Debugging Tips for JUnit 5 Test Failures
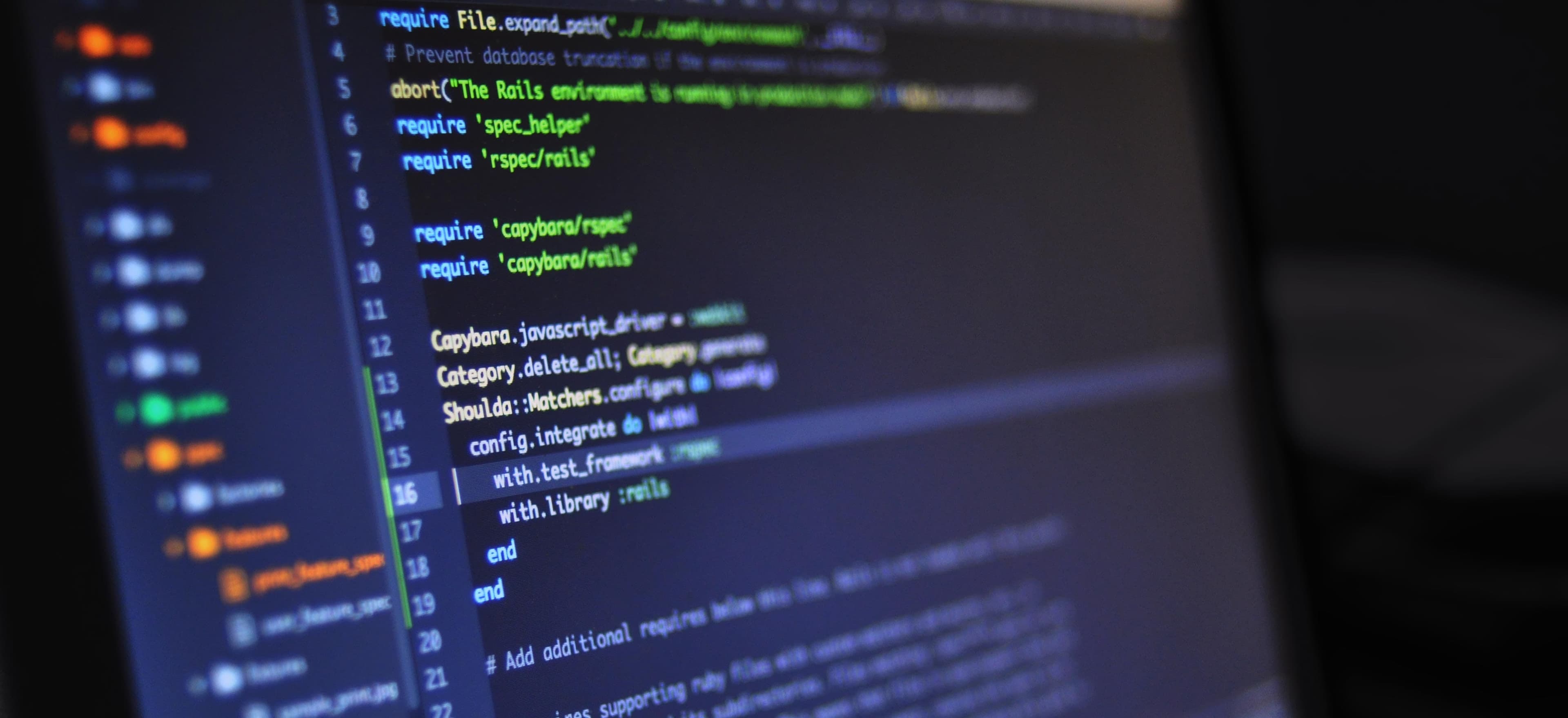
- Published on
Debugging Tips for JUnit 5 Test Failures
When writing unit tests in Java, JUnit is a popular choice for ensuring that your code works as intended. However, dealing with failing tests can be a frustrating experience. In this post, we will explore some helpful tips for debugging JUnit 5 test failures to streamline the troubleshooting process and save valuable time.
1. Read the Error Message Carefully
When a test fails, the first step in debugging is to carefully read the error message provided by JUnit. This message often provides crucial information about what went wrong. Understanding the error message can guide you in identifying the root cause of the failure.
2. Verify Test Preconditions
Before diving into the test code, ensure that the test prerequisites are met. This includes verifying that any required resources, such as databases or external services, are available and correctly configured for the test to run successfully.
3. Use System.out.println for Debugging
One of the simplest yet effective ways to debug failing tests is by strategically placing System.out.println
statements within the test code. This allows you to inspect the state of variables and the flow of the test execution.
Here's an example of using System.out.println
for debugging purposes:
@Test
void testWithSystemOutPrintln() {
int result = someOperation(3, 4);
System.out.println("Result: " + result);
assertEquals(7, result);
}
In this example, the System.out.println
statement helps to print the result
before the assertion, aiding in understanding the test outcome.
4. Leverage Assertions
JUnit provides a wide range of assertion methods that can be instrumental in debugging test failures. When an assertion fails, it provides valuable insights into the actual and expected values, enabling you to identify discrepancies in the test outcome.
For example, consider the following assertion using JUnit's assertEquals
method:
@Test
void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 2);
assertEquals(4, result, "Addition of 2 and 2 should equal 4");
}
In this case, if the test fails, the assertion message will be displayed, aiding in pinpointing the issue with the addition operation.
5. Employ Conditional Breakpoints
Utilizing conditional breakpoints in your IDE, such as IntelliJ IDEA or Eclipse, can be immensely helpful in debugging JUnit tests. By setting breakpoints with conditions based on certain variables or expressions, you can halt the test execution at critical points and inspect the state of the program.
6. Check for Side Effects
Sometimes, a failed test may not be the direct result of the tested method but rather due to side effects from other parts of the code. It's important to examine the test environment and look for any unexpected interactions that could be affecting the test outcome.
7. Review Test Input Data
Carefully reviewing the input data provided to the test is essential. Ensure that the input values used in the test cover a comprehensive range of scenarios and edge cases. Inadequate test data can lead to overlooked issues that only surface during real-world usage.
8. Utilize Logging
Integrating logging statements within the test code can provide valuable insights into the test execution flow and the values of variables. Logging frameworks like Log4j or SLF4J can be used to capture detailed information during test runs.
Here's an example of incorporating logging using SLF4J:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyTest {
private static final Logger logger = LoggerFactory.getLogger(MyTest.class);
@Test
void testWithLogging() {
logger.debug("Executing testWithLogging");
// Test logic
}
}
By examining the logs, you can gain a deeper understanding of the test behavior and identify any anomalies.
9. Use Parameterized Tests
In scenarios where a test fails with certain inputs, parameterized tests can be leveraged to explore different input combinations without writing separate test methods.
With JUnit 5's @ParameterizedTest
and @CsvSource
, you can efficiently test multiple input parameters, as demonstrated in the following code snippet:
@ParameterizedTest
@CsvSource({ "1, 1, 2", "2, 3, 5", "3, 5, 8" })
void testAddition(int a, int b, int sum) {
assertEquals(sum, calculator.add(a, b), () -> a + " + " + b + " should equal " + sum);
}
By using parameterized tests, you can systematically evaluate various input scenarios, potentially uncovering the specific conditions causing the test failures.
10. Review Exception Handling
If a test involves expected exceptions, ensure that the exception assertions are correctly configured. Verify that the right exceptions are being thrown and caught as per the test requirements.
In conclusion, navigating JUnit 5 test failures requires a combination of attention to detail, strategic debugging tactics, and leveraging the features provided by the framework. By applying the tips outlined in this post, you can effectively approach and resolve test failures with confidence.
Remember, mastering the art of debugging tests not only strengthens the reliability of your codebase but also enhances your proficiency as a Java developer.
Happy debugging!
References: