Solving Protractor Timeout Issues
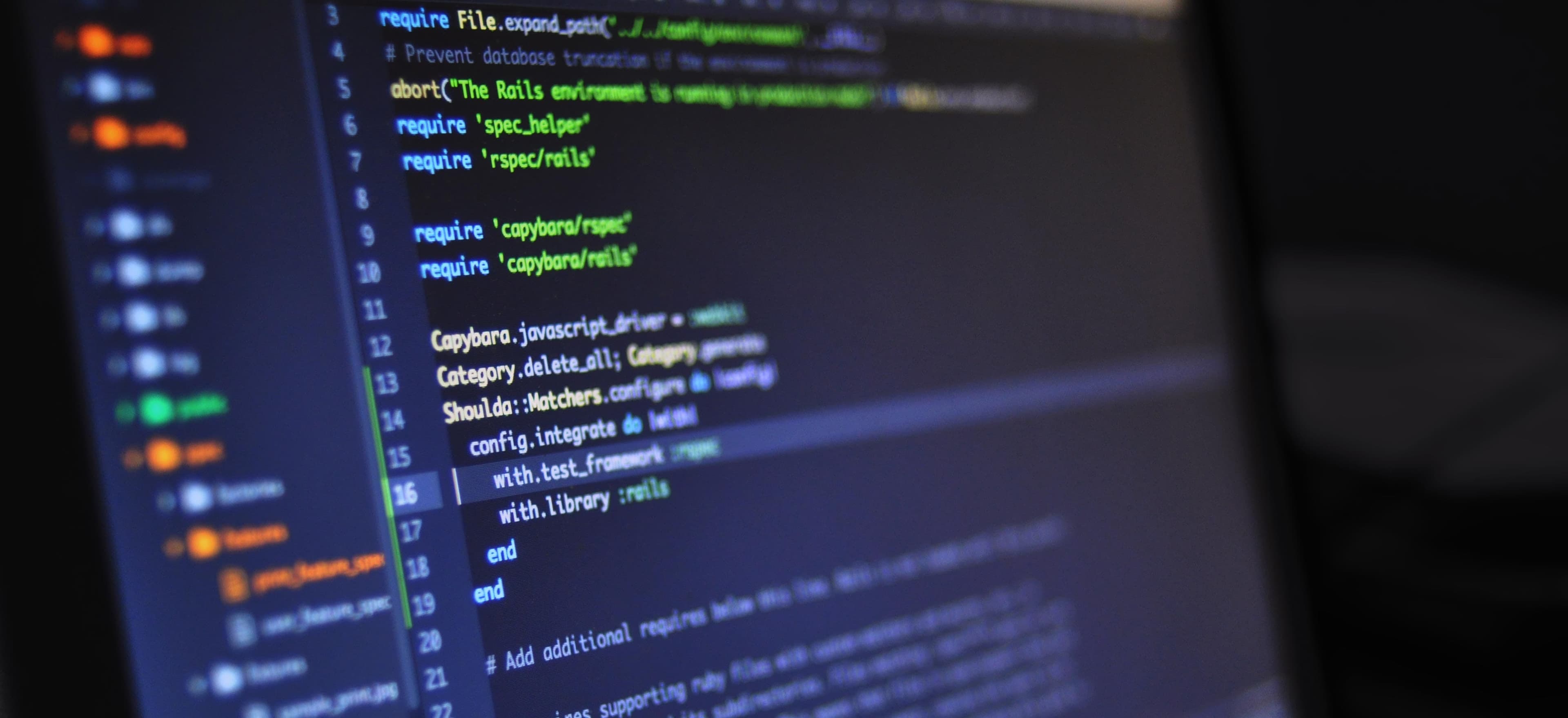
- Published on
Solving Protractor Timeout Issues
If you have worked with Protractor for automated testing in Angular applications, you might have encountered timeout issues. These issues can be frustrating, but fear not - there are strategies to address and solve them. In this article, we will discuss common timeout issues in Protractor and explore practical solutions to overcome them.
Understanding Timeout Issues in Protractor
Timeout issues in Protractor occur when the framework exceeds the maximum time allocated for a certain action. This can happen for various reasons, such as slow network conditions, heavy page load, or complex UI interactions. When Protractor encounters a timeout, it disrupts the test flow and may result in flaky or unreliable test results.
Common Timeout Scenarios
1. Waiting for Elements to Be Present
Consider the following scenario: You have a test that interacts with a button, but the button takes longer than expected to appear on the page. This delay can trigger a timeout issue in Protractor.
2. Asynchronous Operations
When dealing with asynchronous operations such as HTTP requests or animations, Protractor may struggle to synchronize with the application, leading to timeout problems.
Best Practices for Resolving Protractor Timeout Issues
1. Adjusting Protractor's Default Timeout
Protractor provides a default timeout configuration for various actions. By adjusting these timeouts, you can instruct Protractor to wait longer before considering an action as failed.
// protractor.conf.js
exports.config = {
...
jasmineNodeOpts: {
defaultTimeoutInterval: 60000 // Set the default timeout to 60 seconds
},
...
};
Increasing the default timeout can provide more leeway for elements to load or operations to complete, reducing the likelihood of timeouts.
2. Using Expected Conditions
Protractor offers the ExpectedConditions
class, which provides a set of predefined conditions for waiting on elements. These conditions include presenceOf
, visibilityOf
, and invisibilityOf
, among others. By using these conditions, you can explicitly define what Protractor should wait for before proceeding with the test.
const EC = protractor.ExpectedConditions;
const button = element(by.id('myButton'));
browser.wait(EC.visibilityOf(button), 5000, 'Button is not visible');
By specifying expected conditions, you can ensure that Protractor waits for the necessary elements or states before proceeding, reducing the risk of timeouts.
3. Handling Asynchronous Operations
When dealing with asynchronous operations, such as HTTP requests or animations, it's crucial to synchronize Protractor with the application. Using async/await
or Promise
handling can help ensure that Protractor waits for these operations to complete before advancing the test.
it('should wait for asynchronous operation', async () => {
await performAsyncOperation();
// Continue with test assertions
});
By properly handling asynchronous operations, you can mitigate timeout issues caused by synchronization conflicts.
4. Analyzing Network Conditions
In some cases, timeout issues in Protractor may stem from poor network conditions or slow server responses. It's essential to analyze and optimize the network performance to minimize the impact of these external factors on test execution.
A Final Look
Protractor timeout issues can be challenging, but with the right strategies, you can effectively address and solve them. By understanding the root causes of timeout problems and implementing best practices such as adjusting timeouts, using expected conditions, handling asynchronous operations, and optimizing network conditions, you can enhance the stability and reliability of your Protractor tests.
Remember, proactive timeout management is key to maintaining robust and dependable test suites in your Angular applications. With these strategies in place, you can mitigate timeout issues and ensure smooth execution of your Protractor tests.
For further detailed information and advanced strategies regarding Protractor timeout issues, consider exploring the official Protractor documentation!