Understanding File Paths in Java NIO
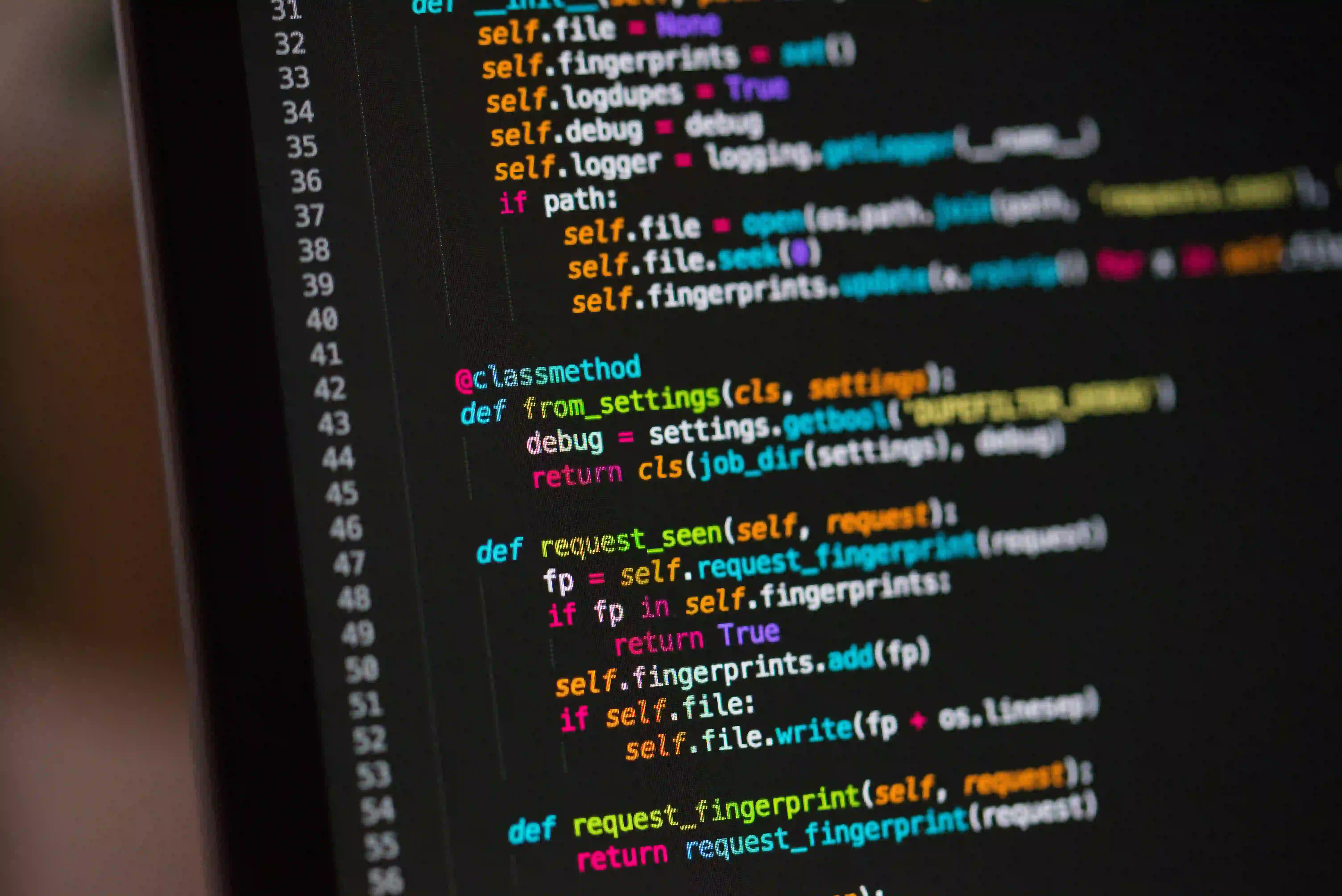
Understanding File Paths in Java NIO
When working with files in Java, it's essential to have a solid understanding of file paths. NIO (New I/O) in Java provides a powerful and flexible way to work with files and directories. In this blog post, we'll dive into the world of file paths in Java NIO and explore how to manipulate them effectively.
What are File Paths?
File paths are the unique locations of files or directories within a file system. In Java, file paths are represented by the Path
class, which is part of the java.nio.file
package. The Path
class provides methods for manipulating file and directory paths, resolving one path against another, and performing other operations related to file locations.
Creating a Path in Java
To create a Path
object in Java, you can use the Paths.get()
method, which returns a Path
by converting a path string or URI. Let's take a look at an example:
import java.nio.file.Path;
import java.nio.file.Paths;
public class PathExample {
public static void main(String[] args) {
Path path = Paths.get("/myDirectory/myFile.txt");
System.out.println(path);
}
}
In this example, we use the Paths.get()
method to create a Path
object that represents the file path "/myDirectory/myFile.txt". We then print the Path
object, which will display the file path.
Resolving Paths
One of the key features of the Path
class is the ability to resolve one path against another. This can be useful when working with relative paths or when combining multiple paths together. The resolve()
method is used to achieve this. Let's illustrate this with an example:
import java.nio.file.Path;
import java.nio.file.Paths;
public class PathResolutionExample {
public static void main(String[] args) {
Path dirPath = Paths.get("/myDirectory");
Path filePath = Paths.get("myFile.txt");
Path resolvedPath = dirPath.resolve(filePath);
System.out.println(resolvedPath);
}
}
In this example, we have a directory path and a file path. We use the resolve()
method to resolve the file path against the directory path, resulting in the combined path "/myDirectory/myFile.txt".
Normalizing Paths
File paths can sometimes contain redundant elements such as ".." (parent directory) or "." (current directory). The normalize()
method in the Path
class can be used to remove these redundant elements and produce a normalized path. Let's see an example:
import java.nio.file.Path;
import java.nio.file.Paths;
public class PathNormalizationExample {
public static void main(String[] args) {
Path path = Paths.get("/myDirectory/./mySubDirectory/../myFile.txt");
Path normalizedPath = path.normalize();
System.out.println(normalizedPath);
}
}
In this example, we have a path with redundant elements. We use the normalize()
method to obtain the normalized path "/myDirectory/myFile.txt" by resolving the redundant elements.
Retrieving File Information
The Path
class provides methods to retrieve information about a file or directory, such as whether it exists, its file name, parent directory, and more.
Let's take a look at an example that demonstrates how to retrieve file information using the Path
class:
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.Files;
public class FileInfoExample {
public static void main(String[] args) {
Path path = Paths.get("/myDirectory/myFile.txt");
System.out.println("File Name: " + path.getFileName());
System.out.println("Parent Directory: " + path.getParent());
System.out.println("File Exists: " + Files.exists(path));
}
}
In this example, we use the Path
methods to retrieve the file name, parent directory, and check whether the file exists using the Files.exists()
method.
Final Considerations
Understanding file paths is crucial when working with files and directories in Java. The Path
class in Java NIO provides a rich set of methods for manipulating file paths, resolving paths, normalizing paths, and retrieving file information. By mastering the concepts and techniques discussed in this blog post, you'll be well-equipped to work with file paths effectively in your Java applications.
To explore more about Java NIO and file handling, visit the official Java NIO documentation.
Happy coding!