Troubleshooting RMI: Addressing Full GC Running Hourly
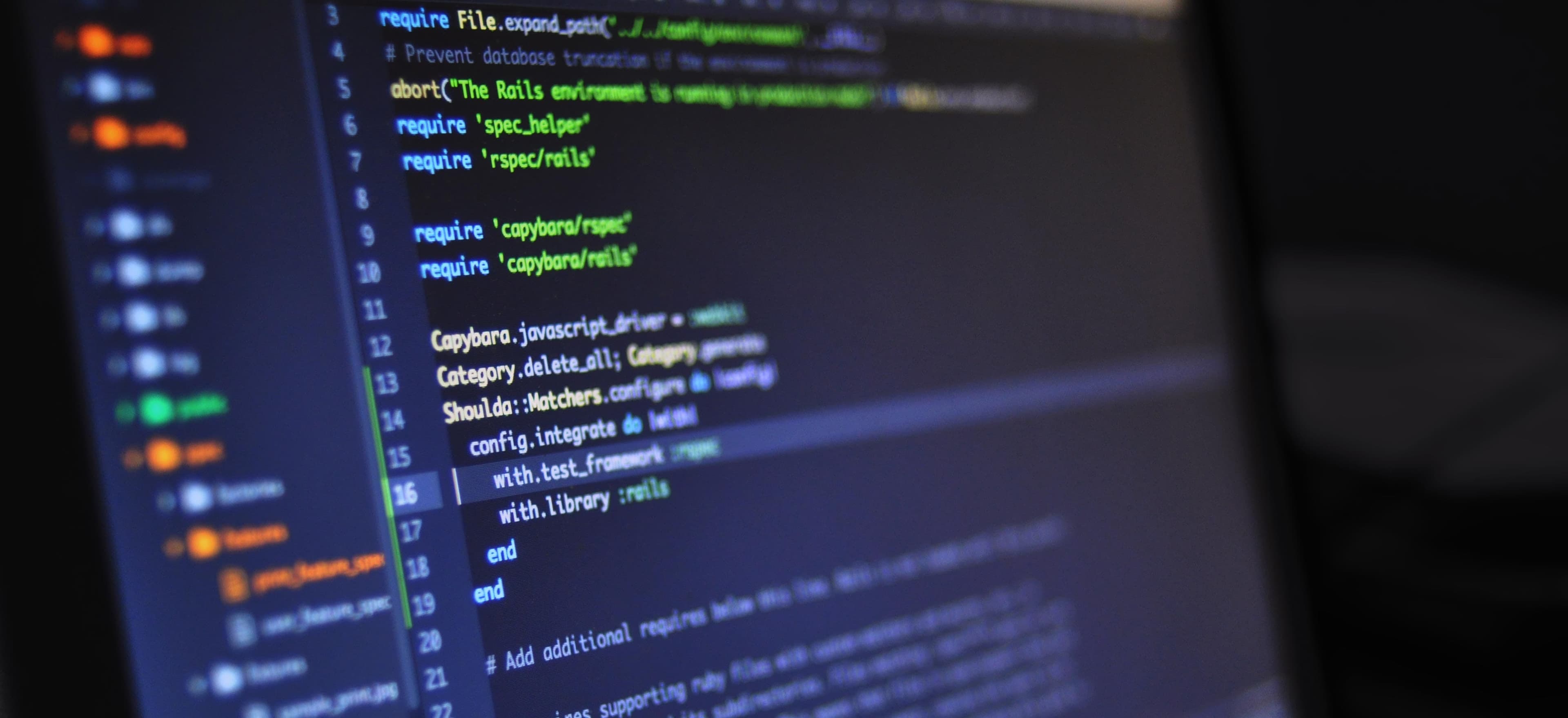
- Published on
Troubleshooting RMI: Addressing Full GC Running Hourly
In the world of Java programming, Remote Method Invocation (RMI) is a powerful tool for communication between different Java Virtual Machines (JVMs). However, like any technology, RMI comes with its own set of challenges. One common issue that arises in RMI applications is the occurrence of Full Garbage Collection (GC) running at unexpected frequencies, such as hourly. In this blog post, we will delve into the potential causes of this issue and explore strategies for troubleshooting and resolving it.
Understanding Full GC and Its Impact on RMI
Before we dive into troubleshooting, it's crucial to comprehend the implications of Full GC running frequently within an RMI application. Full GC is a process in which the JVM reclaims memory by scanning the entire heap and identifying unreferenced objects. When Full GC occurs frequently, it can lead to significant pauses in the application, causing performance degradation and potential service interruptions in RMI-based systems.
Identifying the Root Cause
When faced with the challenge of Full GC running hourly in an RMI application, the first step is to identify the root cause. There are several potential factors that could contribute to this issue, including memory leaks, inefficient object creation, and improper JVM tuning.
Memory Leaks
Memory leaks occur when objects are unintentionally retained in memory, ultimately leading to excessive memory consumption. In an RMI application, memory leaks can be caused by improperly implemented remote objects that are not released after use, or by references held in caches that are never released. These leaks can prompt frequent Full GC cycles to reclaim memory, resulting in hourly occurrences.
Inefficient Object Creation
Inefficient object creation, such as excessive and unnecessary object instantiation, can contribute to a rapid increase in memory usage. This, in turn, can trigger more frequent Full GC runs to manage the growing heap space. Analyzing object creation patterns and optimizing the code for efficient memory usage can help address this issue.
Improper JVM Tuning
Inadequate JVM tuning, including misconfigured heap sizes, garbage collection settings, and other JVM parameters, can also lead to frequent Full GC cycles. Properly tuning the JVM based on the specific requirements of the RMI application can significantly mitigate this issue.
Troubleshooting Strategies
Once the potential causes have been identified, the next step is to implement targeted troubleshooting strategies to address the issue of Full GC running hourly in the RMI application. Let's explore some effective approaches to troubleshooting this problem.
Use of Profiling Tools
Employing profiling tools such as VisualVM, YourKit, or Java Mission Control can provide valuable insights into memory usage, object creation patterns, and garbage collection behavior within the RMI application. These tools enable developers to identify memory leaks, inefficient object creation, and suboptimal JVM configurations.
Example of profiling tool usage in Java:
// Using VisualVM to analyze memory usage
public class MemoryAnalyzer {
public static void main(String[] args) {
// Code for initializing the RMI application
// ...
// Enable JMX for the RMI application
// ...
// Connect VisualVM to the running RMI application for memory analysis
// ...
}
}
Why: Profiling tools offer a visual representation of memory usage and performance metrics, allowing developers to pinpoint potential memory-related issues in the RMI application.
Memory Dump Analysis
Taking memory dumps at regular intervals and analyzing them using tools like Eclipse Memory Analyzer can reveal detailed information about memory allocation, object retention, and potential memory leaks within the RMI application.
// Triggering a memory dump programmatically
public class MemoryDumpTrigger {
public static void main(String[] args) {
// Code for initializing the RMI application
// ...
// Trigger a memory dump at a specific interval or upon encountering Full GC
// ...
}
}
Why: Memory dump analysis provides in-depth visibility into memory usage and object retention, aiding in the identification of potential memory leak sources.
Code Review and Optimization
Conducting a comprehensive review of the RMI application's codebase to identify inefficient object creation, unnecessary object retention, and improper resource management is essential. Optimizing the code to minimize memory footprint and improve object reuse can significantly reduce the frequency of Full GC cycles.
Example of code optimization in RMI application:
// Refactoring remote object instantiation for efficiency
public class RemoteObjectFactory {
public RemoteObject createRemoteObject() {
// Existing code for creating remote object
// ...
// Refactor to reuse existing objects or employ object pooling
// ...
}
}
Why: Code review and optimization aim to address inefficient object creation and management practices that contribute to frequent Full GC invocations.
Implementing Solutions
Armed with insights from troubleshooting strategies, it's time to implement targeted solutions to alleviate the issue of Full GC running hourly in the RMI application. Based on the identified root causes, the following actions can be taken:
Memory Leak Resolution
Identify and rectify memory leaks within the RMI application by ensuring proper release of resources, implementing effective caching strategies with proper eviction policies, and employing weak references where applicable.
Object Creation Optimization
Optimize object creation by reusing objects where possible, employing object pooling for frequently used resources, and minimizing the instantiation of unnecessary objects within the RMI application.
JVM Tuning
Fine-tune the JVM parameters, including heap sizes, garbage collection algorithms, and memory allocation settings, to align with the memory requirements and usage patterns of the RMI application.
A Final Look
Troubleshooting and resolving the issue of Full GC running hourly in an RMI application involves a systematic approach that encompasses identification of root causes, implementation of targeted troubleshooting strategies, and application of specific solutions tailored to the identified issues. By leveraging profiling tools, memory dump analysis, code optimization, and proper JVM tuning, developers can effectively address the challenges of excessive Full GC cycles and ensure the optimal performance of RMI-based systems.
Remember, proactive monitoring and periodic reassessment of memory and garbage collection behavior are essential to maintain the long-term stability and efficiency of RMI applications.
In conclusion, addressing Full GC running hourly in RMI applications demands meticulous analysis, strategic intervention, and continuous vigilance to uphold performance excellence in Java-based distributed systems.
For further reading on RMI and Java memory management, consider exploring Oracle's official documentation and insightful articles on memory optimization techniques in Java.
Stay tuned for more Java-centric insights and best practices on our blog!