Maximizing Team Efficiency with Agile Inventory Management
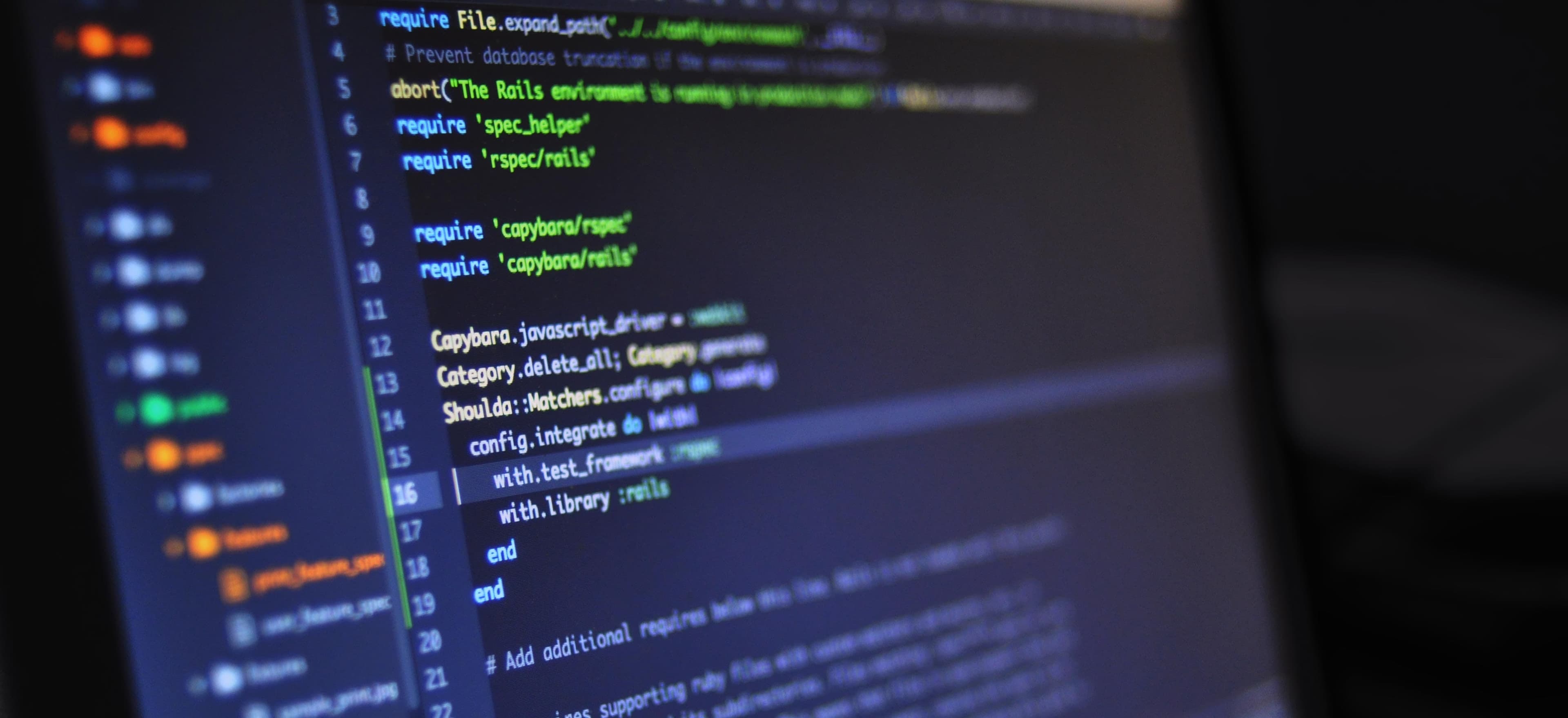
- Published on
Maximizing Team Efficiency with Agile Inventory Management
In the fast-paced world of software development, managing project resources efficiently is crucial for delivering high-quality products on time. One key resource that often gets overlooked is the management of third-party libraries, frameworks, and tools that are used in the development process. Efficiently managing these resources can save time, reduce errors, and improve overall team productivity.
Inventory Management in Java
Java is one of the most popular programming languages for building enterprise applications and managing third-party dependencies is a critical aspect of Java development. In this article, we will explore how to effectively manage dependencies in a Java project using agile inventory management techniques. We will delve into using tools like Maven and Gradle to automate dependency management and streamline the build process.
Why Efficient Inventory Management Matters
Before we delve into the technical implementation, let’s understand why efficient inventory management matters in Java development.
1. Dependency Conflict Resolution
In large projects with numerous dependencies, conflicts are bound to occur. Efficient inventory management provides mechanisms to resolve these conflicts, ensuring that the project utilizes the correct versions of dependencies without causing runtime issues.
2. Build Time Optimization
By managing dependencies efficiently, unnecessary downloads and redundant dependencies can be avoided, which in turn optimizes the build time. This is particularly crucial for large-scale projects where every second counts.
3. Enhanced Collaboration
Efficient inventory management facilitates better collaboration within development teams. Developers can easily share and synchronize project dependencies, reducing the chances of discrepancies and making the onboarding process smoother for new team members.
Now that we understand the importance of efficient inventory management, let’s explore how to achieve this in a Java project.
Using Maven for Dependency Management
Maven is a popular build automation tool used primarily for Java projects. It simplifies the build process and manages project dependencies using its powerful project object model (POM) concept.
Declaring Dependencies in Maven POM
Maven uses the pom.xml
file to declare project configurations, including dependencies. Here’s an example of declaring a dependency using Maven:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
</dependencies>
The above snippet illustrates how the Spring Framework’s core library is declared as a dependency in a Maven project. Maven allows us to specify the groupId, artifactId, and version of the dependency, ensuring that the correct version is used consistently across the team.
Resolving Dependency Conflicts with Maven
Maven’s dependency resolution mechanism ensures that conflicts are resolved based on predefined rules, typically favoring the nearest definition of a dependency. This alleviates the burden of manually resolving conflicts within the project code.
Gradle for Agile Dependency Management
Gradle is another powerful build automation tool that provides a flexible and efficient approach to dependency management. It uses Groovy or Kotlin DSL for scripting build configurations, and it is increasingly becoming the preferred choice for many Java projects.
Declaring Dependencies in Gradle Build Script
In Gradle, dependencies are declared in the build script using a concise and readable syntax. Here’s an example of declaring a dependency using Gradle:
dependencies {
implementation 'org.springframework:spring-core:5.3.8'
}
The above Gradle snippet achieves the same goal as the Maven snippet, declaring the Spring Framework’s core library as a dependency. Gradle’s expressive syntax significantly reduces boilerplate code, resulting in a clearer and more maintainable build script.
Efficient Task Automation with Gradle
One of the key advantages of Gradle is its support for task automation. This allows for seamless integration of various tasks related to dependency management, such as resolving, downloading, and updating dependencies, making it an ideal choice for agile inventory management.
Continuous Integration and Agile Inventory Management
In the context of agile software development, efficient inventory management extends beyond local development environments. Tools like Jenkins and Travis CI integrate seamlessly with dependency management tools like Maven and Gradle, enabling automated builds and tests in a continuous integration (CI) environment.
Jenkins Integration for Maven and Gradle
Jenkins, a widely used CI/CD tool, can be configured to build, test, and deploy Maven and Gradle projects. By leveraging Jenkins pipelines, teams can automate the entire build process, including dependency management tasks, ensuring that the inventory remains consistent across all stages of the development lifecycle.
Travis CI for GitHub Projects
For open-source projects hosted on GitHub, Travis CI provides an excellent platform for automating builds and tests. It seamlessly integrates with Maven and Gradle, allowing projects to benefit from continuous integration and efficient inventory management without additional infrastructure overhead.
Bringing It All Together
Effective agile inventory management is vital for maximizing team efficiency in Java development. By leveraging tools like Maven and Gradle, teams can streamline dependency management, resolve conflicts, and optimize build times. Integrating continuous integration tools such as Jenkins and Travis CI further enhances the efficiency of inventory management in a collaborative development environment.
Efficient inventory management may seem like a small piece of the puzzle, but its impact on team productivity and project deliverables should not be underestimated. Embracing agile inventory management practices empowers development teams to focus on building great software without being bogged down by dependency-related issues.
Checkout our other articles