Handling Duplicate Files in Gradle Build Process
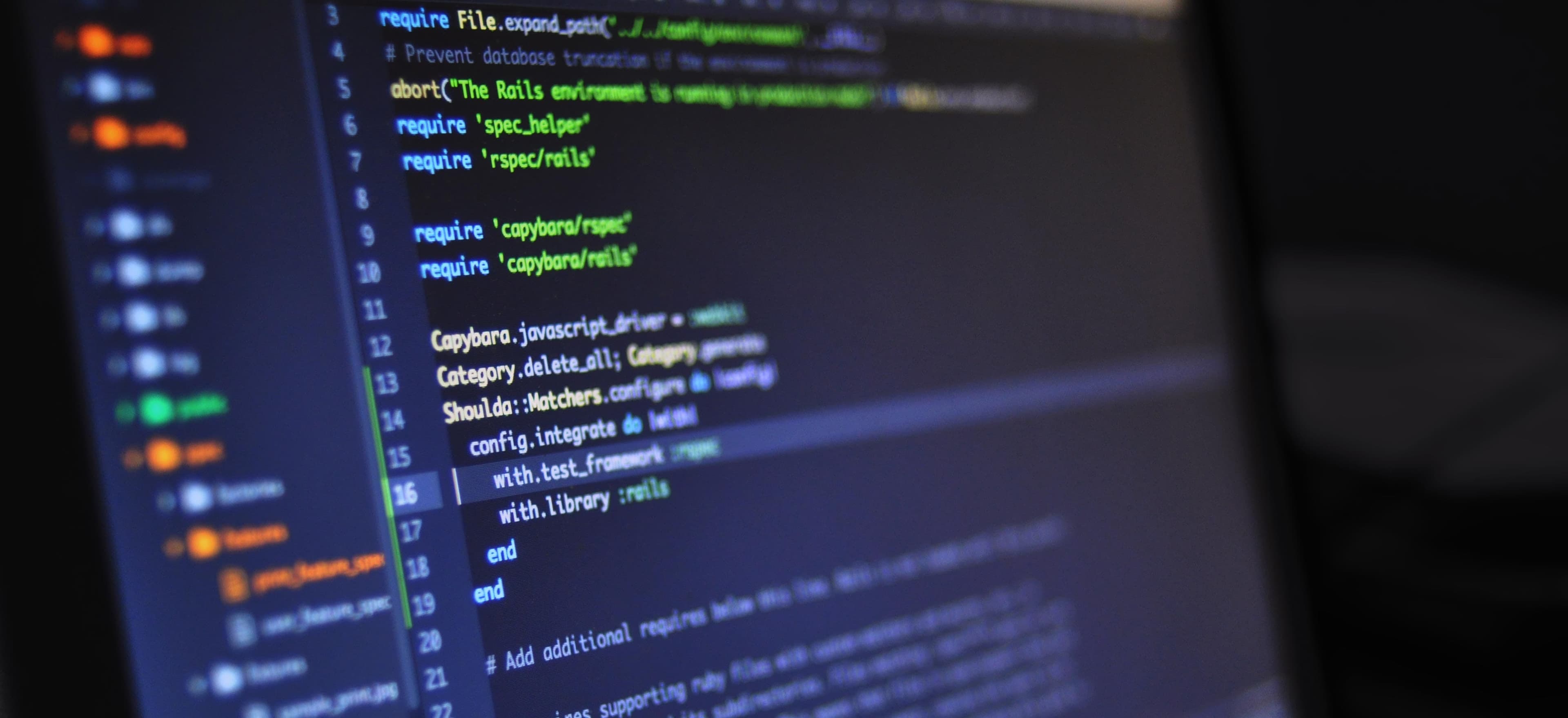
- Published on
Handling Duplicate Files in Gradle Build Process
Duplicate files can often become a headache in any software development project, and a Gradle build process is no exception. In this blog post, we will explore ways to handle duplicate files in the Gradle build process and discuss why it is important to address this issue. We will also provide practical examples and code snippets to demonstrate how to tackle duplicate files effectively.
Why Duplicate Files Matter in a Gradle Build Process
Duplicate files in a Gradle build process can lead to several problems, including:
-
Ambiguous Resource Resolution: Duplicate files can cause ambiguity when resolving resources, leading to unexpected behavior or errors in the application.
-
Increased Build Size: Including duplicate files in the build can increase the size of the final artifact, resulting in longer build times and larger deployment packages.
-
Potential Name Collisions: Duplicate files may have the same name but different content, potentially causing conflicts and overwriting of files during the build process.
Now that we understand the importance of addressing duplicate files, let's delve into some strategies to handle them effectively in a Gradle build.
Excluding Duplicate Files in Gradle Dependencies
One common scenario where duplicate files may arise is when resolving dependencies in a Gradle project. Suppose we have two dependencies that contain the same file, such as a configuration file or a resource. We can use the exclude
directive in the dependencies
block to exclude the specific files that are causing conflicts.
dependencies {
implementation('group:artifact:version') {
exclude group: 'com.example', module: 'duplicate-file'
}
}
In this example, we are excluding the duplicate-file
module from the com.example
group to prevent it from conflicting with another module.
It's essential to carefully identify the duplicate files and their sources before excluding them, as indiscriminate exclusion may lead to missing essential resources or functionality.
Resolving Duplicate Files Using Gradle's Copy Task
Another approach to handling duplicate files is to use Gradle's Copy
task to selectively copy files while avoiding duplicates. For instance, let's assume we have a scenario where two source directories contain duplicate files, and we want to merge them while avoiding conflicts.
task mergeFiles(type: Copy) {
from 'sourceDir1'
from 'sourceDir2'
into 'destinationDir'
duplicatesStrategy 'exclude'
}
In this example, the mergeFiles
task uses the Copy
type with the specified source directories and destination directory. The duplicatesStrategy
option is set to 'exclude'
, causing the task to exclude duplicate files when copying.
Using Gradle Build Cache to Avoid Recompilation of Duplicate Files
The Gradle Build Cache is another powerful tool for handling duplicate files, especially in the context of incremental builds. When a file is recompiled, it can introduce duplicates in the build output. By utilizing the Gradle Build Cache, the recompilation of duplicate files can be avoided, leading to faster build times and efficient resource utilization.
tasks.withType(JavaCompile) {
options.incremental = true
options.fork = true
options.forkOptions.jvmArgs << "-Xmx2g"
options.compilerArgs << "-g"
outputs.upToDateWhen { true }
}
In this example, we configure the JavaCompile
task to leverage incremental compilation and specify additional compiler options. By setting outputs.upToDateWhen { true }
, we ensure that the task is considered up to date regardless of duplicate files, thus utilizing the build cache effectively.
The Bottom Line
In conclusion, handling duplicate files in a Gradle build process is essential for ensuring resource efficiency, build stability, and application reliability. By employing strategies such as excluding duplicate files in dependencies, using Gradle's Copy
task, and leveraging the Gradle Build Cache, developers can effectively manage and resolve duplicate files in their projects.
Addressing the issue of duplicate files not only streamlines the build process but also promotes a cleaner and more maintainable codebase. As software development projects grow in complexity, mastering the art of handling duplicate files becomes an indispensable skill for every developer.
By applying the techniques discussed in this blog post, developers can mitigate the risks associated with duplicate files and optimize the Gradle build process for enhanced productivity and performance.
For further reading on Gradle build optimization and best practices, check out the Gradle documentation and Gradle Build Cache guide.
Remember, a well-managed build process is a cornerstone of successful software development, and addressing duplicate files is a crucial aspect of achieving that goal. Happy coding!