Configuring Message Driven Bean with AWS SQS in Spring Boot
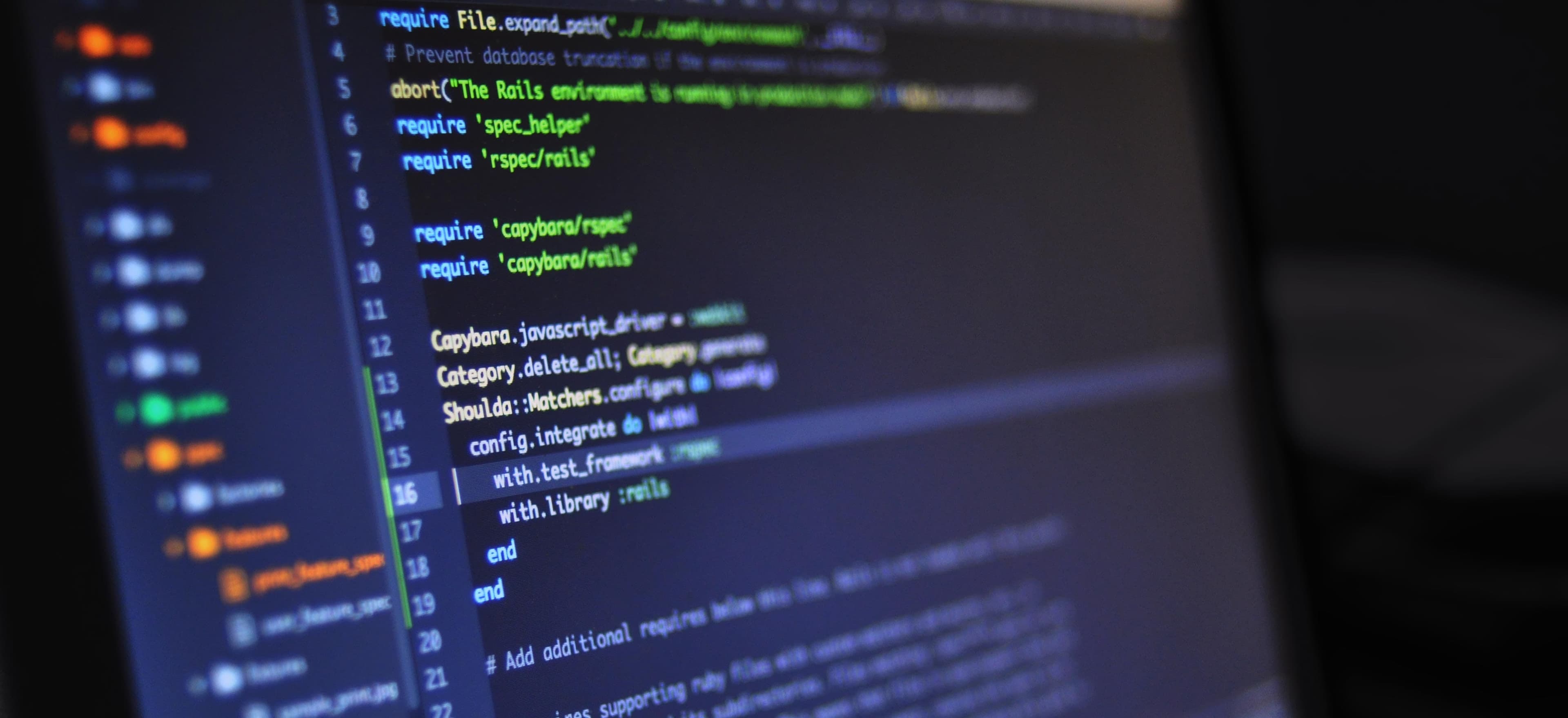
- Published on
Configuring Message Driven Bean with AWS SQS in Spring Boot
In this tutorial, we will explore how to configure a Message-Driven Bean (MDB) to consume messages from an Amazon Simple Queue Service (SQS) in a Spring Boot application. Amazon SQS is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications.
Prerequisites
- Basic understanding of Java and Spring Boot
- AWS account with access key and secret access key
- Spring Boot project set up
Setting Up AWS SQS
Before we begin, let's create an SQS queue in the AWS Management Console. Log in to your AWS account and navigate to the SQS dashboard. Click on "Create New Queue" and follow the prompts to create a Standard Queue.
Adding Dependencies
Start by adding the necessary dependencies to your Spring Boot project's pom.xml
file.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-aws</artifactId>
</dependency>
Configuring AWS Credentials
In order to interact with AWS services, you need to provide your AWS credentials to the Spring Boot application. This can be done in the application.properties
or application.yml
file.
cloud:
aws:
credentials:
accessKey: your_access_key
secretKey: your_secret_key
region:
static: your_aws_region
Replace your_access_key
, your_secret_key
, and your_aws_region
with your actual AWS credentials and region.
Creating a Message-Driven Bean
Next, create a Message-Driven Bean (MDB) to consume messages from the SQS queue. You can achieve this by using Spring's @SqsListener
annotation.
import org.springframework.cloud.aws.messaging.listener.annotation.SqsListener;
import org.springframework.stereotype.Component;
@Component
public class SqsMessageListener {
@SqsListener("your_queue_url")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
// Process the received message
}
}
Replace your_queue_url
with the URL of the SQS queue you created in the AWS Management Console.
The SqsMessageListener
class is annotated with @Component
to make it a Spring-managed bean. The @SqsListener
annotation is used to specify the queue from which messages should be consumed.
Integration with Spring Boot
To enable the message-driven bean in your Spring Boot application, you need to create a configuration class and enable the Amazon SQS listener.
import org.springframework.cloud.aws.messaging.config.annotation.EnableSqs;
@EnableSqs
@Configuration
public class SqsConfig {
// Additional configuration if needed
}
The @EnableSqs
annotation tells Spring Boot to enable listening for SQS messages in the application.
Testing the Configuration
To test the setup, start your Spring Boot application and send a message to the SQS queue using the AWS Management Console or the AWS SDK. You should see the application logging the received message as defined in the receiveMessage
method of the SqsMessageListener
.
The Bottom Line
In this tutorial, we have seen how to configure a Message-Driven Bean to consume messages from an AWS SQS queue in a Spring Boot application. This setup enables seamless integration with AWS SQS, allowing for reliable and scalable message processing within your application.
By leveraging the power of message queuing and Spring's simplicity, you can easily build robust and resilient systems that are capable of handling asynchronous messaging and distributed processing.
Remember to manage your AWS credentials securely, and consider implementing error handling and message processing strategies to handle various scenarios in a production environment.
Now that you have a solid understanding of integrating AWS SQS with Spring Boot, you can explore advanced features such as message deserialization, concurrency settings, and error handling to further enhance your application's messaging capabilities.
Happy coding!