Troubleshooting Multiple View Definitions in Spring MVC
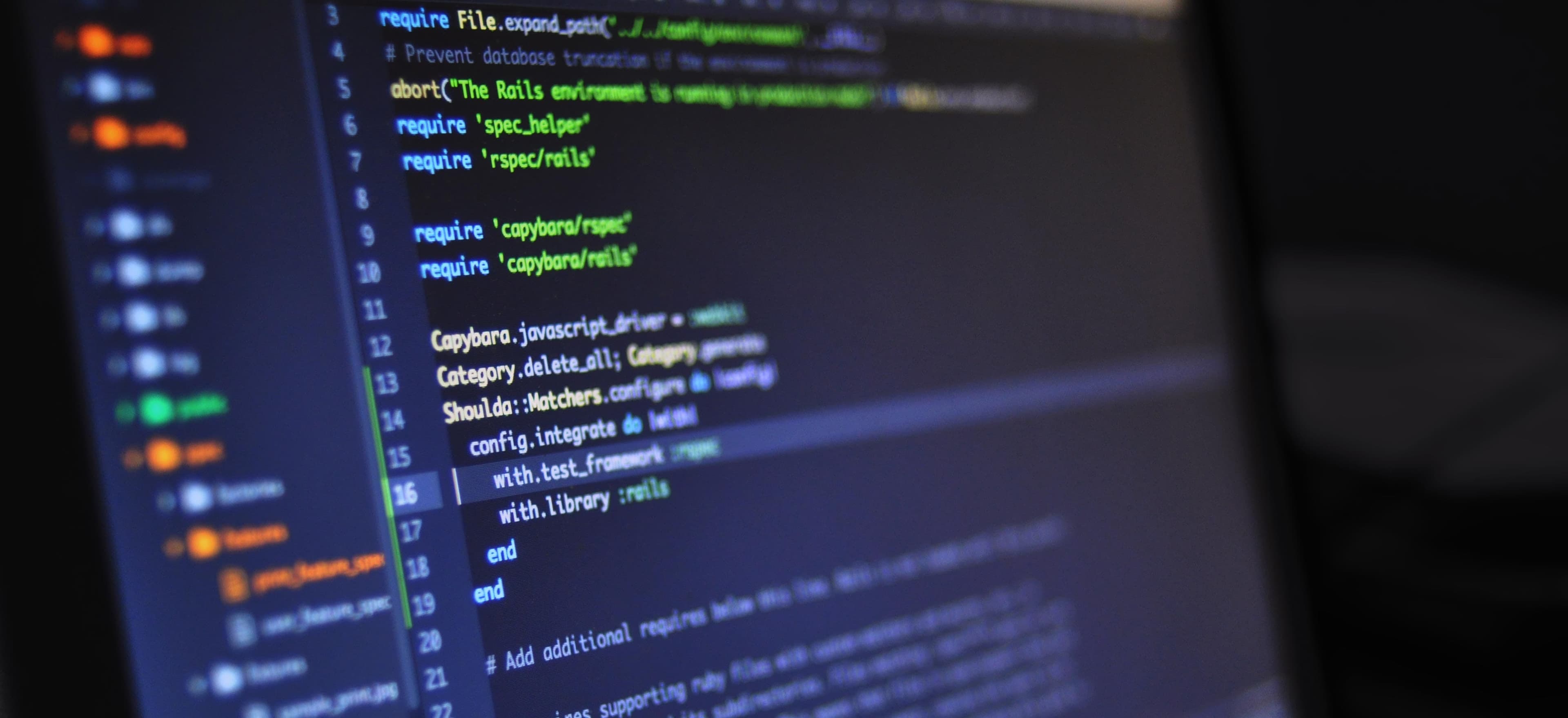
- Published on
Troubleshooting Multiple View Definitions in Spring MVC
Are you encountering issues with defining and using multiple views in your Spring MVC application? Don't worry, you're not alone. This blog post will walk you through common problems related to multiple view definitions in Spring MVC and how to troubleshoot them effectively.
Understanding View Resolvers in Spring MVC
Before we dive into troubleshooting, let's quickly review the concept of view resolvers in Spring MVC. View resolvers are responsible for mapping logical view names to actual views. In a typical Spring MVC application, you might define multiple view resolvers to handle different types of views, such as JSP, Thymeleaf, or FreeMarker.
Problem: Multiple View Definitions not Resolving Correctly
One common issue that developers encounter is having multiple view definitions but encountering errors when trying to resolve them. This can manifest as unresolved view names or the wrong view being rendered.
Let's look at an example configuration:
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public ViewResolver jspViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/views/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
@Bean
public ViewResolver thymeleafViewResolver(SpringTemplateEngine templateEngine) {
ThymeleafViewResolver viewResolver = new ThymeleafViewResolver();
viewResolver.setTemplateEngine(templateEngine);
return viewResolver;
}
}
In this example, we have configured two view resolvers: one for JSP views and one for Thymeleaf views. However, when trying to render a view, Spring MVC may not resolve the correct view resolver, leading to unexpected behavior.
Solution: View Resolver Order
One possible reason for this issue is the order in which the view resolvers are prioritized. By default, Spring MVC uses a ContentNegotiatingViewResolver
to determine the appropriate view resolver based on the request and available view resolvers. If the order of view resolvers is not specified, it can lead to conflicts.
To address this, you can explicitly define the order of the view resolvers using the configureViewResolvers
method:
@Override
public void configureViewResolvers(ViewResolverRegistry registry) {
registry.viewResolver(jspViewResolver()).order(1);
registry.viewResolver(thymeleafViewResolver(templateEngine())).order(2);
}
In this code snippet, we are specifying the order of the view resolvers, ensuring that the JSP view resolver is prioritized over the Thymeleaf view resolver. This explicit ordering can help resolve conflicts and ensure that the correct view resolver is used for rendering views.
Problem: Unsupported View Types
Another common issue when working with multiple view definitions is encountering unsupported view types. For example, you might define a view resolver for a specific view technology, only to find that Spring MVC does not recognize or support it.
Let's consider an example where we attempt to use a FreeMarker view resolver:
@Bean
public FreeMarkerViewResolver freeMarkerViewResolver() {
FreeMarkerViewResolver viewResolver = new FreeMarkerViewResolver();
viewResolver.setPrefix("/WEB-INF/views/");
viewResolver.setSuffix(".ftl");
return viewResolver;
}
In this case, even after configuring the FreeMarker view resolver, Spring MVC might not recognize FreeMarker templates as valid view types.
Solution: View Resolver Configuration
When dealing with unsupported view types, it's essential to ensure that the necessary configurations are in place. In the case of FreeMarker, you need to register the FreeMarker template engine with Spring MVC to enable support for FreeMarker views.
@Bean
public FreeMarkerConfigurer freeMarkerConfigurer() {
FreeMarkerConfigurer configurer = new FreeMarkerConfigurer();
configurer.setTemplateLoaderPath("/WEB-INF/views/");
return configurer;
}
@Bean
public FreeMarkerViewResolver freeMarkerViewResolver() {
FreeMarkerViewResolver viewResolver = new FreeMarkerViewResolver();
viewResolver.setCache(false);
viewResolver.setPrefix("");
viewResolver.setSuffix(".ftl");
viewResolver.setContentType("text/html; charset=UTF-8");
return viewResolver;
}
Here, we've registered a FreeMarkerConfigurer
to configure FreeMarker settings and a FreeMarkerViewResolver
to handle the FreeMarker views. By setting the appropriate configurations and providing Spring MVC with the necessary information, we can ensure that unsupported view types are recognized and supported.
Key Takeaways
In conclusion, troubleshooting multiple view definitions in Spring MVC often involves addressing issues related to view resolver ordering and unsupported view types. By carefully configuring and prioritizing view resolvers, as well as ensuring that the necessary configurations for specific view types are in place, you can effectively overcome these challenges and achieve seamless handling of multiple views in your Spring MVC application.
Remember, understanding the underlying mechanisms of view resolution and being aware of the various configurations at your disposal are essential for successful troubleshooting. With these insights and the solutions presented in this blog post, you're well-equipped to tackle any issues related to multiple view definitions in Spring MVC.
I hope you found this guide helpful. For more in-depth understanding, you can explore the official Spring Framework Documentation and Baeldung's Spring MVC tutorials. Stay tuned for more insightful content!