Managing Data Consistency in Event Sourced CQRS Read Model
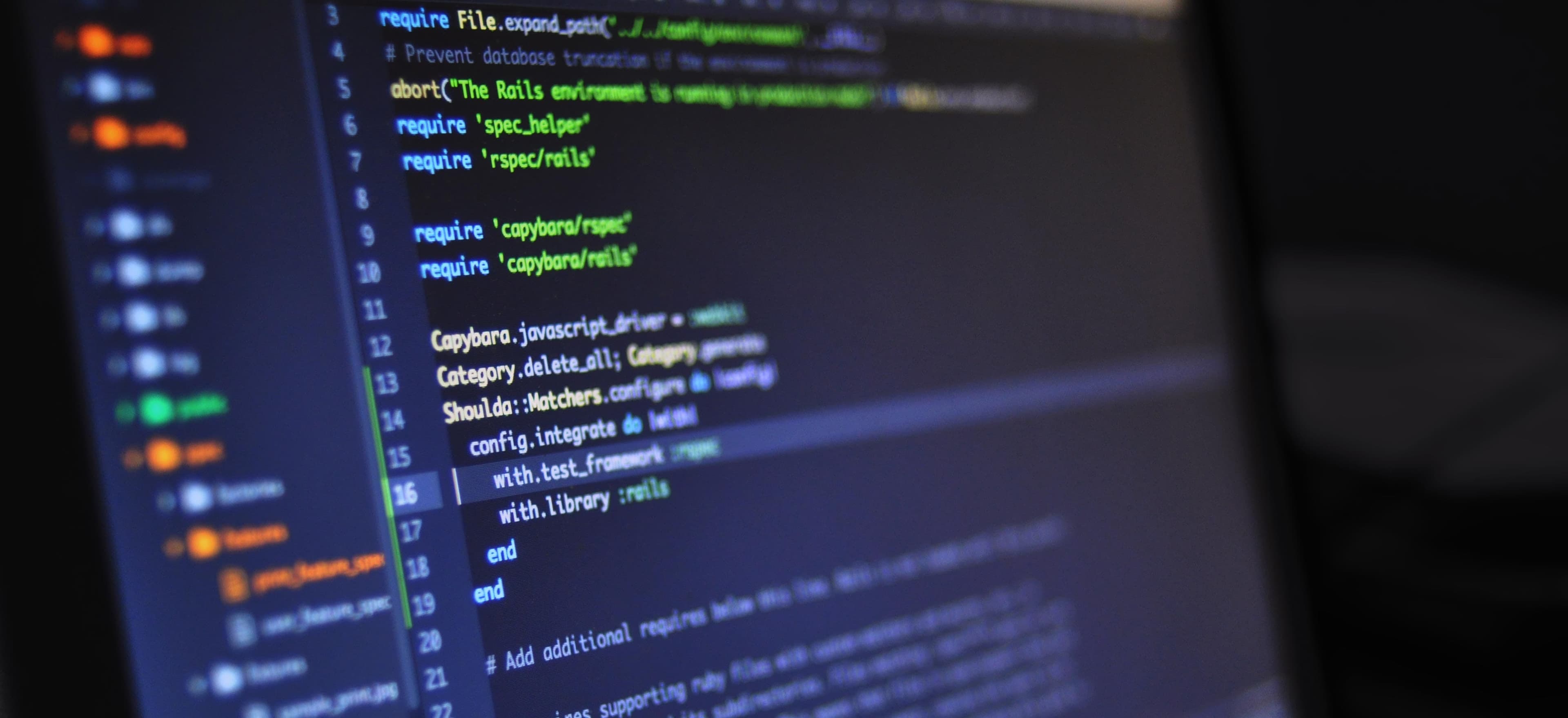
- Published on
Managing Data Consistency in Event Sourced CQRS Read Model
In the world of event sourcing and CQRS (Command Query Responsibility Segregation), ensuring data consistency across the read model can be quite challenging. Event sourcing involves capturing all changes to an application state as a sequence of events, while CQRS separates the responsibility of handling command (write) and query (read) operations.
In this blog post, we'll delve into the strategies and best practices for managing data consistency in event-sourced CQRS read models using Java.
Understanding the Challenge
When a command is executed and events are generated, the read model needs to be updated to reflect the latest state of the application. However, asynchronous processing of events and the potential for failures can lead to inconsistencies between the write and read models.
Applying Eventual Consistency
In the CQRS architecture, it's important to embrace eventual consistency. This means that the read model might not always be in sync with the write model immediately after a command is executed. Instead, it will eventually converge to a consistent state as events are processed.
Event Handlers and Projections
In Java, event handlers are responsible for consuming domain events and updating the read model. Projections represent the denormalized view of the data and are constructed by applying events in the correct order.
Let's consider an example of an event handler that updates the read model when a ProductCreatedEvent
is received:
@EventHandler
public void on(ProductCreatedEvent event) {
ProductView productView = new ProductView(event.getProductId(), event.getName(), event.getPrice());
// Update the read model with the new product
productViewRepository.save(productView);
}
The ProductView
serves as a denormalized representation of the product and is stored in the read model database.
Handling Eventual Consistency
In a distributed system, achieving perfect consistency at all times is impractical. Instead, we need to handle eventual consistency gracefully. This involves informing users that the read model might not immediately reflect the latest changes.
Optimistic Concurrency Control
One way to address data consistency is to use optimistic concurrency control. When updating the read model, we can include a version number for each entity and use it to ensure that updates are applied in the correct sequence.
@EventHandler
public void on(ProductPriceUpdatedEvent event) {
ProductView productView = productViewRepository.findById(event.getProductId());
if (productView.getVersion() == event.getExpectedVersion()) {
productView.setPrice(event.getNewPrice());
productView.setVersion(productView.getVersion() + 1);
productViewRepository.save(productView);
} else {
// Handle concurrency conflict
}
}
In this example, the expectedVersion
in the event ensures that the update is applied only if the read model is at the expected version.
Compensation Mechanisms
In scenarios where the read model update fails or encounters errors, it's crucial to have compensation mechanisms in place. These mechanisms might include retry policies, error handling strategies, or even human intervention processes to reconcile the inconsistencies.
Event Replay and Rebuilding Read Models
In some cases, the read model might diverge significantly from the write model due to errors or data corruption. Event replay and rebuilding the read model from scratch can help recover from such scenarios.
Testing for Data Consistency
Unit tests and integration tests should be in place to validate the correctness and consistency of the read model updates. Mocking event sources and ensuring that the read model reflects the expected state after processing events is crucial for maintaining data consistency.
A Final Look
Managing data consistency in event-sourced CQRS read models requires a careful balance of embracing eventual consistency, using concurrency control mechanisms, implementing compensation strategies, and testing rigorously. By applying these strategies, we can ensure that the read model accurately reflects the application state despite the asynchronous nature of event processing.
To delve deeper into the world of event sourcing and CQRS, check out this comprehensive guide on Microservices and CQRS. Additionally, for a hands-on approach to event sourcing and CQRS in Java, explore the Axon Framework, a powerful toolkit for building event-driven microservices.
Happy coding!