Setting Up a Virtual Mesos Cluster Efficiently
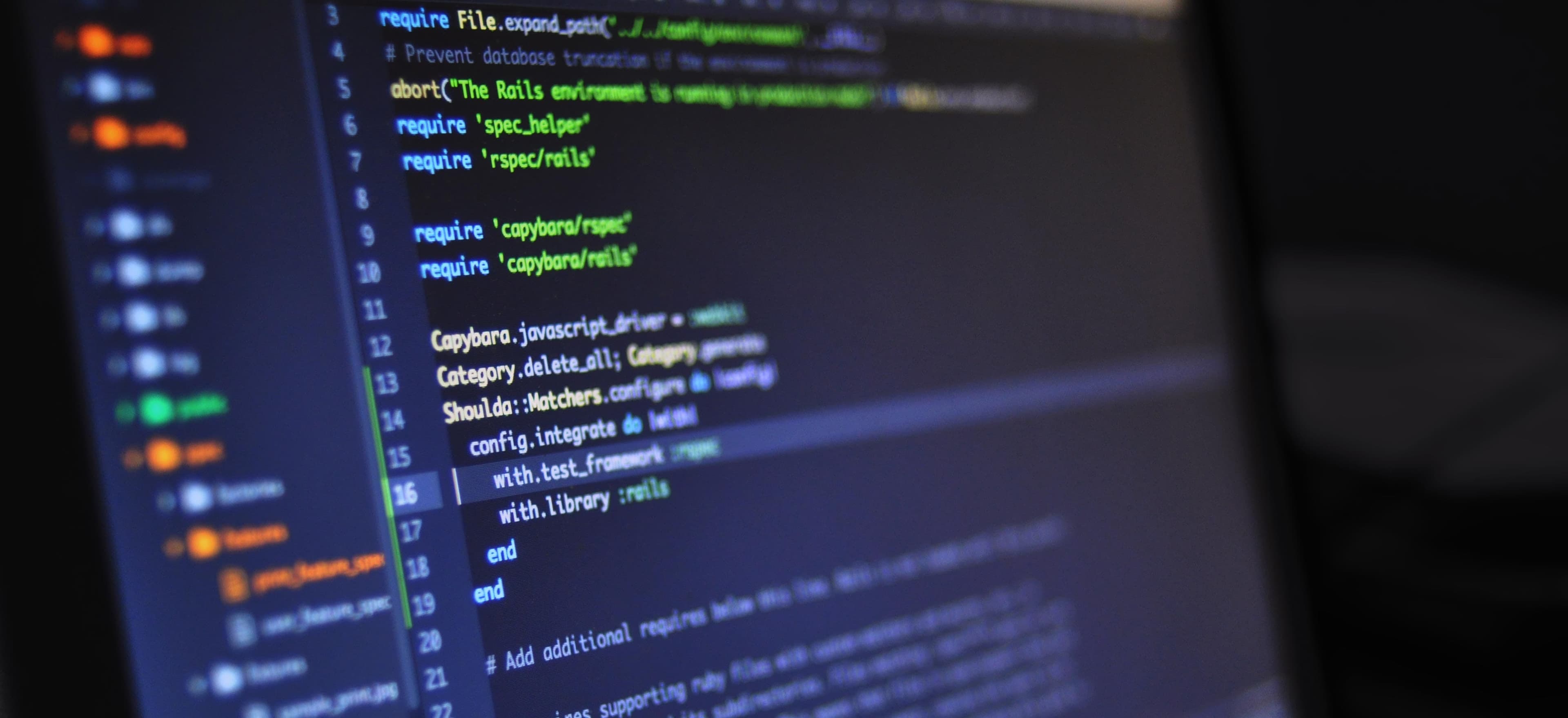
- Published on
Setting Up a Virtual Mesos Cluster Efficiently
In this post, we will walk through setting up a virtual Mesos cluster efficiently using Java. Mesos is a powerful distributed systems kernel that provides resources such as CPU, memory, and storage to applications across a cluster of machines. This post assumes some familiarity with Mesos concepts, but even if you are new to Mesos, you will find this guide clear and helpful.
Prerequisites
Before we dive into setting up a virtual Mesos cluster, ensure you have the following installed and set up:
- Java Development Kit (JDK) - Mesos is written in C++, but we can interact with it via the Mesos Java API.
- VirtualBox or any other virtualization software of your choice.
- Vagrant - Vagrant provides easy-to-configure, reproducible, and portable work environments.
Setting Up Vagrant
First, let's create a directory for our Mesos cluster, navigate to it, and run vagrant init
to create a Vagrantfile
. We will use the official Ubuntu Xenial64 box:
mkdir mesos-cluster
cd mesos-cluster
vagrant init ubuntu/xenial64
Configuring the Vagrantfile
Open the Vagrantfile
in your preferred text editor and modify it to include the following provision for setting up a Mesos master and slaves:
Vagrant.configure("2") do |config|
config.vm.box = "ubuntu/xenial64"
# Setting up a Mesos Master
config.vm.define "mesos-master" do |master|
master.vm.network "private_network", ip: "192.168.33.10"
master.vm.provision "shell", path: "provision/master.sh"
end
# Setting up a Mesos Slave
(1..2).each do |i|
config.vm.define "mesos-slave-#{i}" do |slave|
slave.vm.network "private_network", ip: "192.168.33.1#{i + 10}"
slave.vm.provision "shell", path: "provision/slave.sh"
end
end
end
Installing Java on Mesos Nodes
Create a provision
directory and within it, create master.sh
and slave.sh
files. In each file, install Java using the following commands:
# master.sh
#!/bin/bash
apt-get update
apt-get install -y default-jdk
# slave.sh
#!/bin/bash
apt-get update
apt-get install -y default-jdk
Mesos Java API
Now, let's write a simple Java application that interacts with our Mesos cluster. We will be using the Mesos Java API to connect to the Mesos cluster, submit a sample task, and receive task status updates.
Connecting to the Mesos Cluster
To connect to the Mesos cluster, we need to create a MesosSchedulerDriver
and pass it our scheduler implementation:
import org.apache.mesos.MesosSchedulerDriver;
import org.apache.mesos.Protos.FrameworkInfo;
public class MesosJavaApp {
public static void main(String[] args) {
// Initialize your scheduler implementation (omitted for brevity)
MesosScheduler scheduler = new MesosScheduler();
FrameworkInfo frameworkInfo = FrameworkInfo.newBuilder()
.setName("Mesos-Java-App")
.setUser("") // Insert your username here
.setFailoverTimeout(120) // Set an appropriate failover timeout
.setCheckpoint(true)
.build();
MesosSchedulerDriver driver = new MesosSchedulerDriver(scheduler, frameworkInfo, "YOUR_MESOS_MASTER_IP:5050");
int status = driver.run() == Protos.Status.DRIVER_STOPPED ? 0 : 1;
driver.stop();
System.exit(status);
}
}
Submitting a Sample Task
Assuming we have a MesosScheduler
implementation, we can submit a sample task as follows:
import org.apache.mesos.Protos.CommandInfo;
import org.apache.mesos.Protos.ContainerInfo;
import org.apache.mesos.Protos.ExecutorInfo;
import org.apache.mesos.Protos.TaskInfo;
public class MesosScheduler implements Scheduler {
// Other overridden methods (omitted for brevity)
public void submitTask() {
TaskInfo task = TaskInfo.newBuilder()
.setName("Sample-Task")
.setTaskId(TaskID.newBuilder().setValue("task1"))
.setSlaveId(SlaveID.newBuilder().setValue("slave1"))
.setCommand(CommandInfo.newBuilder()
.setValue("echo 'Hello, Mesos!'")
).build();
driver.launchTasks(Collections.singletonList(task));
}
}
Receiving Task Status Updates
We can handle task status updates in our scheduler implementation:
import org.apache.mesos.SchedulerDriver;
public class MesosScheduler implements Scheduler {
// Other overridden methods (omitted for brevity)
public void statusUpdate(ExecutorDriver driver, TaskStatus status) {
System.out.println("Task " + status.getTaskId().getValue() + " is in state " + status.getState());
}
}
A Final Look
In this post, we've explored setting up a virtual Mesos cluster efficiently using Vagrant, configuring nodes, and writing a Java application to interact with the cluster using the Mesos Java API. Mesos provides a powerful platform for developing and running distributed systems, and interacting with it using Java opens up a wide range of possibilities for building robust and scalable applications.
By following the steps outlined in this post, you can quickly get started with experimenting and developing applications on a Mesos cluster, sharpening your skills in distributed systems and cluster management.
Now that you have a functional Mesos cluster and a basic Java application interacting with it, you can further explore Mesos capabilities, experiment with different types of tasks and frameworks, and delve into more advanced Mesos features to harness the full potential of distributed computing. Happy clustering!
Remember to refer to the official Mesos documentation for in-depth details on Mesos concepts, API, and best practices.
Happy coding!