Overusing Java's String Class Can Lead to Performance Issues
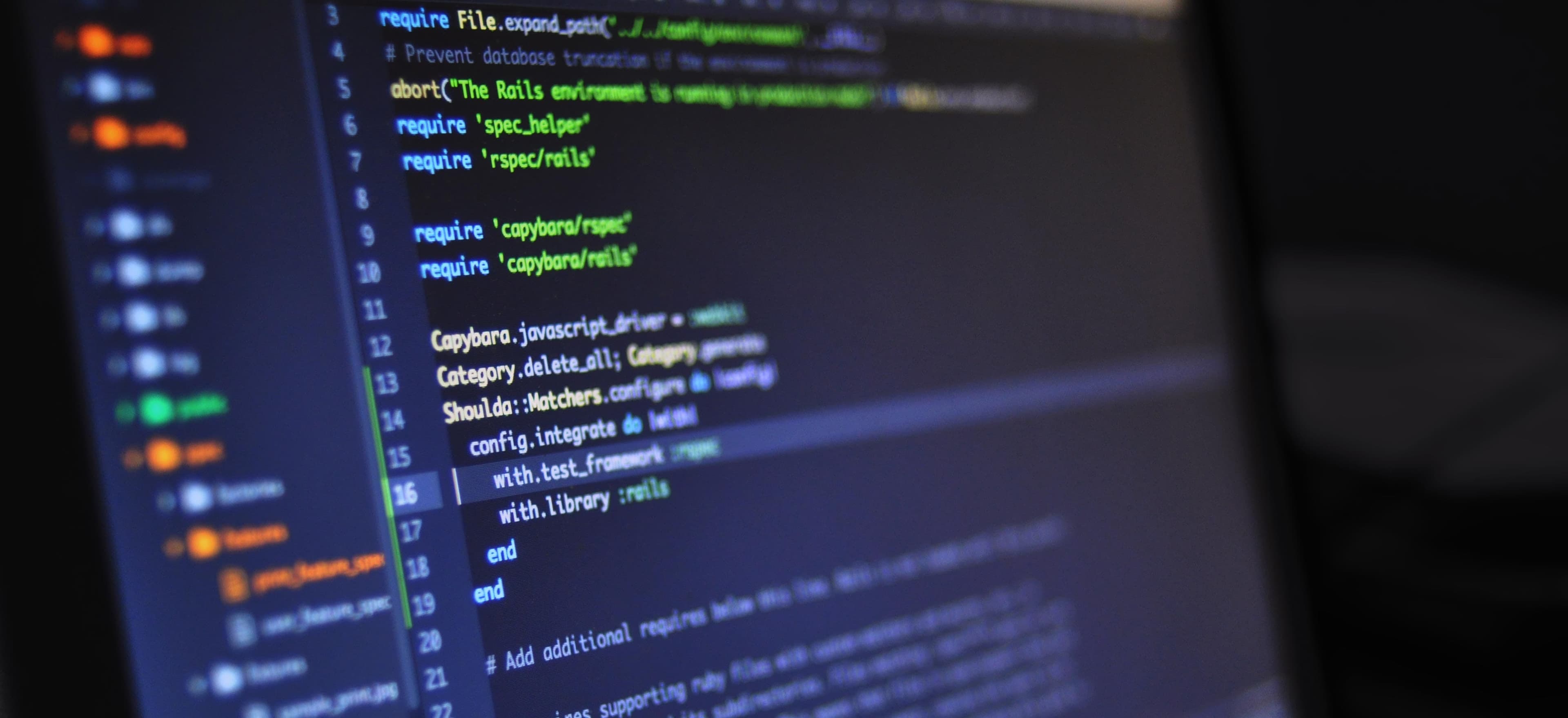
- Published on
The Problem with Overusing Java's String Class
When it comes to Java programming, the String
class is one of the most commonly used classes. It's versatile, powerful, and simplifies many programming tasks. However, overreliance on the String
class can lead to performance issues, especially in scenarios where a large number of string operations are being performed.
In this blog post, we'll delve into the potential performance problems associated with overusing the String
class in Java. We'll explore why these issues occur, how to identify them, and most importantly, how to mitigate them.
Understanding the Performance Impact
Java's String
class is immutable, meaning that once a String
object is created, it cannot be modified. Any operation that appears to modify the content of a String
object actually creates a new String
object with the modified content. While this immutability has its benefits in terms of thread safety and state predictability, it can result in excessive memory consumption and increased garbage collection overhead when not used judiciously.
Consider the following scenario:
String result = "";
for (int i = 0; i < 1000; i++) {
result += "some text";
}
At first glance, this code seems harmless. However, each time the +=
operation is performed, a new String
object is created to store the concatenated result. With 1000 iterations, this quickly leads to the creation of 1000 String
objects, each of which needs to be garbage collected. This can have a significant impact on both memory consumption and execution time, especially in more complex real-world scenarios.
The Solution: Using StringBuilder
To mitigate the performance issues associated with frequent string concatenation, Java provides the StringBuilder
class. Unlike String
, StringBuilder
is mutable, allowing for efficient modification of character sequences.
Let's revisit the previous example using StringBuilder
:
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append("some text");
}
String finalResult = result.toString();
In this revised code, a StringBuilder
object is used to efficiently append the "some text" string in each iteration. Only one StringBuilder
object is created, and the final String
result is generated using the toString
method. This approach significantly reduces the number of objects created and provides a more efficient way to handle string concatenation.
Additional Considerations
While using StringBuilder
addresses the issue of frequent string concatenation, it's important to note that not all string operations necessarily lead to performance problems. Care should be taken to identify the specific scenarios where performance bottlenecks are observed.
Additionally, modern Java versions provide the StringJoiner
class, which offers a streamlined way to join multiple strings with a delimiter. This can be particularly useful when dealing with collections or arrays of strings.
Bringing It All Together
In summary, while the String
class is a fundamental and powerful component of Java programming, overusing it can lead to performance issues, especially in scenarios involving frequent string manipulation. By understanding the impact of excessive string operations and leveraging alternatives such as StringBuilder
and StringJoiner
, developers can mitigate these performance concerns and ensure the efficient handling of string-related tasks in their Java applications.
By optimizing string operations, developers can improve the overall performance and responsiveness of their Java applications, leading to a more robust and reliable software experience for end users.
For more in-depth insights into Java performance optimization, check out Java Performance: The Definitive Guide, a comprehensive resource on maximizing the performance of Java applications.
Remember, while it's important to write code that's clear and maintainable, it's equally crucial to consider the performance implications of every design decision. This balance will ultimately lead to well-performing and efficient Java applications.