Choosing the Right Java Micro Framework: A Definitive Guide
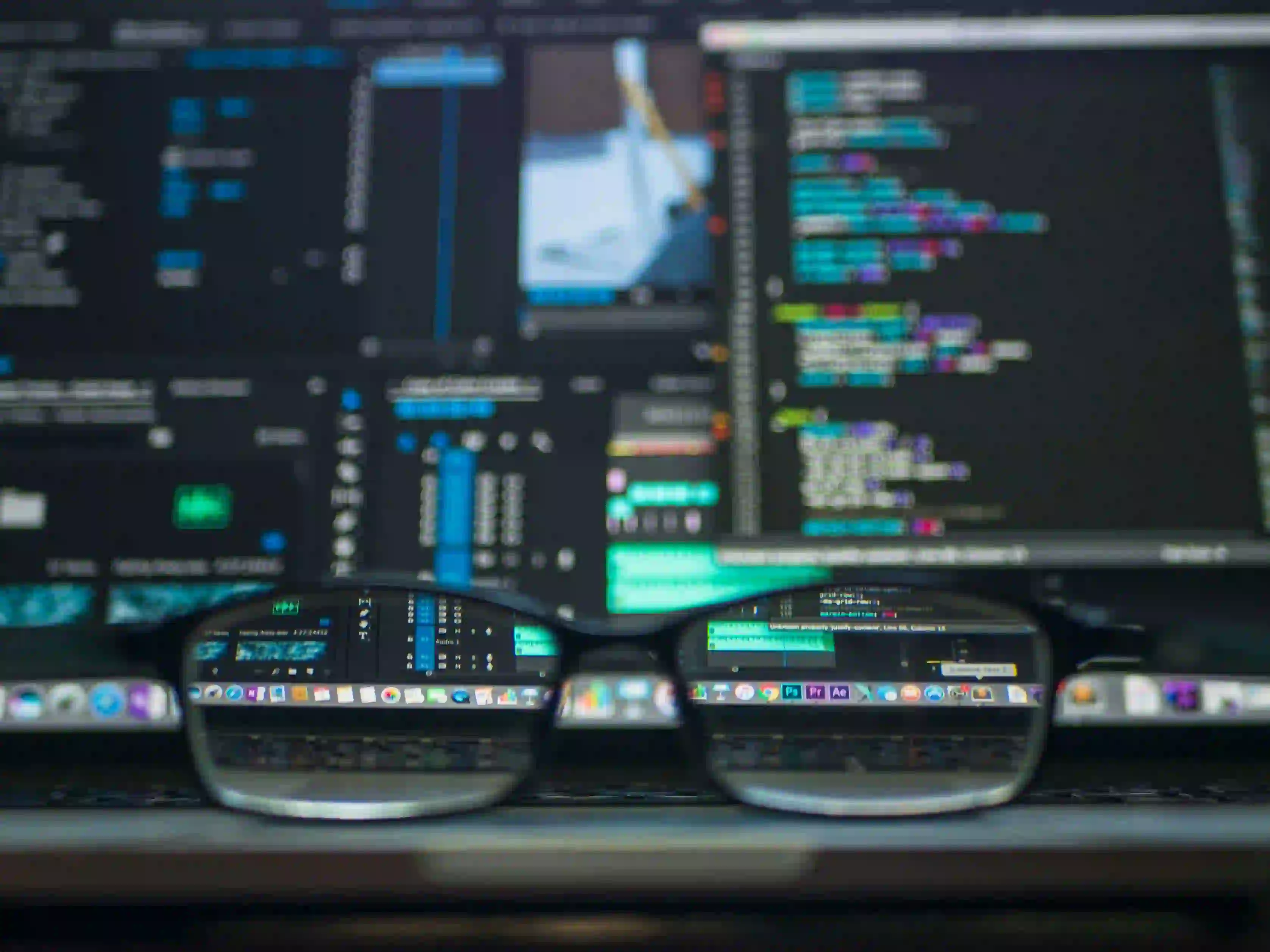
Choosing the Right Java Micro Framework: A Definitive Guide
If you are a Java developer, you might find yourself at a crossroads when it comes to choosing the right micro framework for your next project. With an array of options available, each with its own set of features and capabilities, making the right choice can be a daunting task. In this guide, we will take a deep dive into the world of Java micro frameworks, compare some of the most popular options, and help you make an informed decision.
Why Choose a Java Micro Framework?
Before we delve into the specifics of each framework, let's first understand why Java micro frameworks are gaining traction in the development community. Java micro frameworks are designed to be lightweight, offering just enough functionality to build web applications without the complexities of a full-fledged framework like Spring or Java EE. They promote rapid development, simple deployment, and are well-suited for building microservices and RESTful APIs.
Popular Java Micro Frameworks
1. Spark Framework
Spark is a micro framework for creating web applications in Kotlin and Java 8. It provides a simple and expressive syntax, making it a favorite among developers who value clean and concise code. Spark is known for its ease of use and is a great choice for building RESTful APIs and small to medium web applications.
Why Choose Spark?
- Easy to get started with
- Clear and intuitive syntax
- Great for building RESTful services
// Example route using Spark
import static spark.Spark.*;
public class HelloWorld {
public static void main(String[] args) {
get("/hello", (req, res) -> "Hello World");
}
}
2. Javalin
Javalin is a lightweight web framework for Java and Kotlin that is inspired by Spark and Ktor. It is known for its simplicity, ease of use, and focus on creating modern, high-performance web applications. Javalin provides a simple and expressive API and is suitable for building microservices and RESTful APIs.
Why Choose Javalin?
- Modern and lightweight
- Built-in support for WebSockets
- Focus on simplicity and ease of use
// Example Javalin app
import io.javalin.Javalin;
public class HelloWorld {
public static void main(String[] args) {
Javalin app = Javalin.create().start(7000);
app.get("/", ctx -> ctx.result("Hello World"));
}
}
3. Micronaut
Micronaut is a modern, JVM-based framework designed for building modular, easily testable microservice and serverless applications. It boasts minimal startup time and memory usage, making it ideal for cloud-native applications. Micronaut provides features like dependency injection, AOP, and ahead-of-time (AOT) compilation for superior performance.
Why Choose Micronaut?
- Low memory footprint and fast startup time
- Built-in support for GraalVM and Kotlin
- Ideal for cloud-native and serverless applications
// Example Micronaut controller
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
@Controller("/hello")
public class HelloController {
@Get("/")
public String index() {
return "Hello World";
}
}
How to Choose the Right Framework
When choosing the right Java micro framework for your project, consider the following factors:
1. Project Requirements
Understand the specific requirements of your project. Are you building a small RESTful API, a microservice, or a larger web application? Each framework caters to different use cases, so aligning the project requirements with the strengths of the framework is crucial.
2. Performance and Scalability
Consider the performance and scalability needs of your application. Some frameworks, like Micronaut, are optimized for high performance and low memory usage, making them suitable for resource-constrained environments.
3. Community and Support
Evaluate the community and support behind each framework. A thriving community means better documentation, more third-party libraries, and active development. This support can be instrumental in overcoming challenges during development.
4. Integration and Ecosystem
Consider the integration capabilities and ecosystem of the framework. Does it seamlessly integrate with other tools and libraries that you intend to use in your project?
5. Learning Curve
Assess the learning curve associated with each framework. Choose a framework that aligns with the expertise of your development team to minimize onboarding time and ensure productivity from the start.
The Closing Argument
In conclusion, choosing the right Java micro framework is a critical decision that can impact the success of your project. Each framework has its own strengths and ideal use cases. By understanding the key factors discussed in this guide and evaluating them in the context of your project, you can make an informed decision that will set you on the path to building efficient and scalable Java applications.
Now armed with the knowledge of Java micro frameworks, it's time to take the next step and start crafting your next project with the perfect framework in tow. Happy coding!
Remember, the right Java micro framework brings ease, simplicity, and speed to your development process.
DISCLAIMER: The code snippets provided in this guide are intended for illustrative purposes only and may not represent production-ready code. Always consider best practices and security measures when implementing code in a production environment.
Resources:
Remember, it's not just about choosing a framework; it's about choosing the right framework for your project. Stay informed, stay updated, and may your code always run smoothly!