Improving Test Clarity with Named Parameters
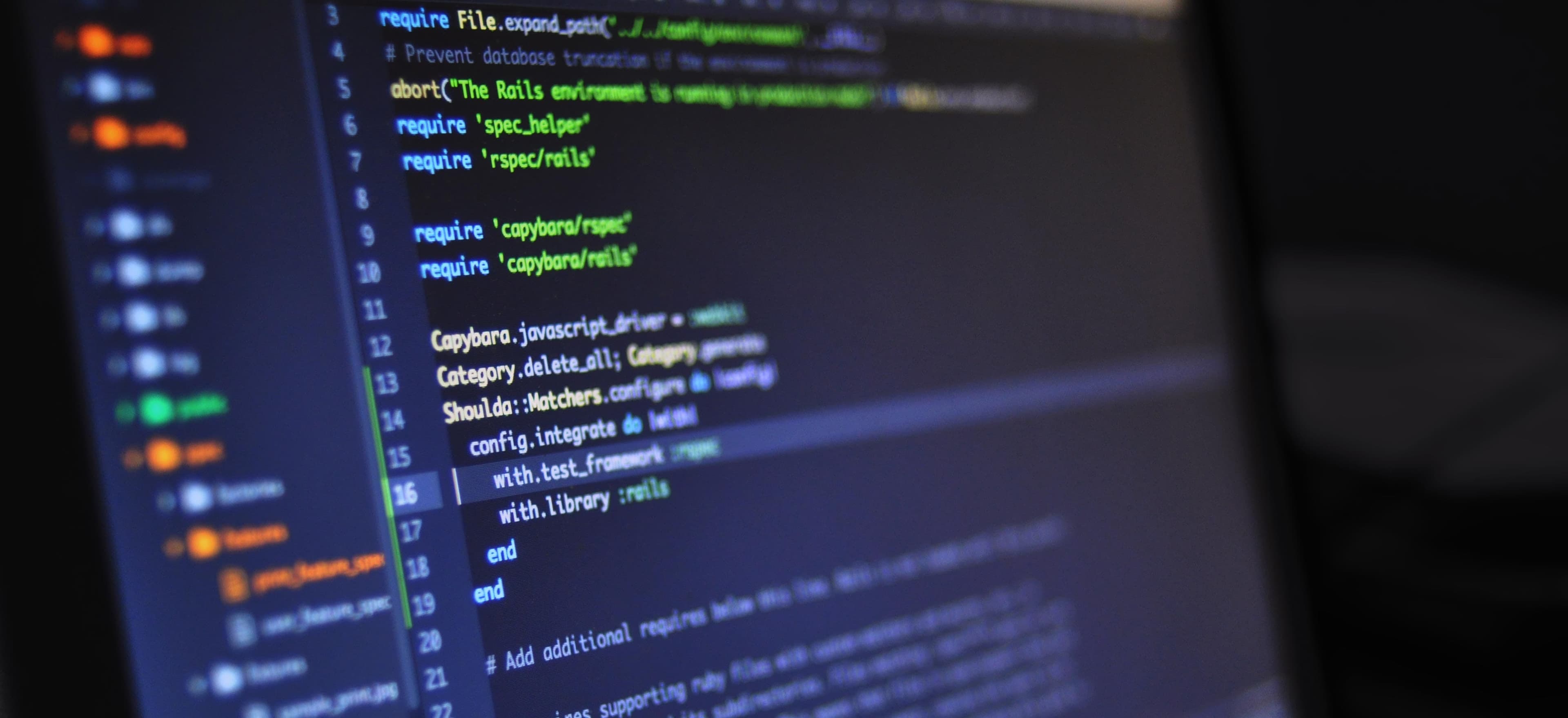
- Published on
Improving Test Clarity with Named Parameters in Java
When writing unit tests in Java, it's essential to ensure the clarity and readability of the test cases. One way to achieve this is by using named parameters, which can greatly enhance the understanding of the test logic. In this article, we'll explore how named parameters can improve the clarity of test cases in Java and provide examples to illustrate their benefits.
The Importance of Clarity in Unit Tests
Clarity in unit tests is crucial for several reasons. Firstly, clear and readable tests make it easier for developers to understand the intent of the test cases. This is especially important when tests need to be maintained or debugged by someone other than the original author. Additionally, clear tests facilitate communication among team members and help ensure that the tests accurately reflect the expected behavior of the code under test.
The Challenge of Positional Parameters
In traditional unit testing, it's common to see tests that rely on positional parameters when invoking methods. For example, consider the following test case:
@Test
public void calculateTotalPrice() {
// Setup
double unitPrice = 10.0;
int quantity = 5;
// Exercise
double totalPrice = calculateTotalPrice(unitPrice, quantity);
// Verify
assertEquals(50.0, totalPrice);
}
While this test is simple, it relies on positional parameters when invoking the calculateTotalPrice
method. If another developer or the original author revisits this test in the future, they might have to spend time deciphering the meaning of calculateTotalPrice(unitPrice, quantity)
. This becomes even more challenging as the number of parameters increases or when the method signature changes.
Introducing Named Parameters
Named parameters provide a solution to the challenge of positional parameters by explicitly specifying the parameter names when invoking methods. In Java, named parameters are not a language feature, but they can be achieved through creative coding techniques.
Let's revisit the previous example with named parameters:
@Test
public void calculateTotalPrice() {
// Setup
double unitPrice = 10.0;
int quantity = 5;
// Exercise
double totalPrice = calculateTotalPrice(unitPrice: unitPrice, quantity: quantity);
// Verify
assertEquals(50.0, totalPrice);
}
In this example, we've used named parameters unitPrice
and quantity
when invoking the calculateTotalPrice
method. This makes the intent of the test case much clearer, as anyone reading the test can easily understand the purpose of each parameter.
Achieving Named Parameters in Java
In Java, achieving named parameters requires a bit of creativity since the language does not support them natively. One way to simulate named parameters is by utilizing the builder pattern. Let's take a look at how we can refactor the calculateTotalPrice
method to accept parameters using the builder pattern:
public class PriceCalculation {
private double unitPrice;
private int quantity;
public PriceCalculation withUnitPrice(double unitPrice) {
this.unitPrice = unitPrice;
return this;
}
public PriceCalculation withQuantity(int quantity) {
this.quantity = quantity;
return this;
}
public double calculateTotalPrice() {
return unitPrice * quantity;
}
}
With this refactoring, the PriceCalculation
class now provides fluent setter methods (withUnitPrice
and withQuantity
) that return the instance of the class itself, allowing method chaining. Here's how the test case looks after the refactoring:
@Test
public void calculateTotalPrice() {
// Setup
PriceCalculation calculator = new PriceCalculation()
.withUnitPrice(10.0)
.withQuantity(5);
// Exercise
double totalPrice = calculator.calculateTotalPrice();
// Verify
assertEquals(50.0, totalPrice);
}
By refactoring the method to accept parameters using the builder pattern, we've effectively achieved named parameters in Java. This approach not only improves the clarity of the test cases but also makes the code more maintainable and adaptable to changes in the method signature.
Benefits of Named Parameters
Using named parameters in Java tests offers several benefits:
-
Improved Readability: Named parameters make the intent of the test cases explicit, improving the readability and understanding of the test logic.
-
Flexibility: When using named parameters, the order of the parameters becomes irrelevant, allowing for more flexible test case design.
-
Adaptability: Named parameters make the tests more resistant to changes in the method signature. If the order or number of parameters changes, the test cases remain unaffected.
-
Self-Documenting Code: Test cases using named parameters act as self-documenting examples of how to use the tested code.
By leveraging named parameters, developers can write unit tests that are easier to understand, maintain, and adapt to changes, contributing to overall code quality and development efficiency.
My Closing Thoughts on the Matter
Named parameters offer a valuable technique for improving the clarity and readability of unit tests in Java. By making the intent of the test cases explicit and decoupling the tests from the method signature, named parameters enhance the maintainability and adaptability of the test code. Although Java does not natively support named parameters, the builder pattern provides a practical way to achieve this functionality, resulting in more expressive and self-documenting tests.
Incorporating named parameters in Java unit tests is a simple yet effective way to enhance the quality and clarity of test cases, ultimately contributing to the robustness and reliability of the codebase.
In your Java projects, consider implementing named parameters to elevate the clarity and comprehension of your unit tests, ultimately leading to more efficient and maintainable code.
Remember, clarity in test cases is not just a nicety but a key aspect of effective software development.
For further reading on writing expressive unit tests in Java, check out Writing Readable and Maintainable Unit Tests in Java.
Start incorporating named parameters into your Java tests today and experience the immediate benefits of improved test clarity and maintainability!