The Power of Functional Interfaces in Java 8
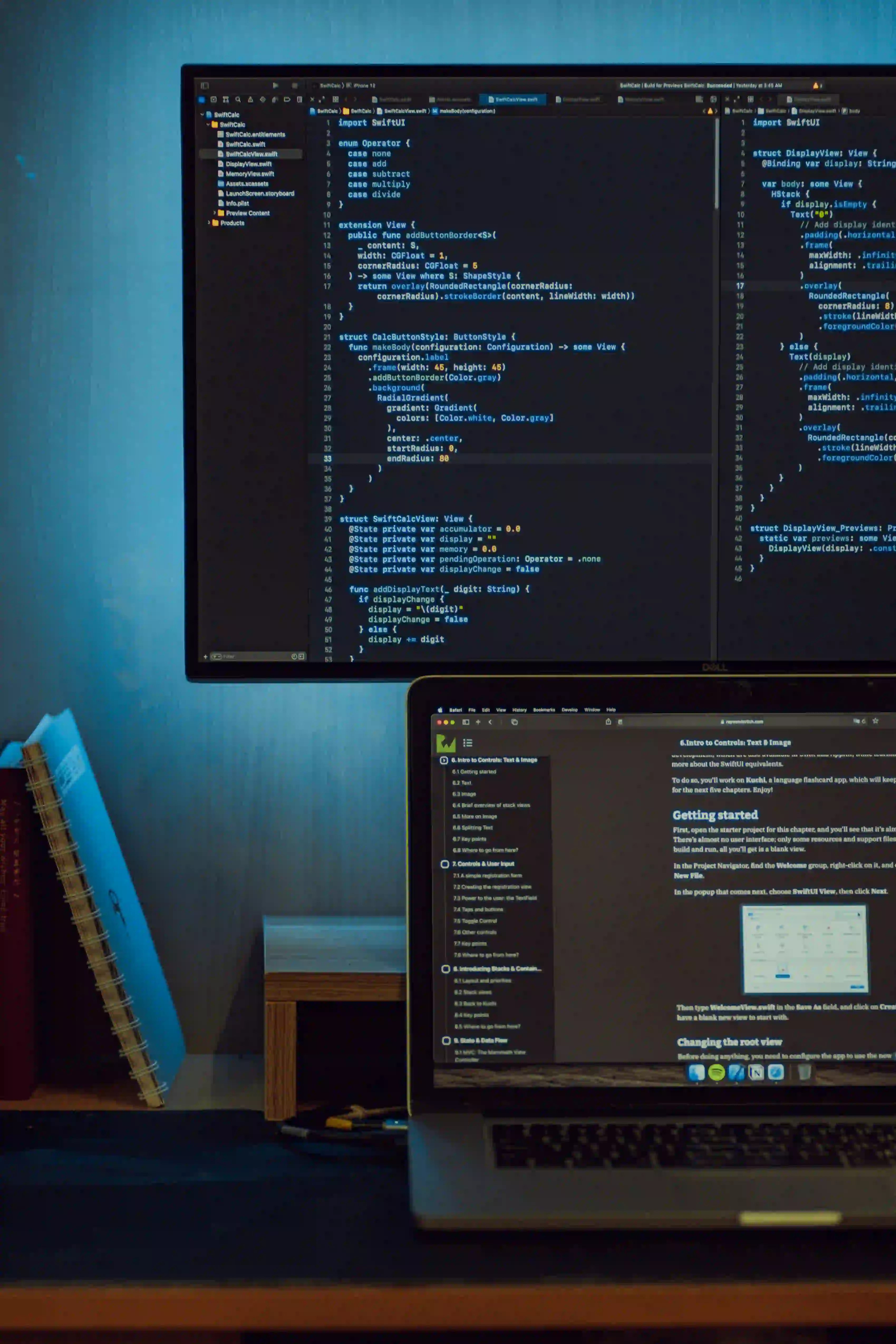
The Power of Functional Interfaces in Java 8
In this blog post, we'll dive into the powerful world of functional interfaces in Java 8. Functional interfaces play a crucial role in the world of functional programming, enabling the use of lambda expressions and method references. We'll explore what functional interfaces are, how they are used, and why they are such a game-changer in Java development.
What are Functional Interfaces?
A functional interface is an interface that contains only one abstract method. Prior to Java 8, interfaces could only declare method signatures, leaving the implementation to the classes that implemented them. However, with the introduction of functional interfaces in Java 8, interfaces can now contain a single abstract method without the need to explicitly declare it as abstract.
@FunctionalInterface
interface MyFunctionalInterface {
void myMethod();
}
The @FunctionalInterface
annotation is optional, but using it explicitly communicates the intent that the interface is intended to be used as a functional interface. This can help prevent accidental addition of more abstract methods in the future, which would violate the functional interface contract.
The Power of Lambda Expressions
Lambda expressions provide a concise way to represent an anonymous function that can be passed around and executed later. They enable you to treat functionality as a method argument or code as data.
MyFunctionalInterface functionalInterface = () -> System.out.println("Hello, Functional Interface!");
functionalInterface.myMethod();
In this example, we have created a lambda expression that implements the single abstract method myMethod
defined in the MyFunctionalInterface
. We have effectively provided the implementation of the interface directly within the lambda expression.
Leveraging Method References
Java 8 also introduced method references, which provide a way to reference methods or constructors without invoking them. This can be particularly useful when implementing functional interfaces that correspond to an existing method.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
In this example, the System.out::println
method reference is passed as the argument to the forEach
method of the List
interface. This results in each element of the list being passed to the println
method.
Why Functional Interfaces Are Game-Changers
Enhanced Readability and Conciseness
Functional interfaces, lambda expressions, and method references collectively contribute to enhanced readability and conciseness of code. They allow developers to express behaviors in a more compact and focused manner, making code easier to understand and maintain.
Improved Code Flexibility
By enabling the treatment of functionality as a method argument, functional interfaces empower developers to write more flexible and reusable code. This makes it easier to pass behavior as an argument and use it in different contexts, ultimately leading to more modular and adaptable code.
Support for Functional Programming Paradigm
The introduction of functional interfaces aligns Java more closely with the functional programming paradigm. This opens up opportunities for leveraging functional programming techniques such as higher-order functions, immutability, and declarative style, which can lead to more robust and scalable applications.
Streamlined Asynchronous Programming
Functional interfaces, in combination with features like CompletableFuture and streams, provide a streamlined way to express asynchronous and concurrent programming patterns. This is particularly valuable in modern application development, where asynchronous operations are increasingly common.
Embracing Functional Interfaces in Everyday Development
Now that we understand the power of functional interfaces, it's important to leverage them effectively in our everyday development.
Use Cases for Functional Interfaces
- Event handling and listeners
- Iteration and filtering of collections
- Asynchronous and parallel programming
- Implementing simple callbacks and strategies
Best Practices
- Choose meaningful names for functional interfaces to clearly convey their intended purpose.
- Favor lambda expressions or method references over traditional anonymous classes for concise and readable code.
- Consider composing multiple functional interfaces to create more complex behaviors.
Potential Pitfalls
- Beware of accidentally adding additional abstract methods to a functional interface, as it would break the contract and lead to compile-time errors.
- Avoid excessive nesting of lambda expressions, as it can reduce code readability and maintainability.
Final Considerations
Functional interfaces, along with lambda expressions and method references, have revolutionized the way Java developers write code. They bring a taste of functional programming to Java, promoting readability, flexibility, and streamlined development. By understanding the power of functional interfaces and incorporating them into our development practices, we can elevate the quality and maintainability of our code.
Incorporating Java 8's functional interfaces into your development toolkit can significantly enhance the way you write code. To delve deeper into the world of functional programming in Java, consider exploring resources such as A Guide to Java Functional Interfaces and Official Java Documentation on Lambda Expressions.
Happy coding!