Dynamic Property Configuration in Spring Cloud
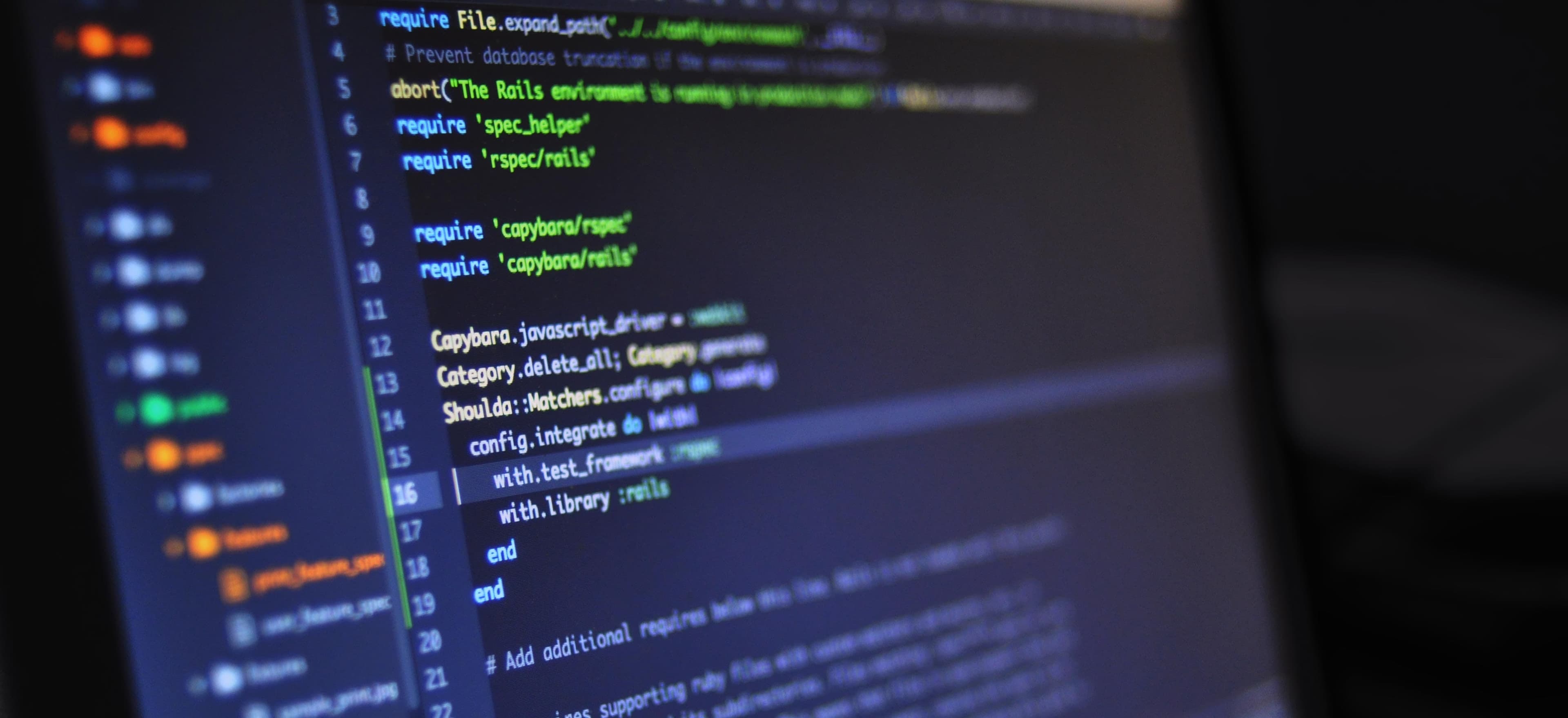
- Published on
Enhancing Flexibility: Dynamic Property Configuration in Spring Cloud
In a microservices architecture, managing configuration properties across multiple services can be quite challenging. Spring Cloud provides a fantastic solution for this through its dynamic property configuration feature. This feature allows us to update configurations at runtime, without restarting services, providing flexibility and agility to the system.
Understanding Dynamic Property Configuration
Dynamic property configuration in Spring Cloud relies on a centralized configuration server, such as Spring Cloud Config, where services can fetch their configuration properties. These properties can be stored in a version-controlled repository like Git, allowing for easy management and tracking of changes.
Implementing Dynamic Property Configuration
To demonstrate this in action, let's create a simple Spring Boot application and configure it to fetch properties dynamically from a Spring Cloud Config server.
Setting Up the Config Server
First, we need to set up the Spring Cloud Config server. We can achieve this by creating a new Spring Boot project and adding the spring-cloud-config-server
dependency.
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
By annotating the main application class with @EnableConfigServer
, we enable the configuration server functionality. We can then configure the server to fetch configuration properties from a Git repository by adding the following properties to the application.properties
file:
spring.cloud.config.server.git.uri=https://github.com/your-org/config-repo
spring.cloud.config.server.git.username=your-username
spring.cloud.config.server.git.password=your-password
Creating a Configurable Service
Next, let's create a simple Spring Boot service that fetches its configuration properties from the Spring Cloud Config server.
@SpringBootApplication
@RefreshScope
public class ConfigurableServiceApplication {
@Autowired
private ConfigurableEnvironment environment;
public static void main(String[] args) {
SpringApplication.run(ConfigurableServiceApplication.class, args);
}
@PostConstruct
public void printConfigProperties() {
System.out.println(environment.getProperty("example.property"));
}
}
In this example, the @RefreshScope
annotation ensures that the configuration properties are refreshed whenever there is a change in the Config server. The @Autowired
ConfigurableEnvironment
allows us to access the properties.
Generating and Fetching Dynamic Configurations
To test the dynamic property configuration, we can make changes to the configuration properties in the Git repository and then trigger a refresh in the configurable service by sending a POST request to the /actuator/refresh
endpoint.
With this setup, our services can dynamically fetch configuration properties from the centralized Config server, allowing for seamless configuration updates without requiring service restarts.
Benefits of Dynamic Property Configuration
Enhanced Flexibility
Dynamic property configuration enhances the flexibility of microservices by allowing them to adapt to configuration changes in real-time. This is particularly useful in scenarios where quick adjustments are needed without interrupting service availability.
Centralized Management
Centralizing configuration properties in a Config server makes it easier to manage and update them across multiple services. It also provides a single source of truth for configurations, reducing the likelihood of inconsistencies.
Version Control
By storing configuration properties in a version-controlled repository like Git, we gain the ability to track changes, revert to previous versions, and maintain an audit trail of configuration modifications.
Lessons Learned
Dynamic property configuration in Spring Cloud offers a powerful mechanism for managing configuration properties in a microservices environment. By leveraging a centralized Config server and version-controlled repository, services can dynamically fetch configurations without the need for service restarts, leading to enhanced flexibility and simplified configuration management.
With the ability to make quick changes and updates without disrupting services, dynamic property configuration is a valuable tool for maintaining a flexible and responsive microservices architecture.
Incorporating dynamic property configuration into your Spring Cloud-based microservices can greatly enhance the agility and configurability of your system, making it a valuable addition to your architecture.
By embracing dynamic property configuration in Spring Cloud, you can ensure that your microservices remain adaptable, resilient, and easy to manage in the face of evolving configuration requirements.