams can lead to performance issues due to overhead from context switching.
Best Practice: Use parallel streams for larger datasets or CPU-intensive operations. For smaller collections, the overhead may negate any performance gain.
Example of correctly using parallel streams:
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Using parallel stream for potentially better performance on large dataset
numbers.parallelStream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
}
}
4. Forgetting to Handle State Changes Correctly
Mutable objects should be handled cautiously when using streams, as they can lead to unpredictability.
Pitfall: Modifying mutable objects during stream operations can yield inconsistent results.
Best Practice: Prefer immutable objects or use defensive copies when working with streams.
Example of mutable object pitfalls:
import java.util.Arrays;
import java.util.List;
public class MutableStateExample {
static class User {
String name;
int age;
User(String name, int age) {
this.name = name;
this.age = age;
}
}
public static void main(String[] args) {
List<User> users = Arrays.asList(new User("Alice", 20), new User("Bob", 22));
// Modifying state inside the stream
users.stream()
.map(user -> {
user.age += 1; // Warning: This modifies the original object
return user;
})
.forEach(user -> System.out.println(user.name + " is now " + user.age));
}
}
5. Not Considering Order of Operations
Order of operations matters when using streams, especially regarding state changes and accumulation.
Pitfall: Incorrectly chaining operations can lead to unexpected results.
Best Practice: Clearly define your intention and handle transformations in a logical order.
For example:
import java.util.Arrays;
import java.util.List;
public class OrderOfOperationsExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Correct order of operations: map first, then filter
names.stream()
.map(String::toUpperCase)
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
}
}
Closing Remarks
Mastering Java Streams is essential for writing efficient and maintainable code, but it requires an understanding of their design and behavior. By avoiding the pitfalls we discussed, you can leverage the power of Java Streams effectively.
For those seeking deeper knowledge, consider checking out Java's official documentation or community-driven guides like Baeldung's Java Stream API tutorial.
Whether you're optimizing performance or striving for elegance, Java Streams can be a powerful ally—if wielded correctly. Happy coding!