Managing Locks in the Java Virtual Machine
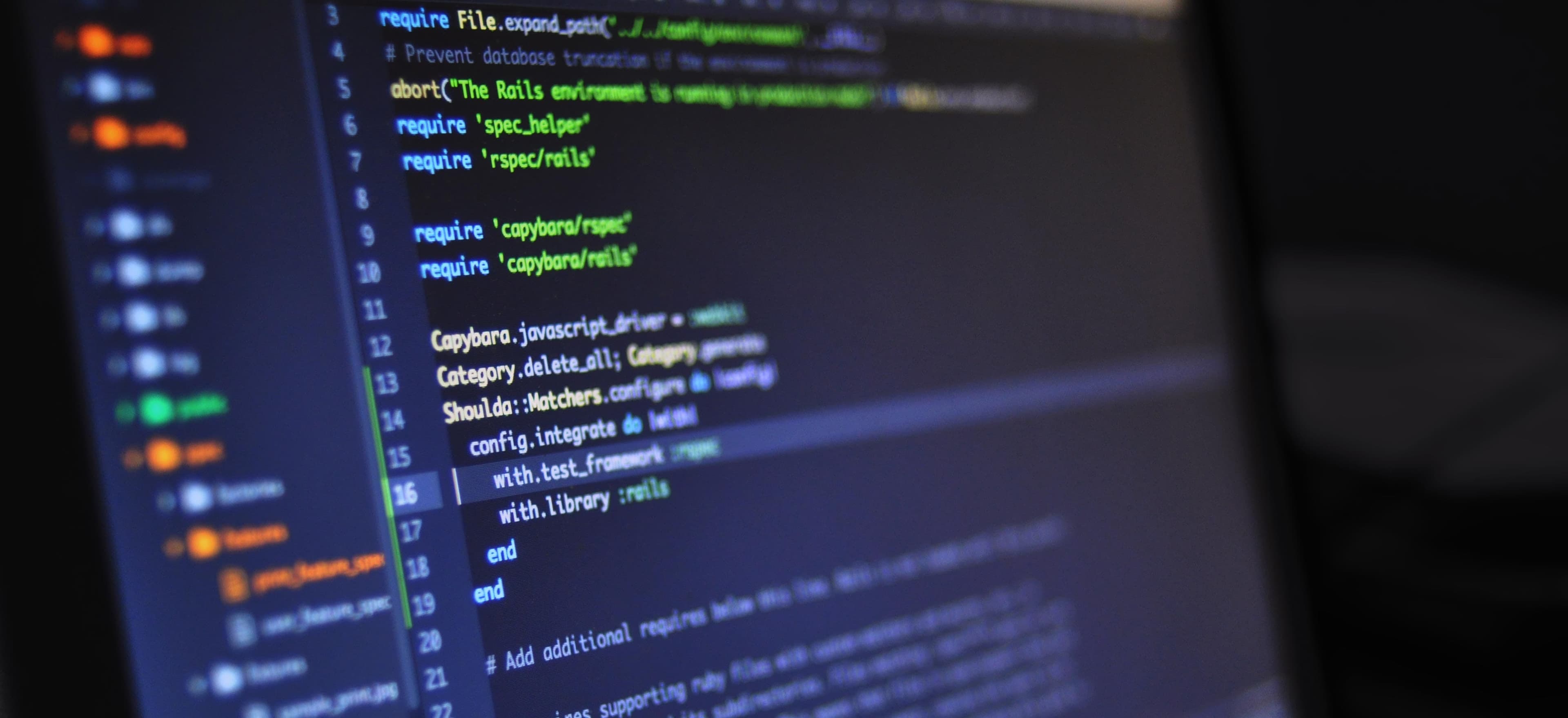
- Published on
In the world of multithreading and concurrent programming, understanding how locks work in the Java Virtual Machine (JVM) is crucial. Locks play a vital role in controlling access to shared resources, preventing race conditions, and ensuring thread safety. In this blog post, we will delve into the intricacies of managing locks in Java, exploring different types of locks, their usage, and best practices for effective lock management.
Starting Off to Locking in Java
In Java, locks are used to control access to critical sections of code by allowing only one thread to execute a specific block of code at a time. This helps in maintaining data integrity and preventing conflicts when multiple threads are accessing shared resources concurrently.
Using synchronized
Keyword for Intrinsic Locks
The most common way to work with locks in Java is by using the synchronized
keyword to create synchronized blocks or methods. When a thread enters a synchronized block, it acquires the intrinsic lock associated with the object's monitor, allowing it to execute the synchronized code. Once the thread exits the synchronized block, it releases the lock, allowing other threads to enter the synchronized section.
public class SynchronizedExample {
private final Object lock = new Object();
public void performSynchronizedTask() {
synchronized (lock) {
// Synchronized code block
// Critical section of code
}
}
}
The synchronized
keyword provides a simple and effective way to manage locks and ensure thread safety. However, it has some limitations, such as lack of flexibility and reusability, which led to the introduction of explicit lock implementations in Java.
Starting Off to Explicit Lock Implementations
Java provides explicit lock implementations through the java.util.concurrent.locks
package, offering more flexibility and control over lock management compared to intrinsic locks. Two of the most commonly used explicit lock implementations are ReentrantLock
and ReadWriteLock
.
Using ReentrantLock
for Explicit Locking
The ReentrantLock
class is a versatile lock implementation that provides features such as lock acquisition with timeouts, fairness policies, and the ability to interrupt lock acquisition. It also supports finer-grained locking and can be used in conjunction with condition variables for advanced thread coordination.
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
public void performLockedTask() {
lock.lock();
try {
// Critical section of code
} finally {
lock.unlock();
}
}
}
Using ReentrantLock
allows for more advanced lock management and can be particularly useful when dealing with complex synchronization requirements.
Using ReadWriteLock
for Optimized Read/Write Access
In scenarios where read operations outnumber write operations, using a ReadWriteLock
can offer better performance compared to exclusive locks. The ReadWriteLock
interface provides separate locks for read and write operations, allowing multiple threads to read simultaneously while ensuring exclusive write access.
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private final ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
private final ReentrantReadWriteLock.ReadLock readLock = readWriteLock.readLock();
private final ReentrantReadWriteLock.WriteLock writeLock = readWriteLock.writeLock();
public void performReadTask() {
readLock.lock();
try {
// Read operation
} finally {
readLock.unlock();
}
}
public void performWriteTask() {
writeLock.lock();
try {
// Write operation
} finally {
writeLock.unlock();
}
}
}
By using ReadWriteLock
, you can optimize concurrency for scenarios where reads are more frequent than writes.
Best Practices for Lock Management in Java
Effective lock management is essential for building robust, concurrent applications in Java. Here are some best practices to consider when working with locks:
1. Use Try-With-Resources for Lock Acquisition
When working with explicit locks such as ReentrantLock
, it is recommended to use the try-with-resources statement to ensure proper lock management and automatic release of the lock, even in the event of an exception.
public void performLockedTask() {
try (var ignored = lock.newLock()) {
// Critical section of code
}
}
Using try-with-resources ensures that the lock is always released, even if an exception occurs within the critical section.
2. Avoid Holding Locks During I/O and Lengthy Operations
To prevent blocking other threads and improve overall concurrency, avoid holding locks during I/O operations, network calls, or lengthy computations. Instead, consider releasing the lock before initiating such operations and re-acquiring it afterward if necessary.
3. Use Fairness Policies When Appropriate
In scenarios where multiple threads contend for a lock, consider using fairness policies to ensure that threads acquire the lock in the order they requested it. This can help prevent thread starvation and promote equitable access to shared resources.
My Closing Thoughts on the Matter
In conclusion, managing locks in the Java Virtual Machine is a critical aspect of building scalable and thread-safe applications. By understanding the intricacies of lock management, leveraging explicit lock implementations, and following best practices, developers can effectively control access to shared resources, prevent race conditions, and ensure thread safety in concurrent Java applications.
In this post, we explored the usage of synchronized
keyword for intrinsic locks, introduced explicit lock implementations such as ReentrantLock
and ReadWriteLock
, and discussed best practices for effective lock management. By applying these principles and techniques, developers can harness the power of locks to build efficient and resilient concurrent Java applications.
For further reading on concurrent programming and lock management in Java, check out Oracle's official documentation and Java Concurrency in Practice by Brian Goetz et al., a highly recommended book for mastering concurrent programming in Java.