Overcoming Testcontainers Setup Challenges in Complex Projects
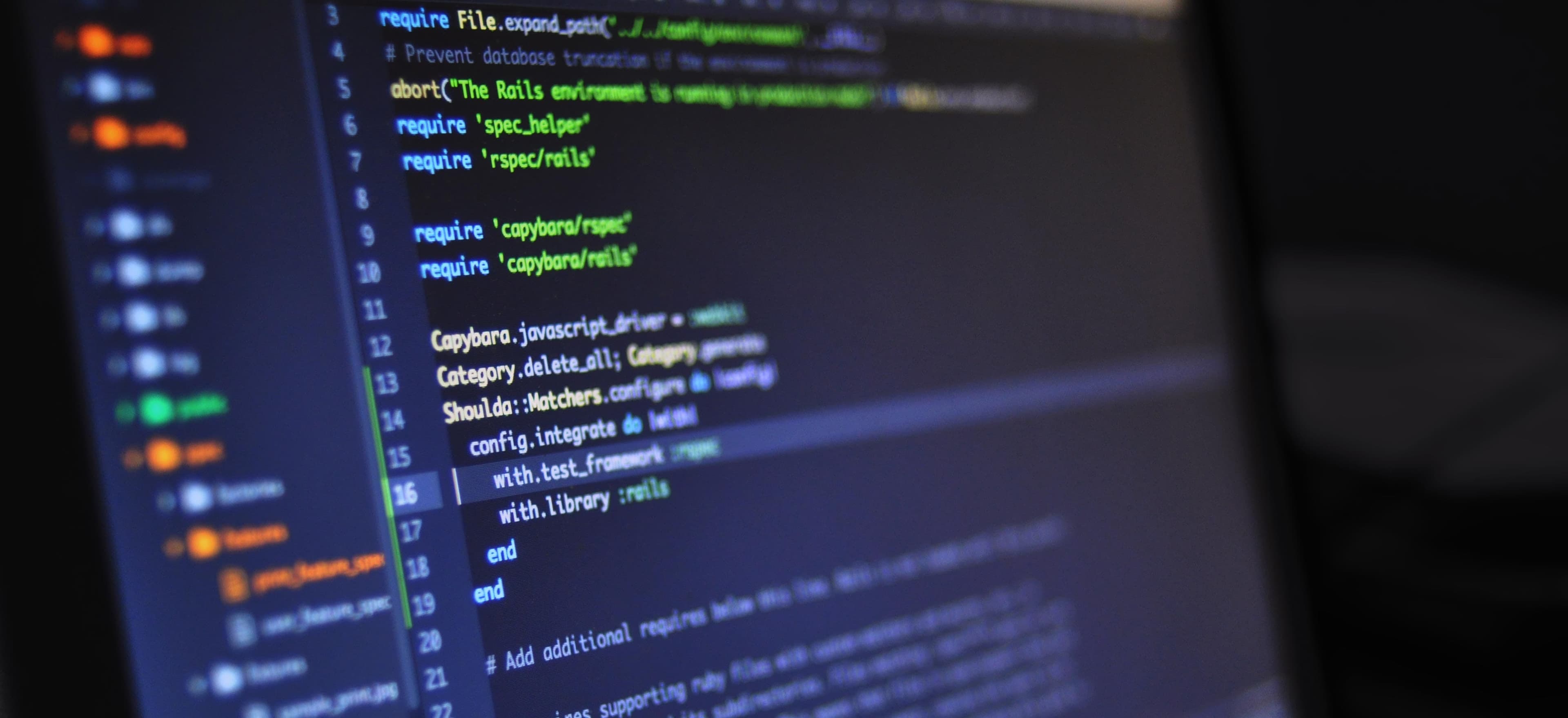
- Published on
Overcoming Testcontainers Setup Challenges in Complex Projects
In modern application development, ensuring robust testing practices is pivotal. One of the leading tools taking center stage in this arena is Testcontainers. This library allows developers to work with Docker containers in their tests, providing a consistent and isolation-friendly environment to run tests against various dependencies like databases, message queues, or web servers. However, setting up Testcontainers in complex projects can present a unique set of challenges. In this blog post, we will explore practical solutions to these challenges, share valuable code snippets, and offer insights into why they work.
What is Testcontainers?
Before diving into the challenges, let's clarify what Testcontainers is. Testcontainers is a Java library that provides lightweight, throwaway instances of common databases, message brokers, and anything else that can run in a Docker container. With Testcontainers, you can run tests in an isolated environment, ensuring that the results are reproducible.
Common Challenges with Testcontainers
- Docker Dependency
- Resource Management
- Configuration Complexity
- Debugging Issues
- Performance
Let’s explore these challenges in detail and how to overcome them.
1. Docker Dependency
Challenge
One of the primary hurdles is the dependency on Docker. If Docker is not set up properly on your development machine or CI environment, running tests can fail unexpectedly.
Solution
Ensure Docker is correctly installed and configured. Provide clear instructions in your project’s documentation to facilitate proper setup.
Here’s how to check if Docker can be accessed:
docker --version
If Docker is installed correctly, this command returns the installed Docker version. Set up environment variables for configuring Docker, especially in CI/CD pipelines.
2. Resource Management
Challenge
Each test case that uses Testcontainers spins up a new container. This can consume significant system resources, potentially impacting the application's performance during testing.
Solution
Reusing Containers By reusing Testcontainers instances across tests, we can mitigate resource consumption.
The following code snippet demonstrates how to use singleton containers throughout your tests:
public class MyDatabaseTest {
private static PostgreSQLContainer<?> postgreSQLContainer = new PostgreSQLContainer<>("postgres:latest");
static {
postgreSQLContainer.start();
}
@Test
public void sampleTest() {
String jdbcUrl = postgreSQLContainer.getJdbcUrl();
// Your test code here
}
}
Why Reuse? Reusing containers reduces overhead from starting and stopping, which can be particularly beneficial in CI environments where resource conservation is necessary.
3. Configuration Complexity
Challenge
Complex projects often have intricate configurations that can be cumbersome to replicate within Testcontainers.
Solution
Utilize environment variables for your configuration or a dedicated test configuration file. This way, you can manage configurations more seamlessly.
public class ConfigurableContainerTest {
private static final String DBImage = System.getenv("DB_IMAGE"); // e.g., "postgres:latest"
@Container
private static PostgreSQLContainer<?> container = new PostgreSQLContainer<>(DBImage)
.withDatabaseName("test_db")
.withUsername("user")
.withPassword("password");
}
Why This Works? Using environment variables allows you to adapt to different environments easily. It enhances the configurability of your project, making it more manageable in diverse setups.
4. Debugging Issues
Challenge
Debugging tests that run in containers can introduce complexity since the stack traces might not be as informative as those in traditional testing scenarios.
Solution
Enable logging within your containers. Standalone database containers might not output logs to your test framework.
public class LoggingContainerTest {
@Container
private static PostgreSQLContainer<?> postgreSQLContainer = new PostgreSQLContainer<>("postgres:latest")
.withCommand("postgres -c log_statement=all"); // Enable all logs
@Test
public void loggingTest() {
// Log output can be monitored
System.out.println(postgreSQLContainer.getLogs());
}
}
Why Enable Logging? Enabling detailed logging ensures that any issues during the test process are documented, providing clear insight into the underlying problem per container usage.
5. Performance
Challenge
Tests can run substantially slower because of container overhead. This can frustrate developers, especially in situations where rapid iteration is key to productivity.
Solution
Parallel Execution Execute tests in parallel wherever possible. Testcontainers supports that natively, and you can enable parallel execution using TestNG or JUnit.
@Ifenv("test.parallel.enabled")
public class ParallelContainerTest {
@Container
private static final PostgreSQLContainer<?> postgreSQLContainer = new PostgreSQLContainer<>("postgres:latest");
@Test
public void firstTest() {
// Test logic here
}
@Test
public void secondTest() {
// Test logic here
}
}
Why Parallel Test Execution? It significantly reduces overall build time by leveraging concurrent execution, which is especially beneficial for large test suites running in CI environments.
The Closing Argument
Using Testcontainers in complex projects can streamline testing immensely, however, it’s essential to acknowledge and work through the challenges presented. By tackling Docker dependencies, managing resources effectively, maintaining flexible configurations, logging for better debugging, and optimizing performance through parallel execution, you can enhance your testing workflows significantly.
For anyone looking to leverage Testcontainers further, be sure to check out the official Testcontainers documentation for advanced topics and community examples. Embracing these practices will not only enhance the quality of your tests but will also improve the overall development experience.
Happy testing!