Using Spring AOP to Throttle Methods
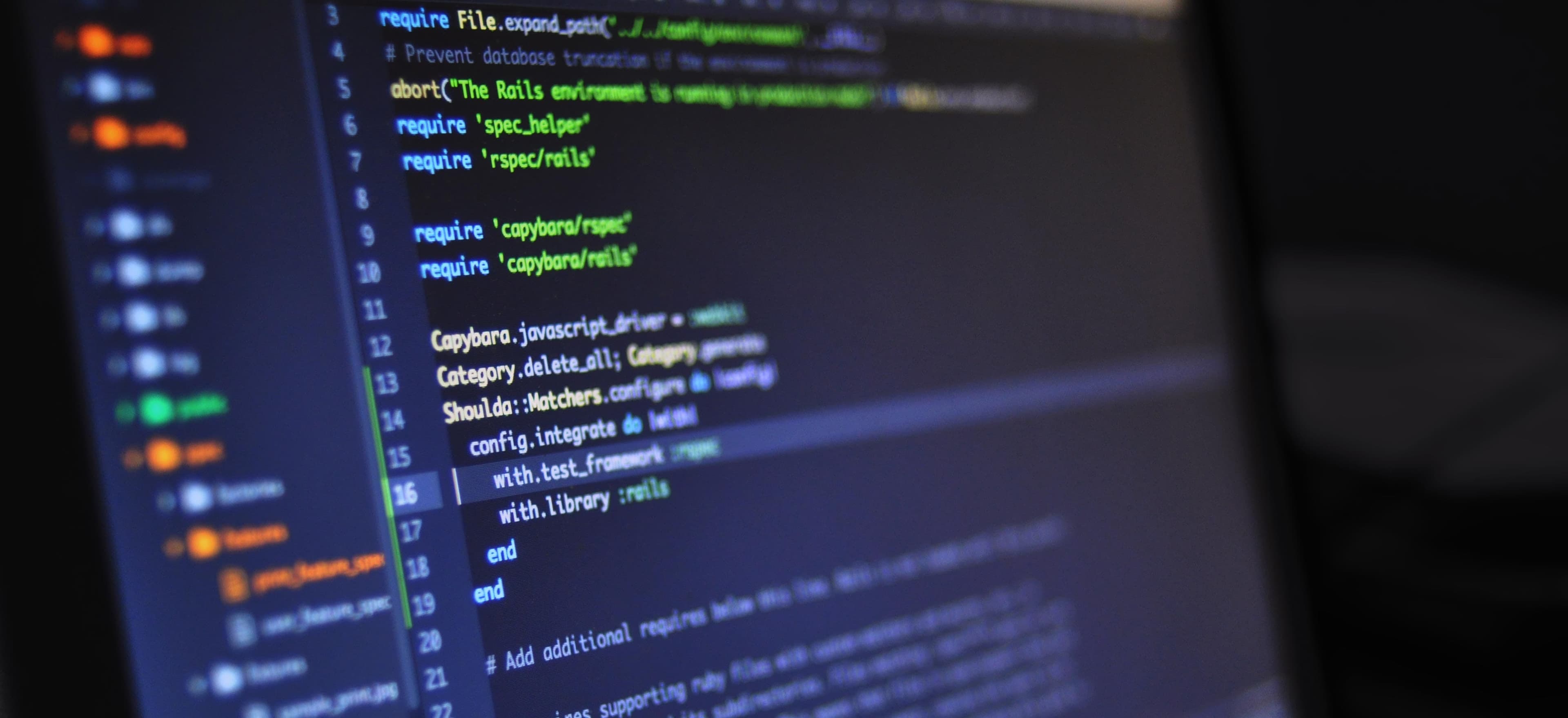
- Published on
Throttling Methods in Java with Spring Aspect-Oriented Programming (AOP)
In many software applications, there is a need to limit the frequency of method invocations, especially when the methods access limited resources or perform expensive operations. Throttling helps to control the rate at which these methods are allowed to execute, thereby preventing overloading and ensuring efficient resource utilization. In this article, we will explore how to implement method throttling in Java using Spring Aspect-Oriented Programming (AOP).
Understanding Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) is a programming paradigm that allows modularization of cross-cutting concerns. In the context of method throttling, AOP provides a convenient way to separate the throttling logic from the business logic of the application. It allows us to define reusable aspects that can be applied to multiple methods without modifying their original implementation.
Implementing Method Throttling with Spring AOP
To demonstrate method throttling using Spring AOP, we will consider a hypothetical scenario where we have a service method that processes incoming requests, and we want to limit the rate at which this method can be invoked.
Step 1: Setting Up the Project
First, we need to set up a Maven or Gradle project with the necessary dependencies for Spring AOP. We will also need the Spring framework for creating the service and defining the throttling aspect.
Step 2: Creating the Throttling Aspect
Next, we define a throttling aspect that will encapsulate the logic for limiting the method invocations. We can use annotations to specify the throttling configuration, such as the maximum allowed invocations per time unit.
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class ThrottlingAspect {
private static final int MAX_INVOCATIONS_PER_MINUTE = 100;
private static long lastInvocationTime = System.currentTimeMillis();
@Around("@annotation(Throttle)")
public Object throttleMethodInvocation(ProceedingJoinPoint joinPoint) throws Throwable {
long currentTime = System.currentTimeMillis();
if (currentTime - lastInvocationTime < 60000 / MAX_INVOCATIONS_PER_MINUTE) {
throw new IllegalAccessException("Throttling limit exceeded");
}
lastInvocationTime = currentTime;
return joinPoint.proceed();
}
}
In the above code snippet, we define a ThrottlingAspect
class annotated with @Aspect
and @Component
to make it a managed bean by Spring. We also define a method throttleMethodInvocation
annotated with @Around
to intercept the target method invocation. Within this method, we check the time elapsed since the last invocation and compare it with the configured throttle limit. If the limit is exceeded, an exception is thrown, indicating that the method invocation has been throttled.
Step 3: Applying Throttling to a Service Method
Now, let's apply the throttling aspect to a specific service method using a custom annotation @Throttle
.
import org.springframework.stereotype.Service;
@Service
public class RequestProcessingService {
@Throttle
public void processRequest(Request request) {
// Method implementation
}
}
In this example, we annotate the processRequest
method with @Throttle
, which triggers the ThrottlingAspect
to enforce the throttling logic whenever this method is called.
Step 4: Configuring Spring AOP
In the Spring configuration, we need to enable the use of AspectJ proxies and component scanning to allow the ThrottlingAspect
to be detected and applied to the annotated methods.
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
// Configuration beans and other settings
}
By enabling @EnableAspectJAutoProxy
, we instruct Spring to create the necessary AOP proxies to apply the aspect behavior.
Final Considerations
In this article, we have explored how to implement method throttling in Java using Spring Aspect-Oriented Programming. By leveraging AOP, we were able to separate the throttling concerns from the business logic, making the code more modular and maintainable. Method throttling is a useful technique for managing resource utilization and preventing overloading in a variety of applications.
By following the steps outlined in this article, you can easily integrate method throttling into your Java applications, ensuring optimal performance and resource management.
For further reading on Spring AOP and method throttling, you can refer to the official Spring documentation and explore more advanced AOP features such as pointcuts and advice types.
Start implementing method throttling in your Java applications with Spring AOP and experience the benefits of controlled method invocations for improved application stability and performance.