Optimizing Data Export in PrimeFaces
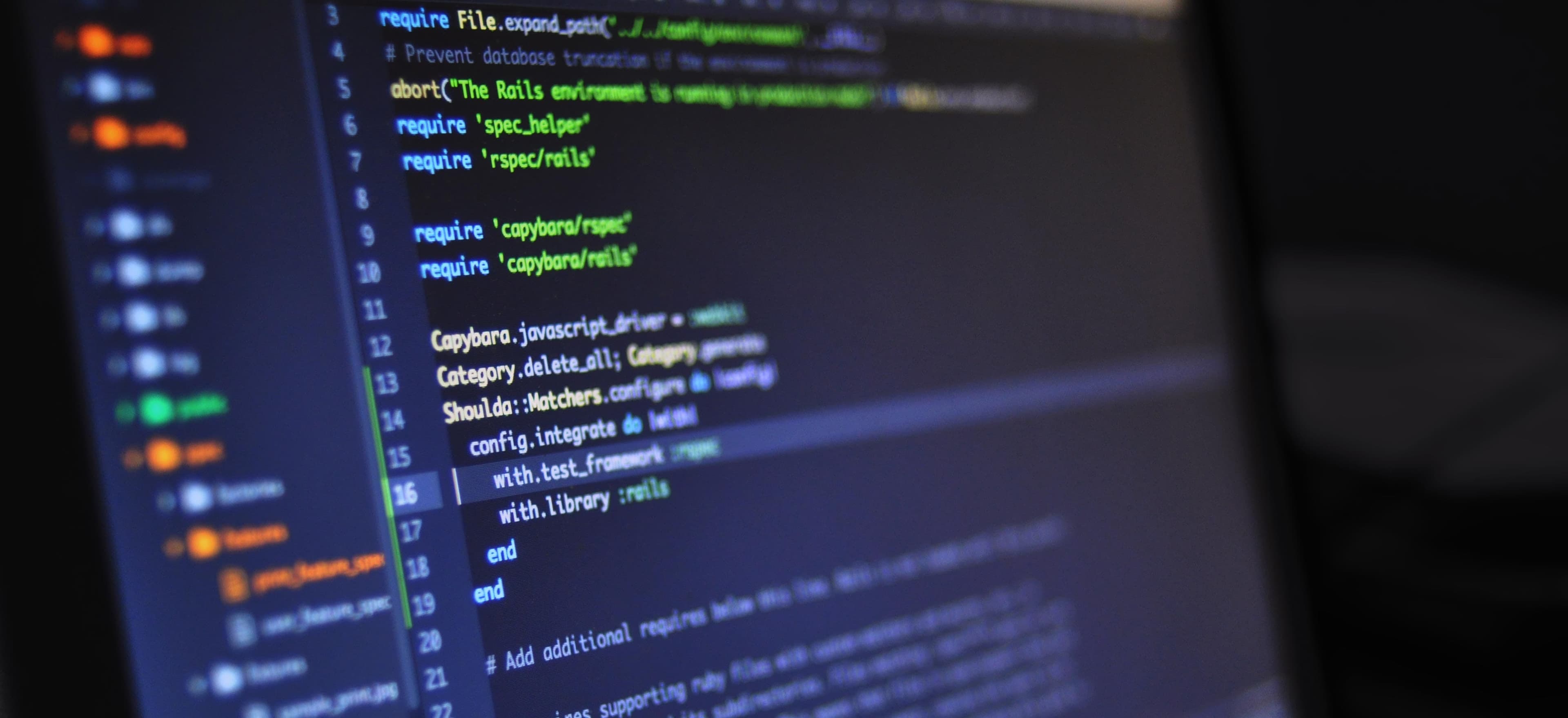
- Published on
Optimizing Data Export in PrimeFaces
Data export is a common requirement in web applications, especially in enterprise-level applications. It allows users to generate reports, analyze data in external tools, and share information with others. In Java web applications, PrimeFaces is a popular choice for building user interfaces, and it provides a convenient way to export data to various formats such as CSV, Excel, PDF, and XML. However, as the volume of data grows, it becomes crucial to optimize the data export functionality for performance and scalability.
In this article, we'll explore some tips and best practices for optimizing data export in PrimeFaces. We'll cover techniques to improve the export performance and enhance the user experience.
Understanding the Export Process in PrimeFaces
Before diving into optimization strategies, it's essential to understand how data export works in PrimeFaces.
PrimeFaces provides a set of components such as DataTable
, DataList
, and DataGrid
for displaying tabular data. These components offer built-in support for exporting data to various formats. When exporting data, PrimeFaces essentially processes the data from the component and generates the output in the selected format. This process involves iterating through the data, creating the appropriate output structure, and writing the content to the output stream.
Tip 1: Consider Lazy Data Loading
When dealing with large datasets, fetching and processing all the data at once can be inefficient and resource-intensive. PrimeFaces supports lazy loading for its data components, which allows data to be loaded dynamically as needed. By implementing lazy loading, you can significantly reduce the initial load time and memory consumption, especially when the dataset is extensive.
Here's an example of implementing lazy loading with PrimeFaces DataTable
:
<p:dataTable value="#{lazyDataModel}" var="item" lazy="true">
<!-- Columns definition -->
</p:dataTable>
By setting the lazy
attribute to true
and providing a custom lazy data model, you can enable lazy loading for the DataTable
. The lazy data model will be responsible for fetching and processing data on-demand, improving the performance of data export operations.
Tip 2: Limit the Exported Data
In scenarios where users may need to export a subset of the data or apply filters before exporting, it's beneficial to limit the exported data to only what the user needs. This reduces the processing time and the size of the exported file, leading to faster exports and improved user experience.
PrimeFaces provides built-in support for applying filters and pagination to data components. You can utilize these features to allow users to refine the data before initiating an export operation. By exporting only the visible or filtered data, the export process becomes more efficient, especially when dealing with large datasets.
Tip 3: Optimize Resource Utilization
During the export process, be mindful of resource utilization to prevent performance bottlenecks. PrimeFaces allows you to customize the export functionality by providing options to set various configurations and optimizations.
For example, when exporting to Excel or PDF, you can optimize the layout and styling to minimize the file size and rendering time. Additionally, you can configure the exporter to handle large datasets efficiently by setting appropriate buffer sizes and pagination settings.
<p:dataTable ...>
<p:column ... />
<!-- More columns -->
<p:ajax event="rowSelect" listener="#{bean.onRowSelect}" update=":form:exportButton" />
</p:dataTable>
<p:commandButton id="exportButton" value="Export" icon="pi pi-file-excel"
onclick="PrimeFaces.monitorDownload(start, stop)" ajax="false">
<p:dataExporter type="xls" target="dataTable" fileName="export" />
</p:commandButton>
In the example above, the p:dataExporter
component is configured to export the data from the dataTable
component to an Excel file. By specifying the appropriate type
, target
, and fileName
, you can optimize the export process to generate the desired output efficiently.
Tip 4: Server-side Data Processing
For very large datasets, consider performing the data processing and export operation on the server side. Instead of transferring the entire dataset to the client and processing it there, you can delegate the export process to the server, where the resources and performance are more controllable.
You can achieve server-side data processing by invoking server-side actions or services to retrieve and process the data for export. This approach minimizes the load on the client-side resources and network bandwidth, resulting in faster exports and reduced memory overhead.
Tip 5: Cache the Data for Repeated Exports
In cases where the same data is likely to be exported multiple times, consider caching the processed data to avoid redundant processing. Caching the data eliminates the need to regenerate the export content for subsequent exports of the same dataset, leading to faster export operations.
You can use server-side caching mechanisms, such as in-memory caching or distributed caching solutions, to store the processed data temporarily. By caching the data, you can improve the responsiveness of the export feature and reduce the overall processing load on the server.
The Last Word
Optimizing data export in PrimeFaces is essential for ensuring efficient performance and a smooth user experience, particularly when dealing with large datasets. By understanding the export process, implementing lazy loading, limiting the exported data, optimizing resource utilization, leveraging server-side processing, and caching the data, you can significantly improve the efficiency of data export operations in your Java web applications.
In summary, adopting these optimization strategies can lead to faster export times, reduced memory overhead, and an overall enhanced user experience when working with data export in PrimeFaces.
Remember, as the volume of data increases, the need for optimization becomes more critical. By implementing these strategies, you can ensure that your PrimeFaces-based web application delivers an efficient and responsive data export feature that meets the performance expectations of your users.
Remember, the key to effective optimization is a thorough understanding of the underlying processes and a strategic application of the appropriate techniques. By implementing these strategies, you can ensure that your PrimeFaces-based web application delivers an efficient and responsive data export feature that meets the performance expectations of your users.