Troubleshooting ActorPath Resolution
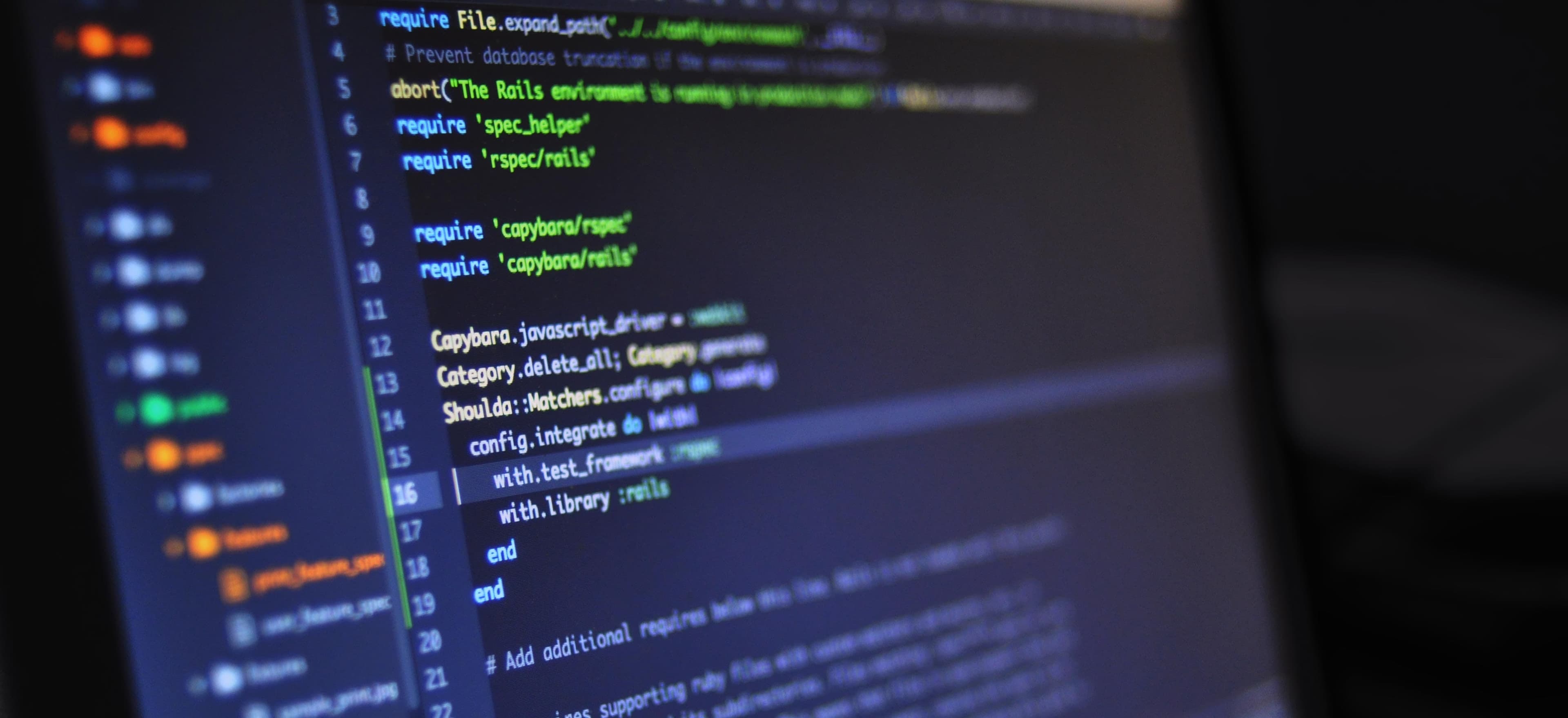
- Published on
Troubleshooting ActorPath Resolution in Akka
Akka is a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications using the Actor model. In the world of Akka, actors are the building blocks for creating scalable and resilient applications. Actors communicate with each other by sending and receiving messages. Each actor has an address, or ActorPath
, which uniquely identifies it within the actor system. However, there are scenarios where resolving and interacting with actor paths can be tricky. In this blog post, we'll delve into common issues related to actor path resolution in Akka and explore how to troubleshoot them effectively.
Understanding ActorPaths
Before diving into troubleshooting, let's start by understanding what an ActorPath is and why it's crucial in Akka. An ActorPath uniquely identifies an actor within the actor system and contains the system's address and the actor's path. For example, an actor path might look like akka://my-system/user/my-actor
. This path consists of the protocol (akka
), the system name (my-system
), and the actor's path relative to the root of the actor tree (user/my-actor
).
Common Issues
Issue 1: Invalid ActorPath
One common issue that developers encounter is dealing with an invalid actor path. This can occur when constructing actor paths manually or when receiving actor paths from external sources like configuration files or user input. Invalid actor paths can lead to runtime errors, making it crucial to validate actor paths before using them.
Troubleshooting Tip:
- Use the
ActorPath.isValidPath
method to validate actor paths programmatically. - If actor paths are retrieved from external sources, consider implementing validation logic to ensure their correctness.
Issue 2: Resolving ActorPath
Another common issue arises when resolving actor paths to interact with specific actors within the actor system. Incorrectly resolved actor paths can result in actors not being found or unintended actors being targeted.
Troubleshooting Tip:
- Double-check the actor system's hierarchy and the path to the target actor.
- Use the
ActorSystem.actorSelection
method to resolve actor paths dynamically. This method returns anActorSelection
object, allowing messages to be sent to the selected actor based on its path.
Issue 3: Remote ActorPath Resolution
In a distributed Akka system, interacting with actors across different nodes introduces complexities in resolving remote actor paths. Issues related to network configuration, actor system naming, and node discovery can impact the resolution of remote actor paths.
Troubleshooting Tip:
- Ensure that network settings and remote actor system configurations are correctly set up.
- Use tools like Akka's Cluster API to facilitate node discovery and cluster management for seamless remote actor path resolution.
Best Practices
To avoid and effectively troubleshoot actor path resolution issues, consider the following best practices:
-
Use ActorRefs: Whenever possible, interact with actors using
ActorRef
rather thanActorPath
. ActorRefs provide type safety and encapsulate actor references, reducing the likelihood of path resolution errors. -
Centralize ActorPath Construction: Centralize the construction of actor paths to avoid inconsistencies and reduce the risk of invalid paths. Consider using factory methods or constants for constructing actor paths.
-
Logging and Monitoring: Implement logging and monitoring to track actor path resolution activities. This can aid in identifying issues and understanding the flow of actor interactions within the system.
-
Unit Testing ActorPath Resolution: Write unit tests specifically targeting actor path resolution to ensure that paths are resolved correctly under various scenarios. Use mock objects or test actors to isolate path resolution logic.
Example Code
Let's look at an example of how to resolve an actor path using ActorSystem.actorSelection
:
import akka.actor.ActorRef;
import akka.actor.ActorSelection;
import akka.actor.ActorSystem;
public class ActorPathResolutionExample {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("my-system");
// Resolve actor path using actorSelection
ActorSelection selection = system.actorSelection("/user/my-actor");
// Use the resolved selection to send a message
selection.tell("Hello", ActorRef.noSender());
}
}
In this example, we create an ActorSystem
and resolve the actor path /user/my-actor
using actorSelection
. We then use the resolved ActorSelection
to send a "Hello" message to the targeted actor.
In Conclusion, Here is What Matters
Troubleshooting actor path resolution in Akka is a critical aspect of building robust and reliable actor-based systems. By understanding common issues, following best practices, and leveraging the appropriate tools and techniques, developers can effectively tackle challenges related to actor path resolution. Remember to validate actor paths, carefully resolve actor paths, and consider the complexities of remote actor path resolution in distributed environments. With a solid understanding of actor paths and the tools available for resolution, developers can ensure smooth and efficient actor interactions within their Akka applications.
For further insights and detailed documentation, refer to Akka's official documentation on ActorPaths and ActorSelection. Also, check out the Akka Cluster documentation for guidance on managing distributed actor systems.