Updating your App for Android 2.3: Common Compatibility Issues
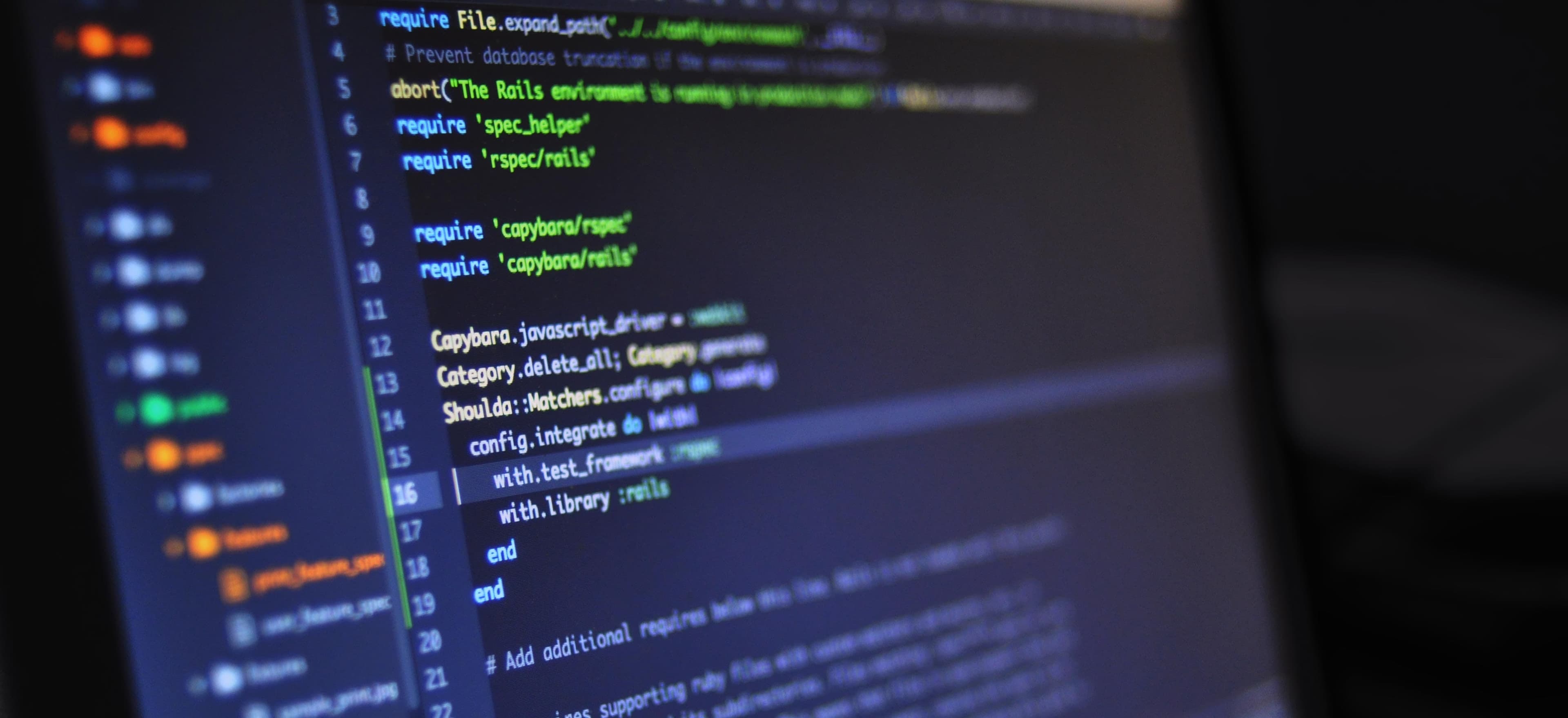
- Published on
Updating Your App for Android 2.3: Common Compatibility Issues
If you have an existing Android app and you want to ensure it remains compatible with older devices running Android 2.3 (Gingerbread), there are a few common compatibility issues you may encounter. In this post, we'll discuss these issues and provide solutions for updating your app to maintain compatibility with Android 2.3.
1. Handling Network Operations on the Main Thread
In Android 2.3, network operations on the main thread are allowed by default. However, this can lead to a poor user experience due to potential ANR (Application Not Responding) errors. To address this issue, it's essential to move network operations to a background thread using AsyncTask or other threading mechanisms.
// Example of using AsyncTask to perform network operations in the background
private class NetworkTask extends AsyncTask<Void, Void, String> {
protected String doInBackground(Void... params) {
// Perform network operation here
return result;
}
protected void onPostExecute(String result) {
// Update UI with the result
}
}
Why: Performing network operations on the main thread can cause the UI to become unresponsive, resulting in a poor user experience.
2. Supporting Different Screen Densities
Android 2.3 supports multiple screen densities, including ldpi, mdpi, hdpi, and xhdpi. When updating your app, ensure that your resources (images, layouts, etc.) are appropriately scaled for different screen densities. Use the proper resource qualifiers (e.g., drawable-ldpi, drawable-mdpi) to provide different assets for different screen densities.
res/
drawable-ldpi/
your_image.png
drawable-mdpi/
your_image.png
drawable-hdpi/
your_image.png
drawable-xhdpi/
your_image.png
Why: Without providing resources for different screen densities, your app may appear distorted or blurry on devices with non-standard densities.
3. Using Deprecated APIs
Android 2.3 introduced several new APIs while deprecating older ones. When updating your app, ensure that you replace deprecated APIs with their modern equivalents to maintain compatibility with Android 2.3 devices. Check the official Android documentation for deprecated APIs and their recommended replacements.
// Example of replacing deprecated API with modern equivalent
// Deprecated method
myTextView.setBackgroundDrawable(getResources().getDrawable(R.drawable.background));
// Modern equivalent
myTextView.setBackground(ContextCompat.getDrawable(this, R.drawable.background));
Why: Deprecated APIs may not function correctly or may be removed in future Android versions, causing compatibility issues on older devices.
4. Handling Permissions
Android 2.3 has a different permission model compared to newer versions. When updating your app, review the permissions required by your app and ensure that they align with the permissions requested by Android 2.3. Additionally, handle runtime permissions if your target SDK version is higher than 2.3 to maintain compatibility.
// Example of handling runtime permissions
if (ContextCompat.checkSelfPermission(this, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_CODE);
}
Why: Failure to handle permissions properly can lead to security vulnerabilities or app crashes on Android 2.3 devices.
5. Testing on Emulators and Real Devices
Finally, one of the crucial steps in ensuring compatibility with Android 2.3 is thorough testing on both emulators and real devices running Android 2.3. Emulators can be used to simulate different screen sizes and densities, while real devices provide accurate performance and behavior testing. Use Android Virtual Device Manager to create emulators for different API levels, including 2.3, and ensure that your app functions as expected.
Why: Testing on emulators and real devices helps identify and resolve compatibility issues specific to Android 2.3, ensuring a smooth user experience across a wide range of devices.
Wrapping Up
By addressing these common compatibility issues and following best practices for updating your app, you can ensure that it remains compatible with Android 2.3 while providing a seamless experience for users on older devices. Keep in mind that while maintaining compatibility with older versions is essential, it's also crucial to balance this with taking advantage of modern features and optimizations available in newer Android versions.
In summary, updating your app for Android 2.3 involves:
- Moving network operations to background threads to avoid ANR errors.
- Providing resources tailored for different screen densities to ensure proper display.
- Replacing deprecated APIs with modern equivalents for continued compatibility.
- Reviewing and handling permissions according to Android 2.3's permission model.
- Thoroughly testing on emulators and real devices to identify and resolve compatibility issues.
Following these guidelines will help you maintain a wider user base while providing a consistent experience across different Android versions.
Remember, keeping your app compatible with older Android versions not only expands your potential user base but also demonstrates your commitment to inclusivity and accessibility in the ever-growing Android ecosystem.
Now, armed with the knowledge of common compatibility issues with Android 2.3, you can confidently update and maintain your app, ensuring that it remains accessible to as many users as possible.