Overcoming CDI 2.0 Compatibility Challenges in JSR-365
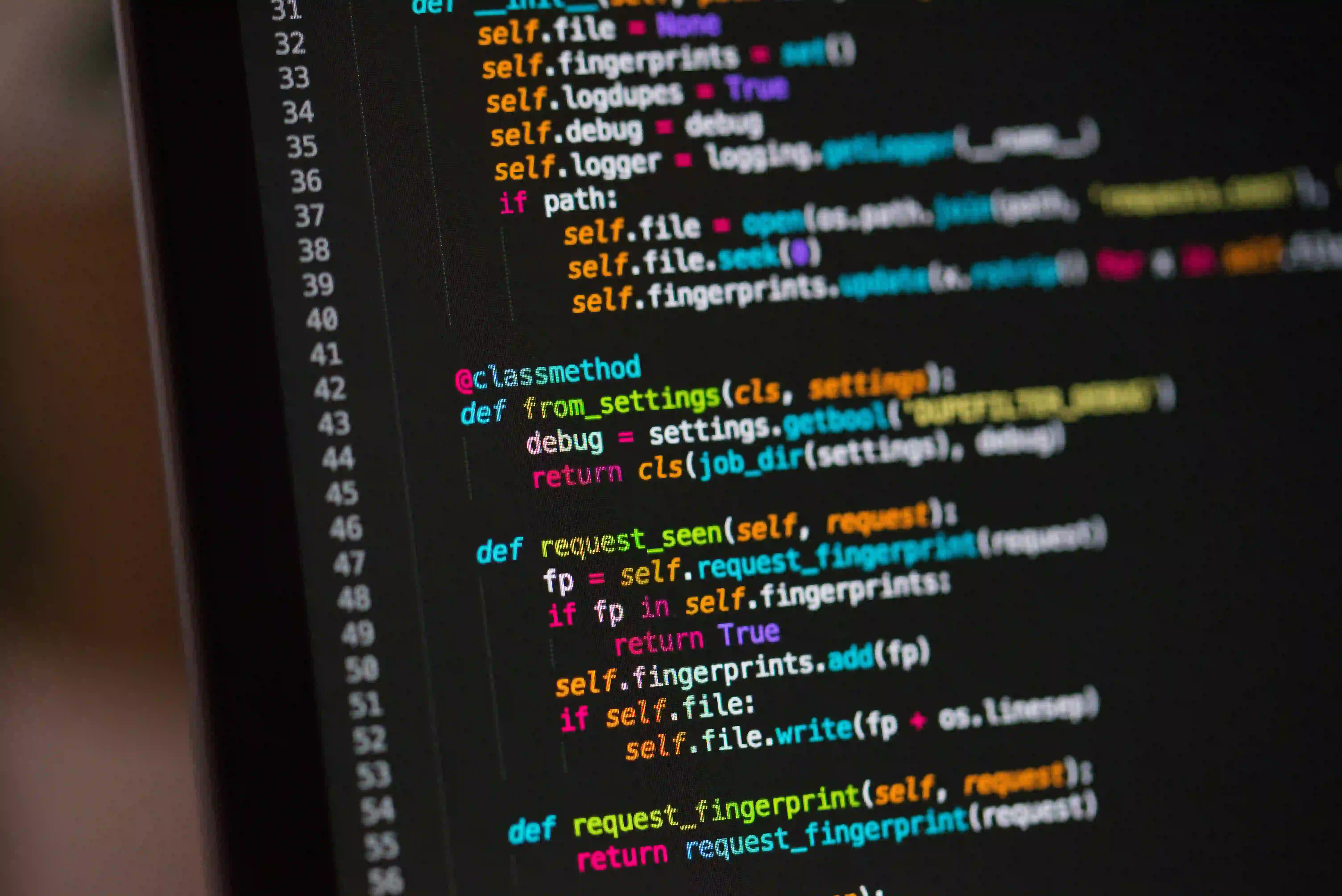
Overcoming CDI 2.0 Compatibility Challenges in JSR-365
Java developers are continuously striving to utilize modern frameworks and specifications that enhance the development experience while ensuring robust application performance. One such framework is Contexts and Dependency Injection (CDI), which promotes a more flexible approach to managing dependencies and contexts within Java applications. With the advent of CDI 2.0 and the JSR-365 specification, there are compatibility challenges that developers must navigate to leverage these enhancements.
In this blog post, we will discuss the key aspects of CDI 2.0, explore compatibility challenges, and provide practical insights to enable a seamless transition. Let’s jump right in!
What is CDI 2.0?
CDI, or Contexts and Dependency Injection, is a powerful dependency injection framework for Java EE (Enterprise Edition) applications. With CDI 2.0, several new features were introduced that improve modularity, portability, and overall ease of integration with other frameworks, notably:
- Improved Event Handling: CDI 2.0 simplifies event notification capabilities, allowing for better decoupling of components.
- Integration with Java SE: CDI can now function outside of the Java EE container, making it suitable for Java SE applications.
- Better Interoperability: Enhanced interoperability with other frameworks such as JAX-RS.
- New Configuration Options: Providing more options for integrating service discovery.
You can read more about the specifications of CDI 2.0 in the official JSR-365 documentation.
The Challenges with Compatibility
Transitioning to CDI 2.0 may not be as smooth as anticipated. The following challenges commonly arise:
1. Legacy Code Dependency
Many applications consist of legacy code that was designed with CDI 1.2 in mind. Upgrading such systems may require substantial modifications, which can introduce bugs if not handled carefully.
Example of Legacy Code:
@Inject
private MyLegacyService myLegacyService;
Why It Might Break: If MyLegacyService
uses an outdated lifecycle method that has changed in CDI 2.0, it may cause runtime exceptions.
2. Component Scope Changes
CDI 2.0 introduces new component scope annotations that may not be supported in CDI 1.2. Applications that rely on specific scopes (e.g., @SessionScoped
, @RequestScoped
) will need to examine the implications of switching to new or modified scopes.
3. Event System Modifications
The event system in CDI 2.0 has undergone enhancements. If your legacy applications are heavily reliant on the old event system, upgrading may cause them to behave unexpectedly.
Updated Event Handling Example:
@Observes
public void onUserRegistration(@Observes UserRegistrationEvent event) {
// Handle the user registration event
}
Why This Matters: By adopting stronger event typing, CDI 2.0 adds type safety. However, moving from loosely-typed to strongly-typed events requires careful consideration of existing event handling logic.
4. Integration with Other Frameworks
Given that CDI 2.0 enhances its interoperability credentials, it might clash with older versions of integrated frameworks. For instance, if an application combines CDI with older JAX-RS versions, inconsistencies might arise.
5. Configuration Issues
Introduced mostly through new configuration properties, some applications might run into issues while trying to set up their CDI environment.
Example Configuration Change:
<beans xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/beans_2_0.xsd"
bean-discovery-mode="all">
<!-- Beans configuration -->
</beans>
Why Keeping Configuration Updated is Important: Adhering to the correct XML schema is vital to ensure compatibility. Otherwise, errors can prevent the application from loading the proper beans.
Strategies for Overcoming Compatibility Challenges
Navigating compatibility issues requires a blend of prudence, structured planning, and testing. Here’s how to ensure a smooth transition:
1. Refactor Legacy Code
Identify legacy implementations in your code that depend on old CDI methodologies. Refactor or redesign these components, aligning them with the new feature set provided by CDI 2.0.
2. Gradual Migration Strategy
Perform a phased upgrade by isolating changes into small, manageable sections. This makes it easier to monitor for errors and ensures swift resolution.
3. Utilize CDI 2.0's Enhanced Features
Leverage the new features of CDI 2.0, such as event handling and configuration options. This will not only reduce the complexity of your application but also provide you with benefits that weren’t previously available.
4. Comprehensive Testing
Implement robust testing protocols to check for regression or integration issues. Utilize unit and integration tests to perform checks continuously before and after the migration.
@Test
public void testUserRegistrationEvent() {
// Simulate registration event
UserRegistrationEvent event = new UserRegistrationEvent();
myService.onUserRegistration(event);
// Perform assertions
assertTrue(myService.wasCalled());
}
Why Testing is Essential: Given the complexity of changes across different areas, tests can catch unintended discrepancies that might occur during the transition.
5. Continuous Monitoring Post-Migration
After migration, monitor your application closely. Pay attention to error logs and performance metrics to quickly identify any issues arising from the upgrade.
Final Considerations
Transitioning to CDI 2.0 under JSR-365 presents both opportunities and challenges for Java developers. By understanding the potential compatibility hurdles and implementing a structured approach to migration, development teams can harness the power of CDI 2.0 while mitigating risks.
As Java continues to evolve, embracing new frameworks and standards like CDI 2.0 will greatly improve the quality and maintainability of applications in the long run. Stay current with the latest Java EE advancements and ensure your applications can leverage the full potential of CDI.
With thoughtful planning and strategic execution, overcoming the hurdles of CDI 2.0 compatibility can transform your Java applications to be more robust, flexible, and adaptable. Happy coding!