Asynchronous Servlets: Handling Long-Running Tasks
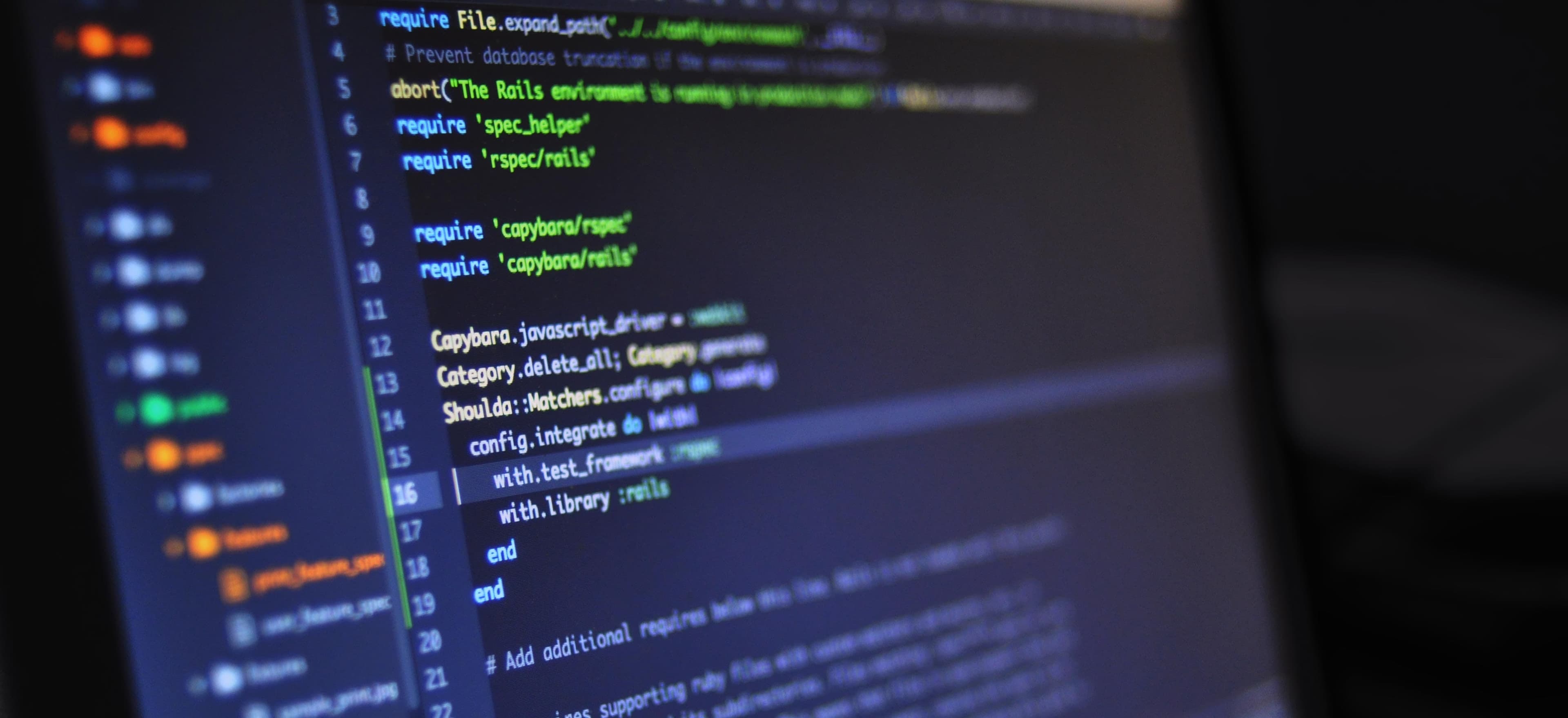
- Published on
Understanding Asynchronous Servlets in Java
In the world of web applications, there are often scenarios where a client request triggers a long-running process on the server side. This could be anything from processing a large file to running a complex database query. Handling such long-running tasks with traditional synchronous servlets can lead to performance bottlenecks and a poor user experience. This is where asynchronous servlets come to the rescue.
What Are Asynchronous Servlets?
In Java, servlets are classes that extend the capabilities of servers that host applications accessed by means of HTTP. An asynchronous servlet is a feature introduced in the Servlet 3.0 specification, allowing servlets to handle long-lived requests without blocking threads.
When a request is initiated by a client, it typically results in a thread being allocated to handle the request for the duration of its execution. For long-running tasks, this approach can lead to thread wastage and potential denial of service. Asynchronous servlets address this issue by allowing the request thread to return immediately to the container, while the long-running task continues in the background.
Implementing Asynchronous Servlets
To implement an asynchronous servlet in Java, you need to follow these steps:
- Start the Asynchronous Context: Obtain the asynchronous context from the request and begin the asynchronous cycle.
- Perform the Long-Running Task: Execute the long-running task on a separate thread.
- Complete the Asynchronous Cycle: Once the task is completed, dispatch or complete the asynchronous cycle.
Code Example
Let’s dive into an example to illustrate the implementation of an asynchronous servlet.
@WebServlet("/asyncTask")
public class AsyncServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
AsyncContext asyncContext = request.startAsync();
CompletableFuture.runAsync(() -> {
// Perform the long-running task here
// This could be processing a file, invoking a web service, or any other time-consuming operation
asyncContext.complete();
});
}
}
In this example, when a client makes a GET request to /asyncTask
, the servlet starts an asynchronous cycle and offloads the long-running task to a separate thread using CompletableFuture.runAsync
. Once the task is completed, asyncContext.complete()
is called to finish the asynchronous cycle.
Advantages of Asynchronous Servlets
Using asynchronous servlets for handling long-running tasks offers several advantages, including:
- Improved Scalability: By not tying up server resources with long-lived requests, asynchronous servlets allow the server to handle a larger number of simultaneous requests without consuming excessive memory or CPU.
- Better Response Times: With the main thread released from waiting for the long-running task to complete, the server can respond to other incoming requests more promptly, leading to improved overall response times.
- Resource Efficiency: Asynchronous handling allows the container to serve a larger number of concurrent users without requiring a thread for each request, leading to better resource utilization.
Best Practices for Asynchronous Servlets
When working with asynchronous servlets, it’s important to keep a few best practices in mind:
- Monitor Resource Utilization: Although asynchronous servlets can improve scalability, it’s crucial to monitor server resources to ensure that long-running tasks do not consume excessive system resources. Tools like JVisualVM can help in this regard.
- Timeout Management: Implement mechanisms to handle timeouts for long-running tasks, ensuring that resources are not held indefinitely. The Servlet 3.0 specification provides facilities for setting timeouts on asynchronous requests.
- Error Handling: Properly handle errors and exceptions that may occur during the execution of long-running tasks. Log and communicate errors effectively to maintain application robustness.
Key Takeaways
In the world of web applications, the ability to handle long-running tasks efficiently is crucial for providing a seamless user experience and ensuring optimal resource utilization. Asynchronous servlets in Java provide a powerful way to manage such tasks, offering improved scalability and response times while efficiently utilizing server resources.
By embracing the asynchronous nature of servlets and following best practices, developers can ensure that their applications are well-equipped to handle long-lived requests without compromising on performance or resource efficiency.
To delve deeper into asynchronous servlets, check out this detailed guide from Oracle that provides in-depth insights into the asynchronous features of Java Servlets.
In conclusion, mastering asynchronous servlets can significantly enhance the performance and scalability of your Java web applications. So, next time you encounter a long-running task, consider leveraging the power of asynchronous servlets to handle it effectively.