Overcoming CI/CD Pipeline Integration Challenges
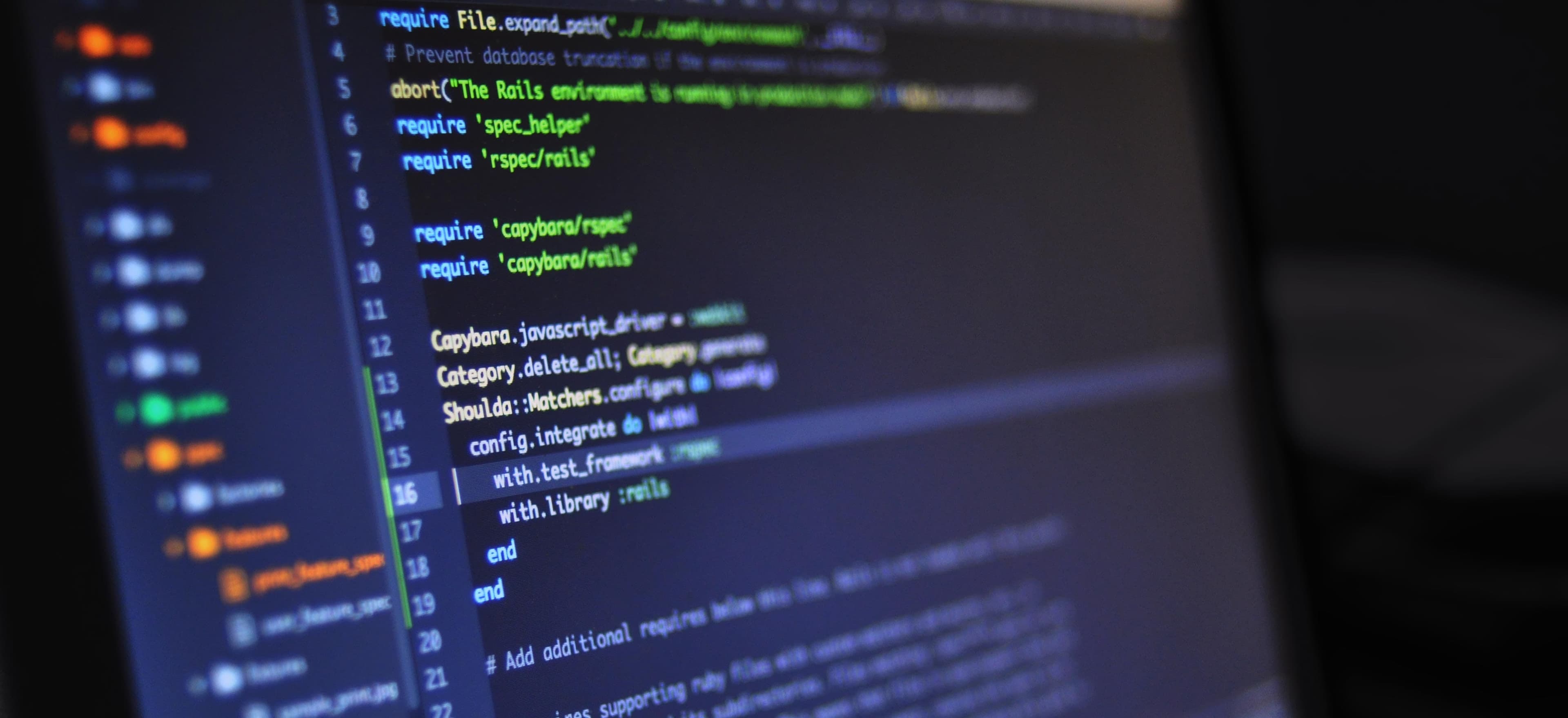
- Published on
Overcoming CI/CD Pipeline Integration Challenges
Continuous Integration/Continuous Deployment (CI/CD) pipelines have become an integral part of modern software development, allowing teams to efficiently build, test, and deploy their code. Despite the benefits, integrating CI/CD pipelines can present significant challenges for development teams. In this article, we'll explore common challenges and discuss strategies for overcoming them using Java, a popular programming language for enterprise applications.
Understanding CI/CD Pipeline Integration
Before delving into the challenges, it's essential to grasp the fundamentals of CI/CD pipeline integration. In a nutshell, CI/CD pipelines automate the process of merging code changes, running tests, and deploying applications. These pipelines ensure that code changes are rapidly and consistently delivered to production, thus enhancing the software development lifecycle.
Common CI/CD Pipeline Integration Challenges
Challenges when integrating CI/CD pipelines may include maintaining compatibility with various tools, orchestrating complex workflows, managing dependencies, ensuring proper testing, and handling deployment across different environments.
Java and CI/CD Integration
Java, with its strong ecosystem and compatibility with various tools, is frequently employed in enterprise CI/CD pipelines. Let's examine how Java can help tackle the challenges of CI/CD integration.
1. Tool Compatibility
Java's extensive ecosystem and wide support make it compatible with popular CI/CD tools such as Jenkins, Bamboo, and GitLab CI/CD. Leveraging Java's compatibility minimizes integration roadblocks, allowing teams to seamlessly incorporate their code into the pipeline.
// Example: Jenkinsfile using Java
pipeline {
agent any
stages {
stage('Build') {
steps {
// Compile Java code
sh 'javac MyApp.java'
}
}
stage('Test') {
steps {
// Run JUnit tests
sh 'java org.junit.runner.JUnitCore TestSuite'
}
}
stage('Deploy') {
steps {
// Deploy the application
sh 'java -jar MyApp.jar'
}
}
}
}
2. Orchestrating Complex Workflows
Java's support for various build automation tools, such as Apache Maven and Gradle, empowers teams to orchestrate complex build and deployment workflows. These tools simplify the management of dependencies, compilation, and packaging, enabling the creation of streamlined CI/CD pipelines.
// Example: Maven POM file defining build and dependency management
<project>
...
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
</dependency>
...
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
...
</plugins>
</build>
</project>
3. Ensuring Proper Testing
Java's well-established testing frameworks, such as JUnit and TestNG, enable comprehensive unit and integration testing within CI/CD pipelines. By leveraging these frameworks, teams can ensure the reliability and quality of their code before deployment.
// Example: JUnit test case for a Java class
public class MyTestClass {
@Test
public void testAddition() {
assertEquals(4, Calculator.add(2, 2));
}
}
4. Handling Deployment Across Environments
Java's platform independence and support for containerization technologies like Docker facilitate seamless application deployment across diverse environments. This capability streamlines the deployment process within CI/CD pipelines, ensuring consistent behavior across different platforms.
// Example: Dockerfile for packaging a Java application
FROM openjdk:8-jre
COPY ./target/myapp.jar /app/
CMD ["java", "-jar", "/app/myapp.jar"]
Wrapping Up
Integrating CI/CD pipelines with Java presents a robust solution to the challenges encountered in modern software development. By leveraging Java's compatibility, build automation tools, testing frameworks, and deployment capabilities, development teams can streamline their CI/CD processes and deliver high-quality software efficiently.
In conclusion, overcoming CI/CD pipeline integration challenges with Java involves embracing its rich ecosystem and leveraging its tools and capabilities to orchestrate seamless and reliable pipelines. With the right approach and utilization of Java's strengths, teams can elevate their CI/CD practices and propel their software delivery to new heights.
To learn more about Java and CI/CD integration, you can explore resources such as Java CI/CD Best Practices and Jenkins Pipeline Documentation.
Remember, with the right strategies and the power of Java, conquering CI/CD pipeline integration challenges is well within reach for software development teams.
Stay tuned for more insightful content on Java and CI/CD practices!