Optimizing GWT Development Environment for Productivity
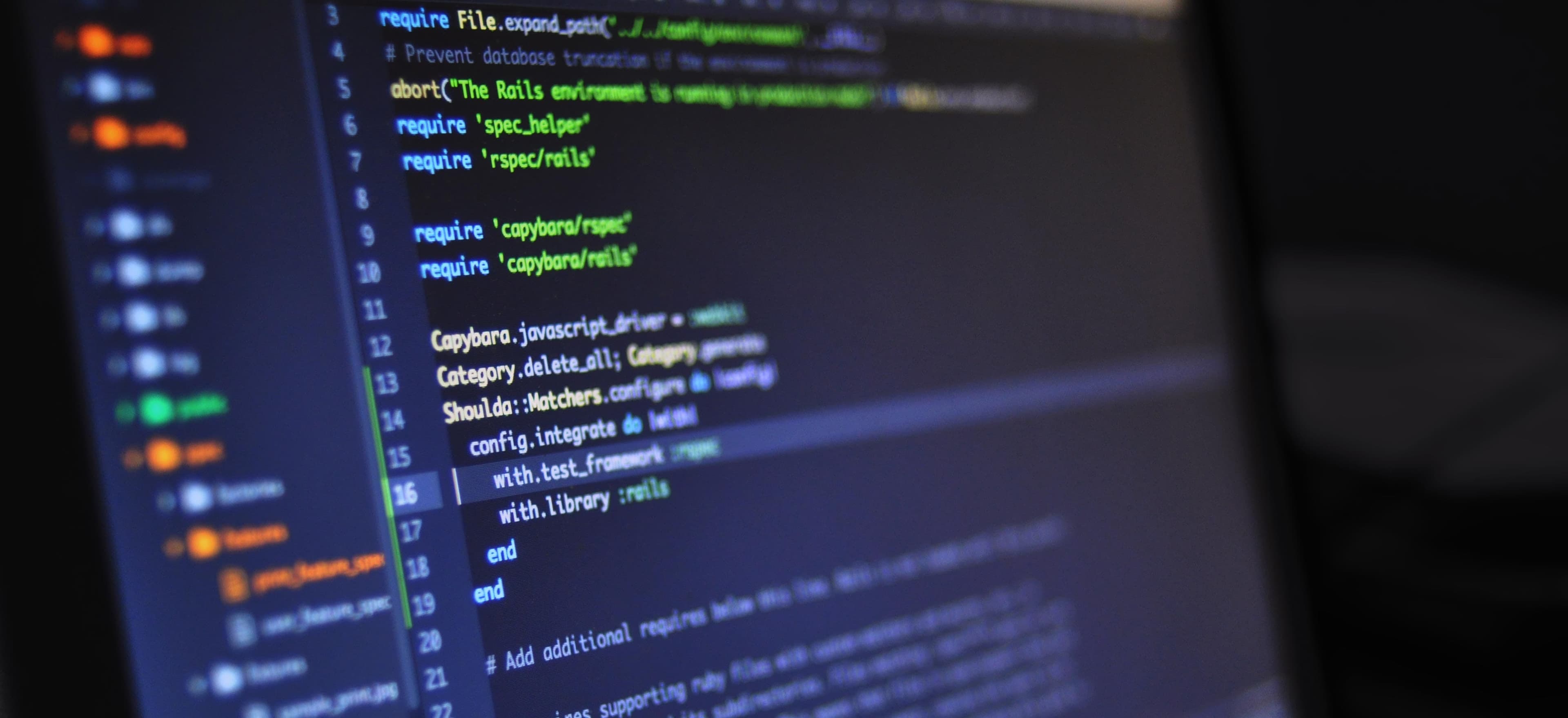
- Published on
Optimizing GWT Development Environment for Productivity
When it comes to developing web applications, an efficient and well-optimized development environment is crucial for boosting productivity. In the Java ecosystem, Google Web Toolkit (GWT) remains a powerful tool for building high-performance, browser-based applications. In this article, we will explore strategies for optimizing the GWT development environment to enhance productivity, streamline workflows, and deliver outstanding web applications.
Setting Up the Development Environment
To begin, ensure that you have the latest version of Java Development Kit (JDK) installed on your system. Additionally, you'll need Apache Maven for managing project dependencies and build configurations. IDEs such as IntelliJ IDEA or Eclipse with the GWT plugin are excellent choices for GWT development due to their robust features and seamless integration with GWT.
Utilizing Super Dev Mode
Super Dev Mode is a powerful feature of GWT that enables faster compilation and enhanced debugging capabilities. By using Super Dev Mode, developers can make changes to the client-side code and see the results without triggering a full recompilation. This significantly reduces iteration times during development and leads to a more fluid debugging experience.
To enable Super Dev Mode, simply add the -XsuperDevMode
flag to the GWT development mode configuration in your build tools or IDE. This will activate the Super Dev Mode support, allowing for quicker code changes and updates without the need for time-consuming recompilation.
// Example Maven configuration for Super Dev Mode
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>gwt-maven-plugin</artifactId>
<version>2.8.2</version>
<configuration>
<extraJvmArgs>-XsuperDevMode</extraJvmArgs>
</configuration>
</plugin>
The use of Super Dev Mode can significantly improve development efficiency and shorten feedback loops, resulting in a more productive development environment.
Embracing Code Splitting for Performance
Code splitting is a vital technique for optimizing the loading performance of GWT applications. By splitting the application's code into smaller, more manageable parts, we can reduce initial loading times and improve the overall user experience. GWT provides a built-in mechanism for code splitting, allowing developers to define entry points and split points within their application.
// Example code splitting in GWT
@SplitPoint
public class MySplitPointPresenter {
// ...
}
By strategically employing code splitting, developers can ensure that only the necessary code is loaded initially, with additional modules fetched on-demand. This approach minimizes the initial payload, leading to faster load times and improved application responsiveness.
Leveraging Deferred Binding
Deferred binding is a core feature of GWT that empowers developers to create optimized, browser-specific code without repeating logic or compromising maintainability. With deferred binding, developers can tailor their application to specific browsers, device types, or other conditions, resulting in a more efficient and tailored user experience.
// Example deferred binding in GWT
public class MyFactory {
public static MyService create() {
if (GWT.create(MyService.class) instanceof MyServiceImplementationChrome) {
return new MyServiceImplementationChrome();
} else {
return new MyServiceImplementationGeneric();
}
}
}
By utilizing deferred binding, developers can optimize their applications for various environments while maintaining a clean and concise codebase. This not only contributes to improved performance but also enhances the overall user satisfaction.
Incorporating Client-Side Caching
Client-side caching is a potent strategy for reducing server load and improving the responsiveness of GWT applications. By caching static resources such as JavaScript, CSS, and image files on the client side, we can minimize the need for repeated downloads and accelerate the overall loading speed of the application.
When implementing client-side caching, it's essential to ensure proper cache control headers are set on the server side, allowing browsers to store resources locally and retrieve them efficiently. Additionally, utilizing versioned file names for static resources can prevent caching issues when updates are deployed.
// Example cache control configuration in server responses
response.setHeader("Cache-Control", "max-age=3600, must-revalidate");
By incorporating client-side caching, developers can create GWT applications that deliver a snappy, responsive user experience while reducing server overhead and bandwidth consumption.
Integrating Automated Testing
Automated testing plays a pivotal role in maintaining code quality, identifying issues early in the development cycle, and ensuring robustness in GWT applications. By integrating testing frameworks such as JUnit and Selenium with GWT, developers can automate the validation of application functionality, UI interactions, and performance benchmarks.
Additionally, the use of continuous integration tools like Jenkins or Travis CI can further streamline the testing process, providing rapid feedback on code changes and reducing the likelihood of regressions.
Lessons Learned
Optimizing the GWT development environment can significantly enhance productivity, code quality, and overall user satisfaction. By leveraging features such as Super Dev Mode, code splitting, deferred binding, client-side caching, and automated testing, developers can create high-performance web applications with remarkable efficiency.
To stay updated with the latest advancements in GWT and best practices for Java web development, it's essential to actively engage with the thriving community and explore reputable resources such as GWT Project and GWT Stack Overflow.
By honing your GWT development environment and embracing best practices, you can embark on a journey to deliver compelling, responsive web applications that resonate with users and stand out in the digital landscape.
Checkout our other articles