Handling Deprecated Features: Navigating from Java 7 to Java 8
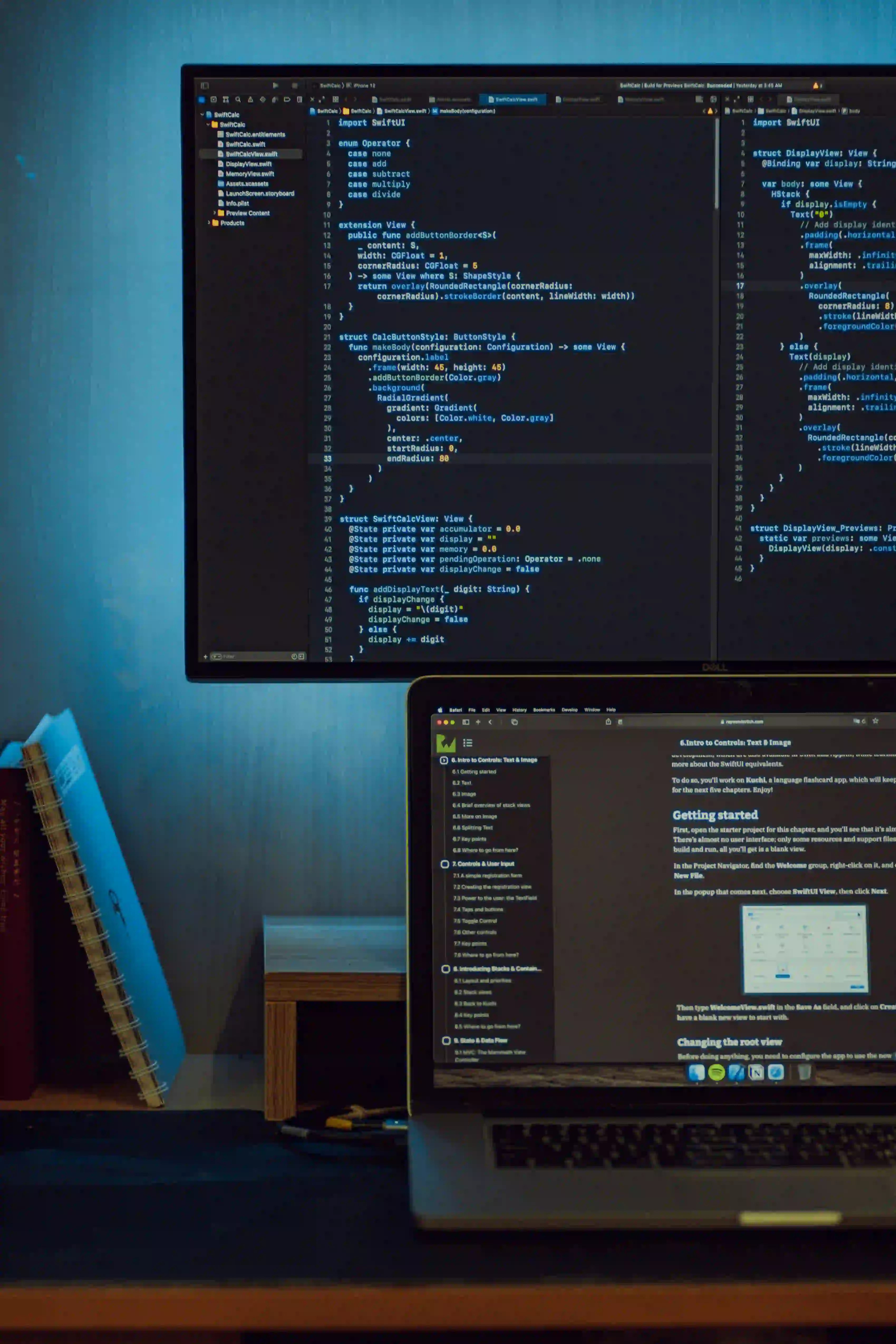
Navigating from Java 7 to Java 8: Handling Deprecated Features
Java 8 brought significant changes and enhancements to the Java programming language, providing developers with powerful tools and features to improve their code. However, as with any major update, there were also some deprecated features that required adjustments for developers migrating from Java 7 to Java 8.
In this article, we will explore some commonly deprecated features in Java 8 and discuss how to handle them effectively in your codebase.
Understanding Deprecated Features
Deprecated features in Java are marked for removal in future versions of the language. While they still work in the current version, they are no longer recommended for use and may cause issues or be completely removed in subsequent releases.
When migrating from Java 7 to Java 8, it's important to identify and address these deprecated features in your code to ensure compatibility and maintainability.
Handling Deprecated Features
1. Date and Time API
In Java 8, the new Date and Time API was introduced to address the shortcomings of the previous java.util.Date
and java.util.Calendar
classes. These older classes were prone to errors and not well-suited for modern date and time operations.
Deprecated Code (Java 7):
Date date = new Date();
Updated Code (Java 8):
LocalDate date = LocalDate.now();
The LocalDate
class from the new Date and Time API provides a more comprehensive and reliable way to work with dates in Java 8. By migrating to this new API, you can avoid the deprecated date and time classes and leverage the enhanced features provided by the updated API.
2. Stream API
Java 8 introduced the Stream API, offering a functional approach to process collections of objects. With the Stream API, developers can perform operations such as filtering, mapping, and reducing on data with concise and expressive syntax.
Deprecated Code (Java 7):
List<String> names = Arrays.asList("John", "Alice", "Bob");
for (String name : names) {
if (name.startsWith("A")) {
System.out.println(name);
}
}
Updated Code (Java 8):
List<String> names = Arrays.asList("John", "Alice", "Bob");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
By transitioning to the Stream API, you can simplify your code and take advantage of parallel processing for improved performance.
3. Functional Interfaces and Lambda Expressions
Java 8 introduced support for functional programming through lambda expressions and functional interfaces. This feature enables more concise and readable code by allowing developers to treat functionality as a method argument or code as data.
Deprecated Code (Java 7):
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Hello, World!");
}
};
Updated Code (Java 8):
Runnable runnable = () -> System.out.println("Hello, World!");
By utilizing lambda expressions, you can simplify code that requires functional interfaces and improve the readability of your Java 8 codebase.
The Closing Argument
Migrating from Java 7 to Java 8 involves addressing deprecated features and leveraging the new tools and capabilities introduced in the latest version. By understanding and handling deprecated features effectively, you can ensure a smooth transition to Java 8 while benefiting from its enhanced functionality.
In conclusion, while Java 8 deprecated certain features, it also introduced powerful new tools and enhancements that empower developers to write cleaner, more expressive code. By embracing these changes and adapting to the updated APIs and best practices, developers can make the most of the Java 8 ecosystem.
For further reading on Java 8's deprecated features and best practices for migration, I recommend checking out the official Java 8 documentation and the Java 8 Migration Guide. These resources provide comprehensive insights into handling deprecations and optimizing code for Java 8.