How to Enable GC Logging Dynamically for Better Performance
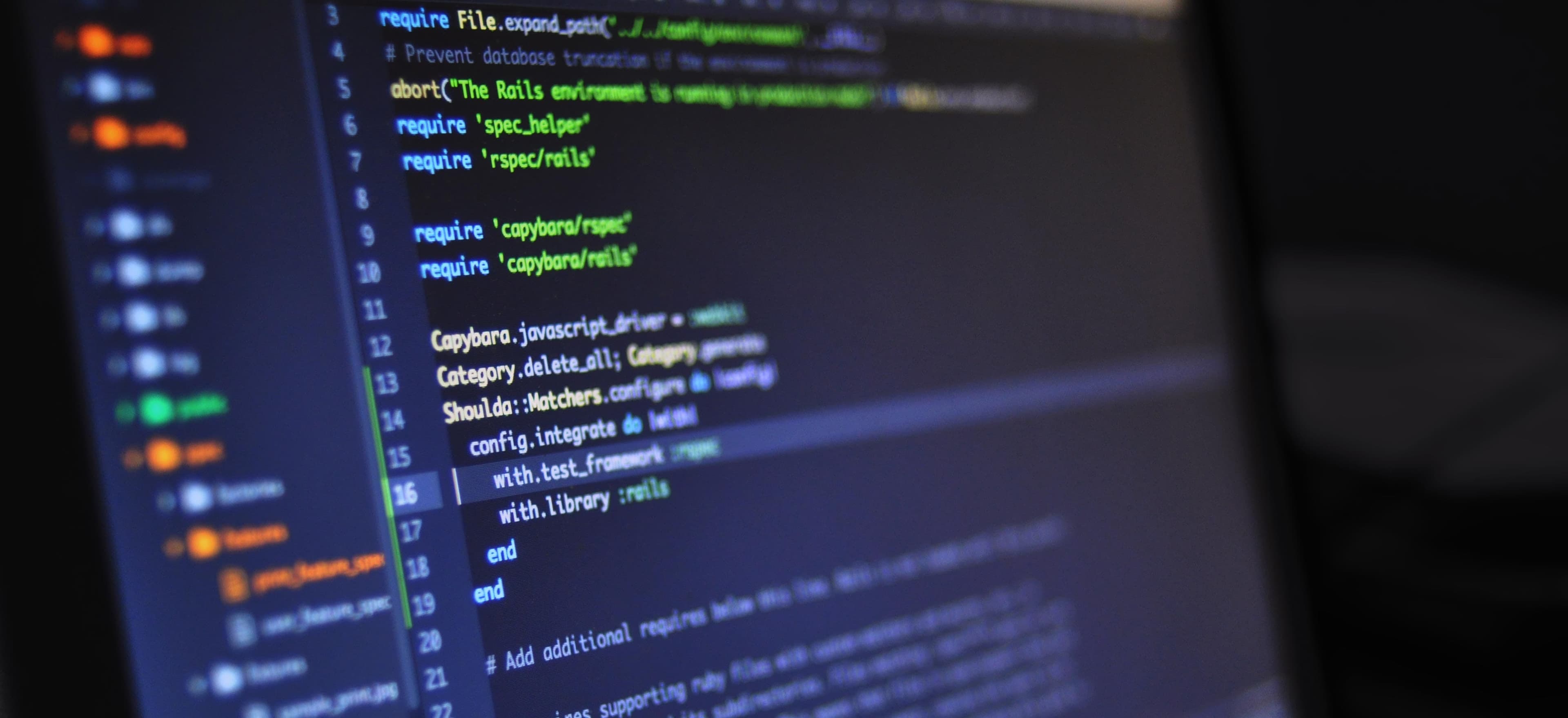
- Published on
How to Enable GC Logging Dynamically for Better Performance in Java
Garbage collection is a critical aspect of Java's memory management system. Properly managing memory can lead to improved application performance, reduced latency, and happier users. In this blog post, we will explore the process of enabling garbage collection (GC) logging dynamically in Java. We will discuss why GC logging is essential, how to enable it at runtime, and the tools that can help you analyze the logs effectively.
What is GC Logging?
GC logging provides insights into the garbage collection process in your Java application. By analyzing these logs, developers can identify memory usage patterns, pinpoint memory leaks, and optimize the performance of their applications.
GC logs contain detailed information about various aspects of the garbage collection process, including:
- Types of collections (minor, major, and full)
- Durations of each collection
- Memory usage before and after collections
- Frequency of collections
Why GC Logging Matters
Understanding how Java performs garbage collection can help you:
- Identify performance bottlenecks: Long GC pauses can lead to noticeable delays in application responsiveness.
- Optimize application performance: By analyzing the frequency and duration of GC pauses, you can fine-tune your application's memory settings.
- Debug memory leaks: GC logs can indicate when objects are not being collected as expected, potentially pointing to memory leaks.
Enabling GC Logging Dynamically
Starting with JDK 9, Java has made it easier to enable GC logging dynamically. Let’s see how you can enable it without needing to restart your application.
Step 1: Check Your Java Version
First, ensure you are running Java 9 or later. You can check your Java version by running the following command in your terminal or command prompt:
java -version
Step 2: Enable GC Logging in a Running Application
You can enable GC logging dynamically using jcmd
, a command-line utility that enables detailed diagnosis of JVM applications. Here’s how you can do this:
-
Find the Process ID (PID) of your Java application:
jps -l
The
jps
command will display a list of Java processes along with their PIDs. -
Enable GC logging using the following command:
jcmd <PID> VM.gc_logging 1
Replace
<PID>
with the actual process ID you retrieved in the previous step. -
Set the logging configuration (if needed). The GC logging parameters are typically set at JVM startup, but you can dynamically configure them as follows:
jcmd <PID> VM.option -XX:+PrintGCDetails
-
To disable GC logging, you can run:
jcmd <PID> VM.gc_logging 0
This method allows for easy configuration during runtime, making it flexible for developers to adjust as needed.
Understanding Key Parameters
When enabling GC logging, it’s crucial to understand various parameters you can set to customize the log outputs effectively. Here’s a breakdown of some popular flags:
-XX:+PrintGC
: Enables logging for garbage collection events.-XX:+PrintGCDetails
: Provides detailed information about each garbage collection event, including memory usage.-XX:+PrintGCTimeStamps
: Includes timestamps for each GC event, allowing you to correlate events with application behavior.
Example Code Snippet with GC Logging
To implement GC logging in your code during the JVM startup, add the following flags in your Java command:
java -XX:+PrintGC -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log -jar myapp.jar
Output Sample
After running your application with GC logging enabled, you’ll see an output similar to the following in your gc.log
file:
[GC (Allocation Failure) 2048K->1024K(8192K), 0.0123456 secs]
[Full GC (Ergonomics) 1024K->512K(8192K), 0.0456789 secs]
In this snippet:
- The logs indicate the type of garbage collection (e.g., Full GC).
- The old size, new size, and memory remaining are evident.
- Duration for each garbage collection event provides insights into how long it took.
Tools for Analyzing GC Logs
While raw log files can be helpful, they may be somewhat challenging to interpret. Here are a few tools that can help you better analyze GC logs:
-
GCViewer: A graphical tool that provides visual insights into your GC logs, helping you to analyze trends and patterns. GCViewer GitHub
-
GCEasy: An online tool that allows you to upload your GC log files for analysis. It provides graphical representations and easily interpretable insights. GCEasy
-
VisualVM: A versatile tool that includes profiling and monitoring capabilities alongside the ability to review GC performance. VisualVM
The Closing Argument
Dynamic GC logging is an invaluable feature of the Java programming language, especially for developers aiming for high-performance applications. By enabling and configuring GC logs, you can gain insights into memory management behaviors, identify performance bottlenecks, and optimize your application’s performance.
Implementing GC logging can seem complex at first, but with the right tools and understanding, you can unravel the mysteries behind your application's garbage collection process. Remember to leverage tools like GCViewer, GCEasy, and VisualVM for a more accessible and visual approach to GC log analysis.
For more insights into Java performance and memory management, be sure to check out the official Java documentation or other community resources. Happy coding!