Loading images in JavaFX 2 without any hassle
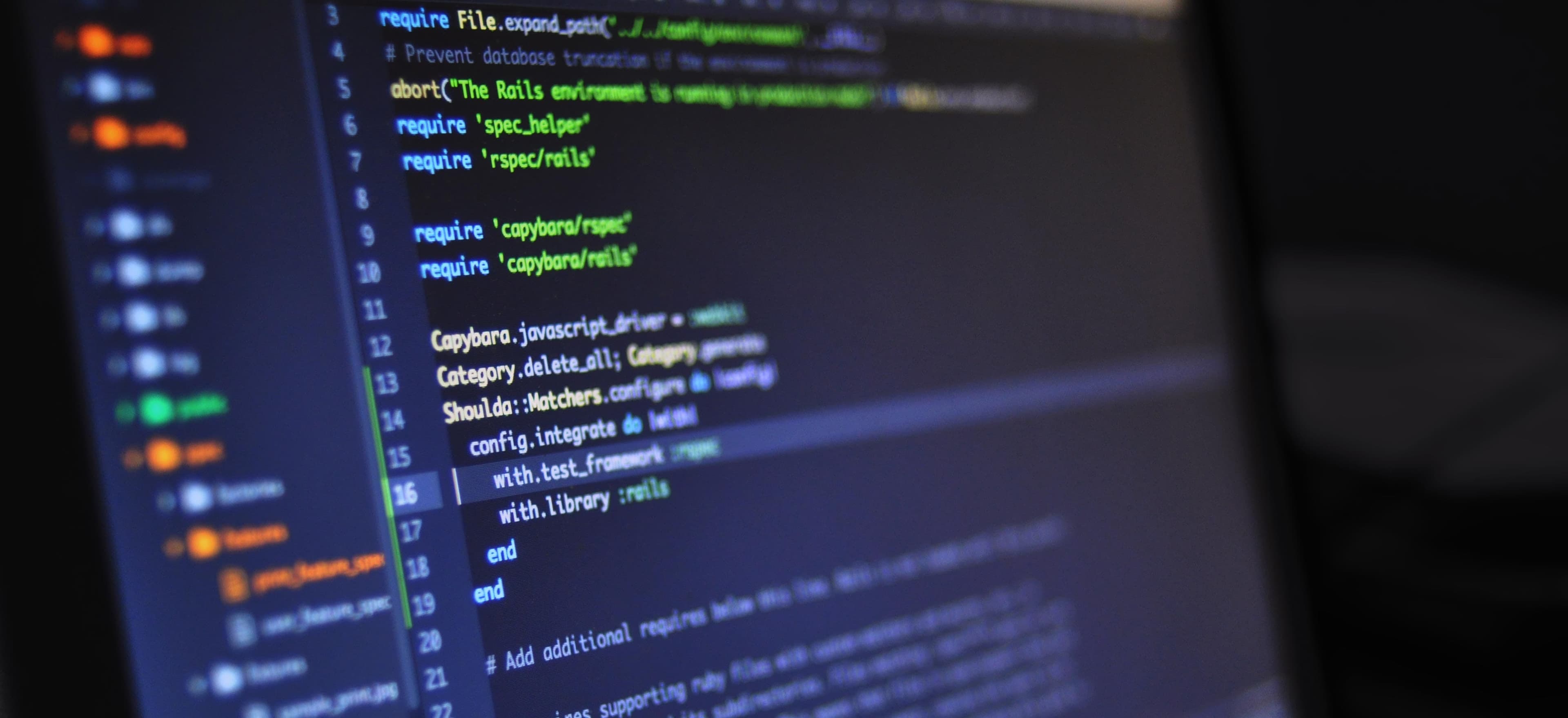
- Published on
Loading Images in JavaFX 2 without Any Hassle
When it comes to creating a visually appealing user interface, JavaFX 2 provides a rich set of features to cater to developers' needs. One common requirement in many applications is the ability to load and display images. In this post, we will discuss the various ways to load images in a JavaFX application without any hassle.
Using Image Class for Simple Image Loading
The Image class in JavaFX is the simplest way to load and display an image. It is part of the javafx.scene.image package and offers constructors for loading images from different sources.
Here's an example of loading an image from a file:
import javafx.scene.image.Image;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class ImageLoader {
public static void main(String[] args) {
try {
Image image = new Image(new FileInputStream("path/to/image.jpg"));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
In the above code, we create a new Image object by passing a FileInputStream of the image file to the constructor. This approach works well for small applications where images are few and can be loaded synchronously.
Asynchronous Image Loading with Background Loading
In larger applications or when dealing with a large number of images, loading images synchronously can lead to unresponsive user interfaces. JavaFX provides a way to load images asynchronously using the javafx.concurrent package.
The Task class allows us to perform background tasks and update the UI when the task is complete. We can use Task to load images in the background and update the UI when the image is ready to be displayed.
Here's an example of asynchronous image loading using Task:
import javafx.application.Application;
import javafx.concurrent.Task;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AsyncImageLoader extends Application {
@Override
public void start(Stage primaryStage) {
Task<Image> imageLoadTask = new Task<>() {
@Override
protected Image call() throws Exception {
return new Image("path/to/image.jpg");
}
};
imageLoadTask.setOnSucceeded(event -> {
Image image = imageLoadTask.getValue();
ImageView imageView = new ImageView(image);
StackPane root = new StackPane(imageView);
primaryStage.setScene(new Scene(root, image.getWidth(), image.getHeight()));
primaryStage.show();
});
new Thread(imageLoadTask).start();
}
public static void main(String[] args) {
launch(args);
}
}
In the above code, we create a Task to load the image in the background. Once the image is loaded, the onSucceeded event is triggered, and we can update the UI with the loaded image.
Using ImageViews for Displaying Images
In JavaFX, the ImageView class is used to display images. It is a part of the javafx.scene.image package and can be added directly to the scene graph for displaying images.
Here's an example of using ImageView to display an image:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ImageViewExample extends Application {
@Override
public void start(Stage primaryStage) {
Image image = new Image("path/to/image.jpg");
ImageView imageView = new ImageView(image);
StackPane root = new StackPane(imageView);
primaryStage.setScene(new Scene(root, image.getWidth(), image.getHeight()));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the above code, we create an ImageView and set the loaded Image as its content. Finally, we add the ImageView to the scene graph to display the image.
Wrapping Up
In this post, we explored various methods to load and display images in a JavaFX application. We discussed using the Image class for simple image loading, asynchronous image loading with background tasks, and displaying images using ImageView. Each approach serves different use cases, and developers can choose the most suitable method based on their application's requirements.
JavaFX's flexibility in image handling empowers developers to create visually stunning user interfaces without the hassle of complex image loading and display mechanisms.
Happy coding!
By applying the approaches discussed in this post, you can elevate the visual elements of your JavaFX applications without compromising performance or user experience. Utilizing the Image class, leveraging asynchronous image loading with Task, and integrating the ImageView class will allow you to effortlessly manage and display images within your JavaFX project. Whether handling a handful of images or dealing with a large repository, these techniques are designed to streamline the image loading process, ultimately enhancing the overall functionality of your application.
Checkout our other articles