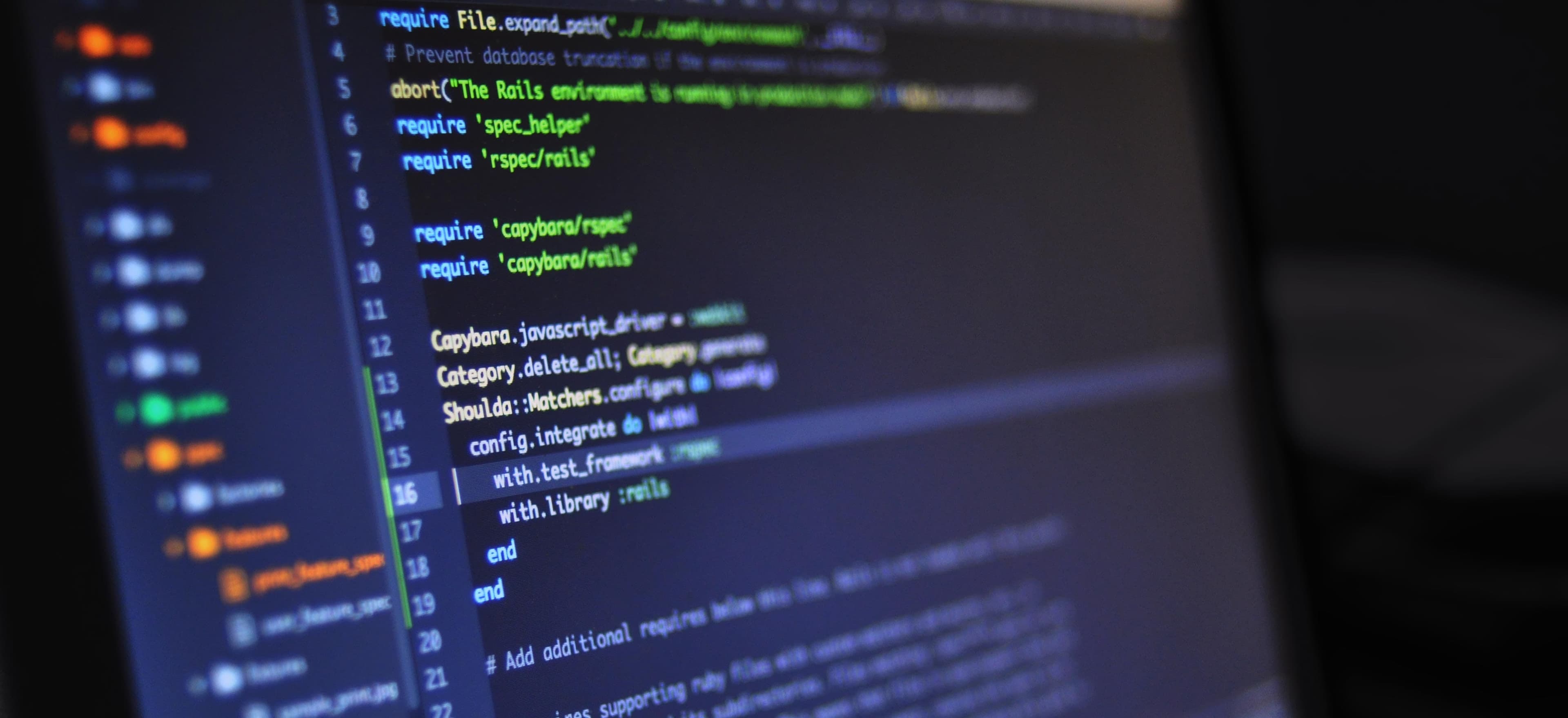
- Published on
Understanding @BeanParam in JAX-RS 2.0
In the world of Java web development, JAX-RS (Java API for RESTful Web Services) is a powerful and widely-used framework for building RESTful web services. It provides a range of annotations and features to simplify the development of web APIs. One of these features is the @BeanParam
annotation, which can be used to streamline and organize the handling of request parameters in JAX-RS resource classes.
What is @BeanParam?
The @BeanParam
annotation is used in JAX-RS 2.0 to inject all the components of a request into a JavaBean parameter. This can be particularly useful when a resource method needs to access multiple query parameters, form parameters, and HTTP header values. By using @BeanParam
, you can create a single parameter object that encapsulates all of these components, making your resource classes cleaner and more maintainable.
How to Use @BeanParam
Using @BeanParam
is quite straightforward. First, you need to create a JavaBean class that contains fields corresponding to the individual components of the request that you want to access. For example, if you want to capture query parameters and a header value, you might create a class like this:
public class RequestParams {
@QueryParam("param1")
private String param1;
@QueryParam("param2")
private int param2;
@HeaderParam("Authorization")
private String authorization;
// Getters and setters
}
In this example, the RequestParams
class encapsulates two query parameters (param1
and param2
) and one HTTP header (Authorization
). It's important to provide appropriate getter and setter methods for these fields in accordance with standard JavaBean conventions.
Once you have defined your parameter class, you can use it with the @BeanParam
annotation in your JAX-RS resource method:
@GET
@Path("/resource")
public Response getResource(@BeanParam RequestParams params) {
// Access params.getParam1(), params.getParam2(), params.getAuthorization() here
// Process the request and return a response
}
In this example, the getResource
method uses @BeanParam
to inject the RequestParams
object, which contains all the components of the request that were specified in the RequestParams
class. This allows you to access and use these components directly within the method.
Why Use @BeanParam?
The use of @BeanParam
offers several benefits when working with JAX-RS resources.
-
Code Organization: By encapsulating related request components in a single parameter object, your resource classes become more organized and easier to maintain. This can be especially helpful when dealing with complex requests that involve multiple parameters and headers.
-
Reusability: Once you have defined a parameter class for a particular type of request, you can easily reuse it across multiple resource methods. This promotes code reuse and can save you from writing repetitive parameter-handling code.
-
Clarity: Using
@BeanParam
makes your resource methods more explicit about the parameters they expect, as the parameter class serves as a clear contract for the expected request components.
When to Use @BeanParam
While @BeanParam
can be a powerful tool, it is not always necessary to use it for every request parameter in your JAX-RS resource classes. It's best suited for situations where you need to handle multiple related parameters or headers, or when you want to improve the organization and clarity of your code. For simple cases involving only one or two parameters, using the individual @QueryParam
or @HeaderParam
annotations directly in the method signature may be more appropriate.
The Bottom Line
In conclusion, the @BeanParam
annotation in JAX-RS 2.0 is a valuable feature for simplifying the handling of request parameters in resource classes. By creating a parameter class that encapsulates all the components of a request, you can improve the organization, reusability, and clarity of your code. While it's not necessary for every situation, @BeanParam
is a powerful tool to have in your JAX-RS arsenal when handling complex requests.
For more information on JAX-RS and @BeanParam
, you can refer to the official JAX-RS 2.0 documentation and Java EE 7 Tutorial.