Containerizing a Java application: Handling dependencies and configurations
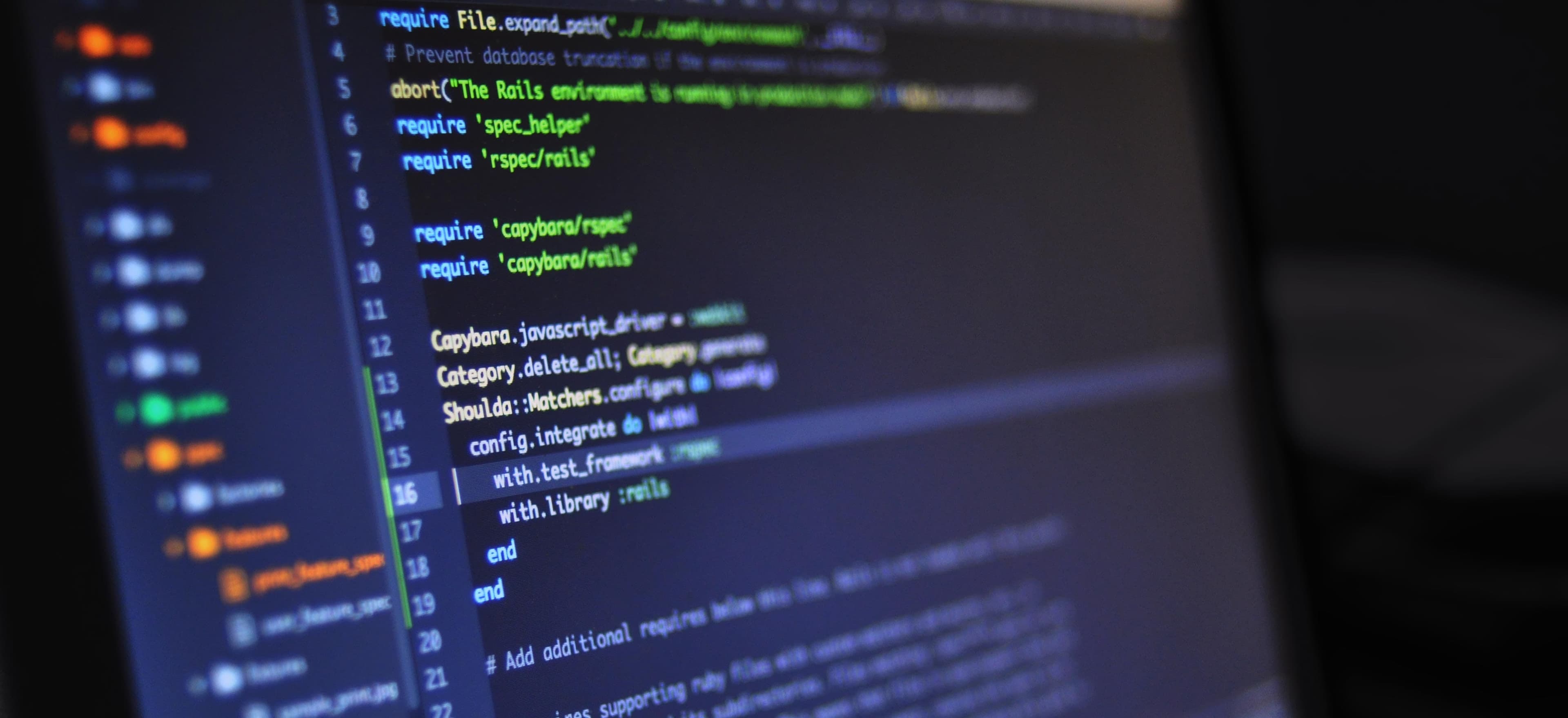
- Published on
Containerizing a Java Application
In today's software development landscape, containerization has become a standard practice for deploying and managing applications. With the rise of technologies like Docker and Kubernetes, containerization provides a consistent environment for applications to run across different infrastructure. This article will focus on containerizing a Java application, specifically addressing the handling of dependencies and configurations.
Why Containerize a Java Application?
Containerizing a Java application offers several benefits, including:
- Portability: Containers encapsulate the application and its dependencies, making it runnable across different environments without compatibility issues.
- Consistency: Containers ensure that the application runs the same everywhere, mitigating the "it works on my machine" problem.
- Scalability: Containers can be easily scaled up or down based on demand, making them ideal for microservices architecture.
Choosing a Base Image
When containerizing a Java application, the choice of base image is crucial. Docker offers official OpenJDK images, which serve as a solid base for Java applications. It's important to select the appropriate version of the OpenJDK image based on the Java version your application requires. For example, if your Java application is compatible with Java 11, you would choose the openjdk:11
as your base image.
# Dockerfile
FROM openjdk:11
COPY ./target/my-java-app.jar /app/my-java-app.jar
CMD ["java", "-jar", "/app/my-java-app.jar"]
In the above Dockerfile, we're using the openjdk:11
as our base image, copying the Java application JAR file into the container, and then specifying the command to run the application. This simple Dockerfile sets up the foundation for containerizing a Java application.
Managing Dependencies with Maven or Gradle
Java applications often rely on dependency management tools such as Maven or Gradle. When containerizing a Java application, it's essential to consider how these dependencies will be handled within the container.
Maven
If your Java application uses Maven for dependency management, you can leverage the maven:3-jdk-11
Docker image, which comes preconfigured with Maven and the specified JDK.
# Dockerfile for Maven-based Java application
FROM maven:3-jdk-11 AS builder
WORKDIR /app
COPY pom.xml .
RUN mvn dependency:go-offline
COPY src/ /app/src/
RUN mvn package
FROM openjdk:11
COPY --from=builder /app/target/my-java-app.jar /app/my-java-app.jar
CMD ["java", "-jar", "/app/my-java-app.jar"]
In this Dockerfile, a multi-stage build is utilized. In the first stage, the Maven image is used to resolve and download the project dependencies. Then, in the second stage, the compiled application JAR is copied into the final image based on the smaller openjdk:11
image.
Gradle
For projects using Gradle, the gradle:6.5.1-jdk11
Docker image provides a pre-installed environment with the specified version of Gradle and the JDK.
# Dockerfile for Gradle-based Java application
FROM gradle:6.5.1-jdk11 AS builder
WORKDIR /app
COPY build.gradle settings.gradle /app/
COPY src /app/src/
RUN gradle build
FROM openjdk:11
COPY --from=builder /app/build/libs/my-java-app.jar /app/my-java-app.jar
CMD ["java", "-jar", "/app/my-java-app.jar"]
The above Dockerfile follows a similar multi-stage approach as the Maven example, ensuring that only the necessary artifacts are included in the final image.
Managing Application Configurations
When containerizing a Java application, it's important to separate the application code from its configuration. This allows for greater flexibility and maintainability. Environment-specific configurations can be passed to the containerized application through environment variables or configuration files.
Environment Variables
Environment variables provide a way to inject configuration values into the application at runtime. Within the Java application, these environment variables can be accessed using System.getenv("VARIABLE_NAME")
or through frameworks like Spring Boot's @Value
annotation.
# Dockerfile with environment variables for configuration
FROM openjdk:11
COPY ./target/my-java-app.jar /app/my-java-app.jar
ENV DATABASE_URL=jdbc:mysql://localhost:3306/mydb
ENV DATABASE_USERNAME=admin
ENV DATABASE_PASSWORD=secretpassword
CMD ["java", "-jar", "/app/my-java-app.jar"]
By defining environment variables in the Dockerfile, the configuration is decoupled from the application code, allowing for easy adjustment based on different deployment environments.
Configuration Files
Another approach to managing application configurations is by utilizing external configuration files. These files can be included within the Docker image or mounted as volumes when running the container.
# Dockerfile with configuration file
FROM openjdk:11
COPY ./target/my-java-app.jar /app/my-java-app.jar
COPY ./config/application.properties /app/application.properties
CMD ["java", "-jar", "/app/my-java-app.jar", "--spring.config.location=/app/application.properties"]
In this example, the application.properties file is included in the Docker image. When running the container, the --spring.config.location
option specifies the location of the external configuration file.
Bringing It All Together
Containerizing a Java application involves thoughtful consideration of managing dependencies and configurations within the containerized environment. Choosing the right base image, handling dependencies with Maven or Gradle, and managing application configurations are essential aspects to ensure a smooth and efficient containerized deployment.
By following best practices and leveraging the tools and techniques discussed in this article, developers can effectively containerize their Java applications, paving the way for streamlined deployment and scalability in modern cloud-native environments.
In this post, we've explored the key concepts and practical approaches to containerize a Java application, with a focus on handling dependencies and configurations. Embracing containerization not only ensures the consistency and portability of Java applications but also sets the stage for leveraging orchestration platforms like Kubernetes for managing containerized workloads.
To delve deeper into Java containerization and its ecosystem, consider exploring Kubernetes for orchestration and Docker Hub for finding and sharing container images. Happy containerizing!