Optimizing Running Totals in NoSQL Databases
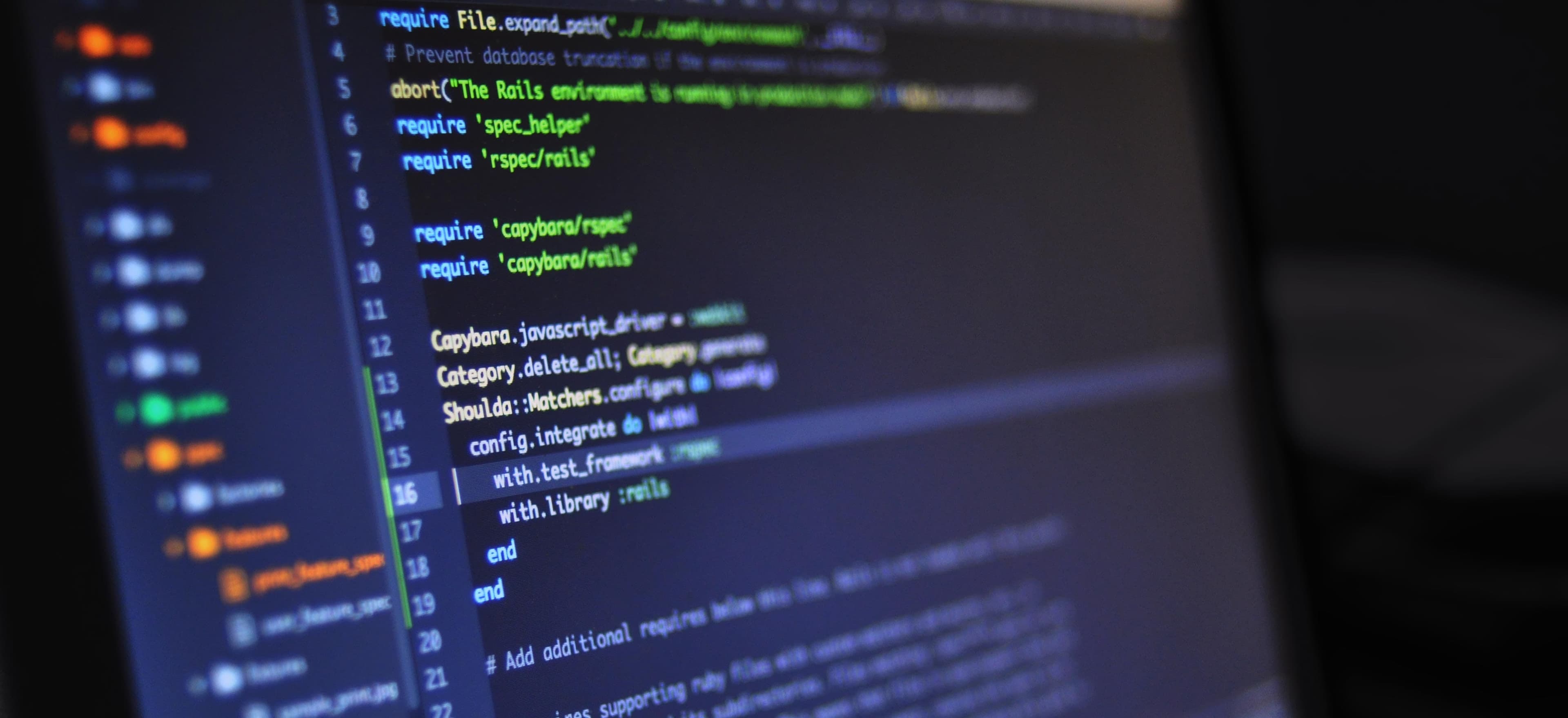
- Published on
Optimizing Running Totals in NoSQL Databases
NoSQL databases have gained popularity due to their ability to handle large volumes of data and scale horizontally. One common use case for NoSQL databases is maintaining running totals, such as calculating the cumulative sum of values over time. In this blog post, we will explore techniques to optimize running totals in NoSQL databases, focusing specifically on Java applications.
Understanding Running Totals
A running total, also known as a cumulative sum, is the summation of a sequence of numbers. In the context of databases, running totals are often used in financial applications to track account balances, in analytics to calculate moving averages, and in various other scenarios where aggregating data over time is necessary.
Using NoSQL Databases for Running Totals
NoSQL databases, such as MongoDB and Cassandra, offer flexibility and scalability for handling running totals. They store data in a non-tabular format, allowing for efficient distribution of data across clusters. However, querying and maintaining running totals in NoSQL databases can be challenging, especially as the data grows.
Optimizing Running Totals in Java
When working with NoSQL databases in Java, it's important to optimize the calculations of running totals to ensure efficient performance. Here are some techniques for optimizing running totals in NoSQL databases:
1. Denormalization
Denormalization involves storing redundant data to avoid expensive joins or calculations at query time. In the context of running totals, denormalization can be used to store pre-calculated cumulative sums alongside the source data.
// Example of denormalization in MongoDB
db.transactions.insert({
amount: 100,
date: ISODate("2022-01-01"),
cumulativeSum: 100
});
By denormalizing the cumulative sum into the transaction document, we avoid the need to recalculate the running total for each query. This approach improves read performance at the cost of increased storage and complexity in keeping the denormalized data in sync.
2. Map-Reduce
Map-Reduce is a programming model for processing and generating large datasets. In NoSQL databases like MongoDB, Map-Reduce can be used to efficiently calculate running totals across a collection of documents.
// Example of Map-Reduce in MongoDB
db.transactions.mapReduce(
function () {
emit(this.date, this.amount);
},
function (key, values) {
return Array.sum(values);
},
{
out: "runningTotals"
}
);
Map-Reduce allows for parallel processing of data and can handle running totals across large datasets. However, it's important to consider the overhead and complexity of Map-Reduce jobs, especially for real-time or frequently updated running totals.
3. Materialized Views
Materialized views store the results of precomputed queries, such as running totals, in a separate collection. In Java applications using NoSQL databases, materialized views can be maintained through triggers or asynchronous processes to update the running totals based on changes to the source data.
// Example of materialized views in MongoDB
db.transactions.aggregate([
{
$group: {
_id: null,
total: { $sum: "$amount" }
}
},
{
$out: "runningTotals"
}
]);
Materialized views can improve query performance by storing the precomputed running totals, but they require careful synchronization and maintenance to ensure consistency with the source data.
Wrapping Up
Optimizing running totals in NoSQL databases is crucial for maintaining efficient performance, especially as data grows. Techniques such as denormalization, Map-Reduce, and materialized views can be leveraged in Java applications to optimize running totals in NoSQL databases. By understanding these optimization techniques, developers can build robust and scalable solutions for handling running totals in their NoSQL databases.
In conclusion, optimizing running totals in NoSQL databases is essential for maintaining efficient performance, and Java developers have various techniques at their disposal to achieve this. By leveraging denormalization, Map-Reduce, and materialized views, developers can ensure that their applications can handle running totals effectively, regardless of the scale of their NoSQL databases.
For more information on NoSQL database optimization, you can refer to this article on MongoDB schema design best practices.
In summary, optimizing running totals in NoSQL databases is crucial for ensuring efficient performance as data grows. Java developers have access to several techniques, such as denormalization, Map-Reduce, and materialized views, which can be employed to optimize running totals in NoSQL databases. By understanding and implementing these techniques, developers can design efficient and scalable solutions for handling running totals in their NoSQL databases.
Checkout our other articles