Securing Your Stateless Spring Application
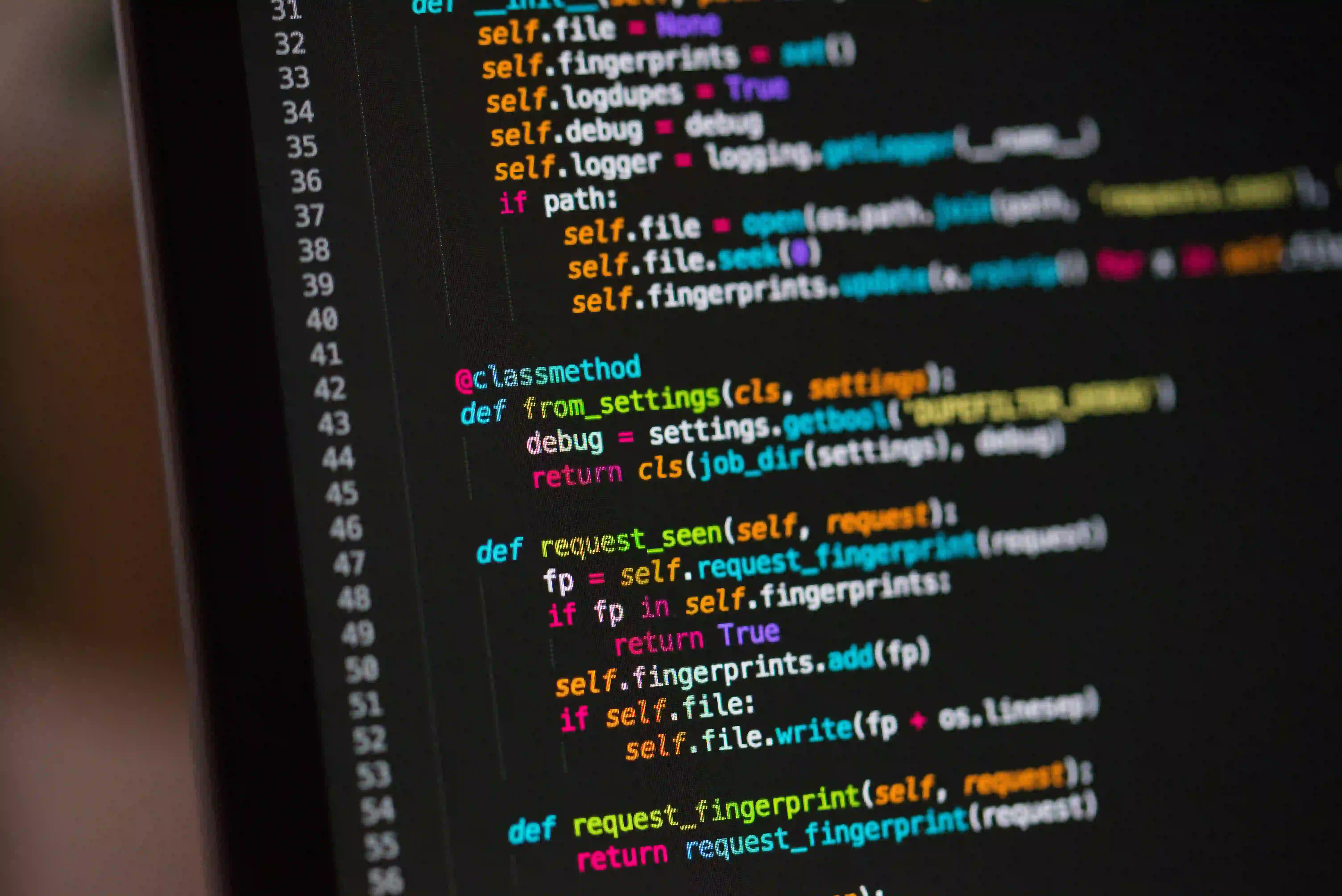
Securing Your Stateless Spring Application
When it comes to building web applications, security is a critical aspect that cannot be overlooked. In the world of Java development, Spring Security has emerged as a powerful and flexible framework for securing Java applications. In this blog post, we will explore how to secure a stateless Spring application using Spring Security.
Understanding Stateless Authentication
Before we dive into securing our Spring application, it's important to understand the concept of stateless authentication. In a stateless authentication mechanism, the server does not store any session information for the client. Each request from the client contains all the necessary information for the server to authenticate and authorize the request.
Stateless authentication is commonly used in RESTful APIs where each request is independent and should contain all the required authentication information. This approach makes scaling easier and reduces the overhead of managing session state on the server.
Setting Up Spring Security
To get started with securing our Spring application, we'll first need to add the Spring Security dependency to our project. We can do this by adding the following dependency to our pom.xml
file for Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
If you're using Gradle, you can add the following dependency to your build.gradle
file:
implementation 'org.springframework.boot:spring-boot-starter-security'
Once the Spring Security dependency is added, Spring Boot will automatically configure some sensible defaults for securing our application.
Configuring Security for Stateless Authentication
Since we are building a stateless application, we'll need to configure our security to work in a stateless manner. We can do this by creating a SecurityConfig
class that extends WebSecurityConfigurerAdapter
and overrides the configure
method to configure our security settings.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated();
}
}
In the above code snippet, we have configured our SecurityConfig
class to disable CSRF protection (as it's not relevant for stateless authentication), set the session creation policy to STATELESS
, and defined that requests to /api/public/**
are permitted without authentication, while any other requests require authentication.
Implementing Stateless Authentication
With our security configuration in place, we can now implement the actual authentication logic for our application. For the purpose of this demonstration, let's assume we have a simple UserController
that exposes a /login
endpoint for users to authenticate.
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private JwtTokenProvider jwtTokenProvider;
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest request) {
Authentication authentication = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(request.getUsername(), request.getPassword())
);
SecurityContextHolder.getContext().setAuthentication(authentication);
String token = jwtTokenProvider.generateToken(authentication);
return ResponseEntity.ok(new JwtAuthenticationResponse(token));
}
}
In the UserController
, we inject an AuthenticationManager
and a JwtTokenProvider
. When a user makes a POST
request to the /login
endpoint with their username and password, the login
method attempts to authenticate the user using the injected AuthenticationManager
. If the authentication is successful, a JWT token is generated using the JwtTokenProvider
and returned in the response.
Using JWT for Stateless Authentication
JSON Web Tokens (JWT) are a popular choice for implementing stateless authentication. A JWT is a compact, URL-safe means of representing claims to be transferred between two parties. In our Spring application, we can use the JwtTokenProvider
to generate and validate JWT tokens.
Here's an example of the JwtTokenProvider
class:
@Component
public class JwtTokenProvider {
@Value("${app.jwtSecret}")
private String jwtSecret;
@Value("${app.jwtExpirationInMs}")
private int jwtExpirationInMs;
public String generateToken(Authentication authentication) {
User principal = (User) authentication.getPrincipal();
Date now = new Date();
Date expiryDate = new Date(now.getTime() + jwtExpirationInMs);
return Jwts.builder()
.setSubject(Long.toString(principal.getId()))
.setIssuedAt(new Date())
.setExpiration(expiryDate)
.signWith(SignatureAlgorithm.HS512, jwtSecret)
.compact();
}
public Long getUserIdFromToken(String token) {
Claims claims = Jwts.parser()
.setSigningKey(jwtSecret)
.parseClaimsJws(token)
.getBody();
return Long.parseLong(claims.getSubject());
}
public boolean validateToken(String authToken) {
try {
Jwts.parser().setSigningKey(jwtSecret).parseClaimsJws(authToken);
return true;
} catch (SignatureException ex) {
// Invalid JWT signature
} catch (MalformedJwtException ex) {
// Invalid JWT token
} catch (ExpiredJwtException ex) {
// Expired JWT token
} catch (UnsupportedJwtException ex) {
// Unsupported JWT token
} catch (IllegalArgumentException ex) {
// JWT claims string is empty
}
return false;
}
}
In the JwtTokenProvider
class, we make use of the io.jsonwebtoken.Jwts
library to create, parse, and validate JWT tokens. We also use the @Value
annotation to inject the JWT secret and expiration time from our application properties.
Securing Endpoints with JWT
Now that we have implemented stateless authentication using JWT, we can secure our endpoints by validating the JWT token provided in the request. This can be achieved by creating a filter that intercepts incoming requests and validates the JWT.
@Component
public class JwtAuthenticationFilter extends OncePerRequestFilter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain)
throws ServletException, IOException {
String token = getJwtFromRequest(request);
if (StringUtils.hasText(token) && jwtTokenProvider.validateToken(token)) {
Long userId = jwtTokenProvider.getUserIdFromToken(token);
UserDetails userDetails = customUserDetailsService.loadUserById(userId);
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
SecurityContextHolder.getContext().setAuthentication(authentication);
}
filterChain.doFilter(request, response);
}
private String getJwtFromRequest(HttpServletRequest request) {
String bearerToken = request.getHeader("Authorization");
if (StringUtils.hasText(bearerToken) && bearerToken.startsWith("Bearer ")) {
return bearerToken.substring(7);
}
return null;
}
}
In the JwtAuthenticationFilter
, we extract the JWT token from the request, validate it using the JwtTokenProvider
, and if valid, set the authentication in the SecurityContextHolder
. We can then register this filter to be applied for all incoming requests in our SecurityConfig
:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ...
.addFilterBefore(jwtAuthenticationFilter, UsernamePasswordAuthenticationFilter.class);
}
Final Thoughts
In this blog post, we've learned how to secure a stateless Spring application using Spring Security and JWT. We configured our security settings to work in a stateless manner, implemented authentication logic using JWT, and secured our endpoints by validating JWT tokens.
By following these best practices for securing stateless applications, you can ensure the safety and integrity of your Java web applications. Remember, security is an ongoing process, so always stay updated with the latest security practices and continue to evolve your application's security measures.
For more in-depth information on Spring Security, be sure to check out the official documentation.