Converting InputStream to String in Java
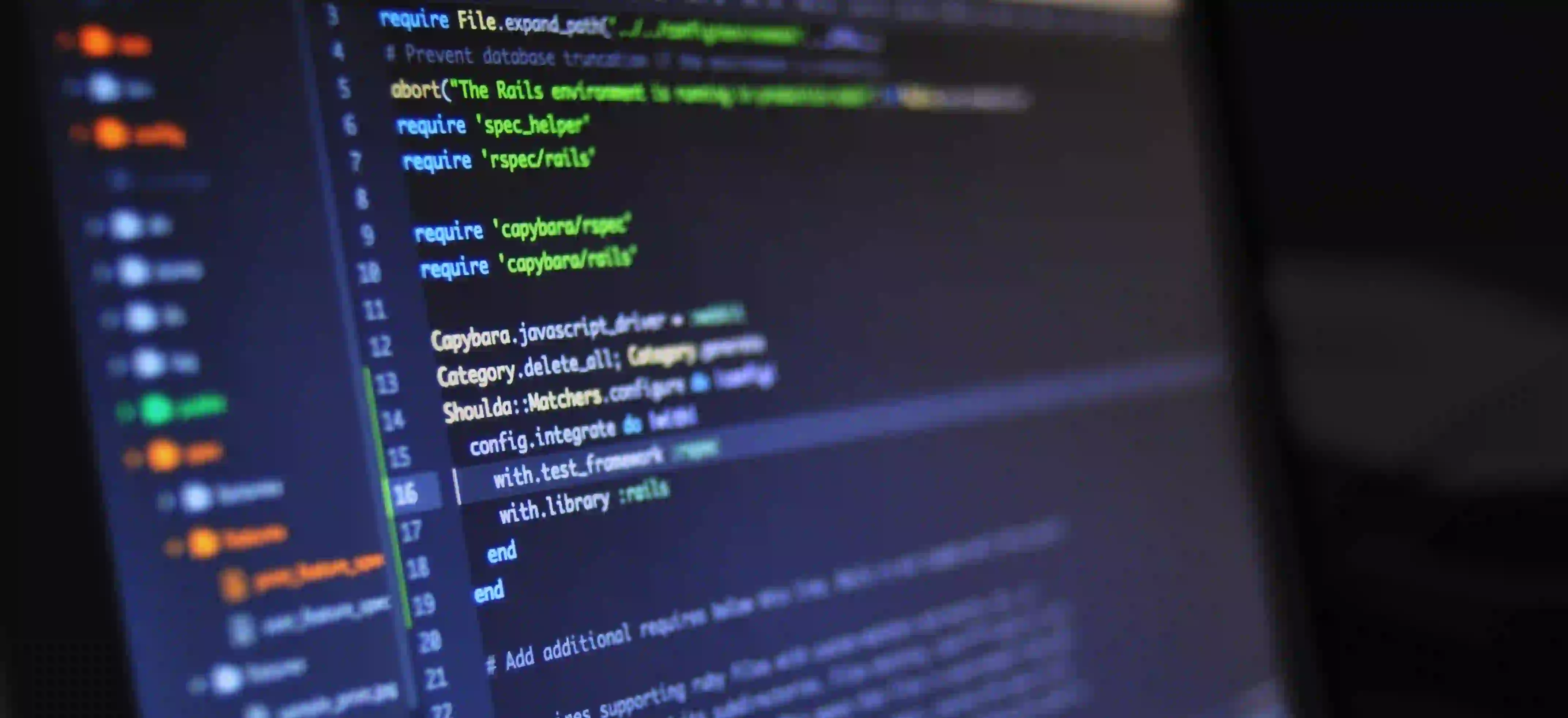
Converting InputStream to String in Java
In Java, it's a common task to convert an InputStream
to a String
, especially when dealing with data coming from network connections, files, or other sources. There are multiple ways to achieve this, and in this blog post, we will explore some of the most effective and efficient methods for doing so.
Using BufferedReader and StringBuilder
One of the simplest and widely used methods to convert an InputStream
to a String
is by using BufferedReader
and StringBuilder
. Here's a quick example of how to do this:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class InputStreamToString {
public static String convertToString(InputStream inputStream) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
return stringBuilder.toString();
}
}
In this code, we create a BufferedReader
to read the input stream line by line, and then we use a StringBuilder
to build the complete String
. This method is efficient for reading large amounts of data from the input stream and can handle different types of encodings.
Using Scanner
Another way to convert an InputStream
to a String
is by using the Scanner
class. Here's how you can achieve this:
import java.io.InputStream;
import java.util.Scanner;
public class InputStreamToString {
public static String convertToString(InputStream inputStream) {
Scanner scanner = new Scanner(inputStream).useDelimiter("\\A");
return scanner.hasNext() ? scanner.next() : "";
}
}
In this approach, we create a Scanner
, set the delimiter to the beginning of the input, and then simply convert it to a String
. This method is concise and straightforward, making it suitable for smaller input streams.
Using Apache Commons IO
Apache Commons IO library provides a convenient utility class IOUtils
that simplifies the process of converting an InputStream
to a String
. You can include this library in your project and use the following method:
import org.apache.commons.io.IOUtils;
import java.io.IOException;
import java.io.InputStream;
public class InputStreamToString {
public static String convertToString(InputStream inputStream) throws IOException {
return IOUtils.toString(inputStream, "UTF-8");
}
}
By using IOUtils
from Apache Commons IO, you can achieve the conversion in a single line of code, handling the encoding internally. This method is particularly useful when working with larger projects and where external libraries are permitted.
Using Java NIO
Java NIO (New I/O) provides a more modern and efficient way to convert an InputStream
to a String
. Here's an example of how you can do this:
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
public class InputStreamToString {
public static String convertToString(InputStream inputStream) throws IOException {
return new String(inputStream.readAllBytes(), StandardCharsets.UTF_8);
}
}
With Java NIO, you can utilize the readAllBytes
method to read all bytes from the input stream and then create a String
using the desired charset. This method is efficient and suitable for modern Java applications.
To Wrap Things Up
In this blog post, we have explored various methods for converting an InputStream
to a String
in Java. Each method has its own advantages, and the choice of method depends on the specific requirements of your project. Whether you prefer the simplicity of using BufferedReader and StringBuilder, the convenience of Apache Commons IO, or the modern approach of Java NIO, you now have multiple options to handle this common task efficiently.
When working on real-world projects, it's essential to consider error handling, resource management, and performance implications when choosing the appropriate method for converting InputStream
to String
. By understanding these techniques, you will be better equipped to handle data manipulation in your Java applications.
Remember, the best method for your specific use case may depend on factors such as the size of the input stream, the encoding, and the overall design of your application. It's important to evaluate each method in context and choose the one that best aligns with your project's requirements.
By mastering the art of converting InputStream
to String
, you are one step closer to becoming a proficient Java developer, adept at handling data streams with finesse and efficiency.
Happy coding!
This blog post was brought to you by JavaMaster - your one-stop destination for all things Java development.
Did this article help you understand how to convert InputStream
to String
in Java? Let me know in the comments below!