Validating User Input in JavaFX with Validation Constraints
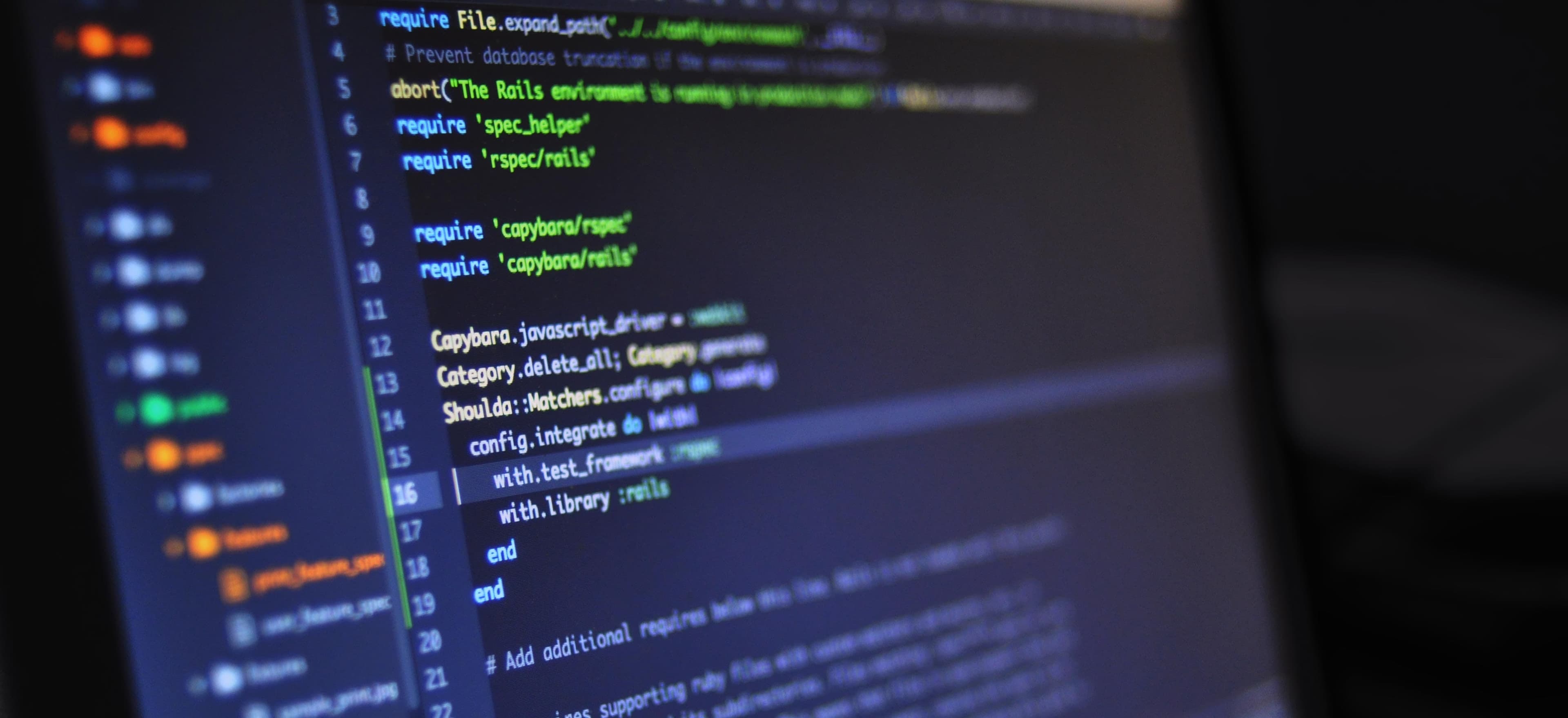
- Published on
Validating User Input in JavaFX with Validation Constraints
When developing a JavaFX application, ensuring that user input is validated is a crucial aspect of creating a robust and user-friendly software. By implementing validation constraints, developers can prevent erroneous data from entering the system, ultimately enhancing the overall user experience and data integrity. In this blog post, we'll delve into the process of validating user input in JavaFX by utilizing validation constraints.
Understanding Validation Constraints
Validation constraints are rules and conditions that are applied to user input to ensure its correctness and conformity with predefined criteria. These constraints define the permissible data formats, ranges, and other conditions that the input must satisfy. In JavaFX, validation constraints are often implemented using the Java Bean Validation (JSR 380) framework, which provides a set of annotations for defining constraints on Java objects.
Setting up the JavaFX Project
Let's begin by setting up a simple JavaFX project. First, make sure you have Java and JavaFX installed on your system. If not, you can follow the official guides for installing Java and JavaFX.
Once everything is set up, create a new JavaFX project in your preferred IDE and add the necessary dependencies for Java Bean Validation. You can include the validation API in your project by adding the following Maven dependency:
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
<version>2.0.1.Final</version>
</dependency>
Creating a Form for User Input
Next, let's create a simple form in our JavaFX application that captures user input. For demonstration purposes, we'll create a form to capture user registration details, including username, email, and password.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class UserRegistrationForm extends Application {
@Override
public void start(Stage primaryStage) {
VBox root = new VBox(10);
TextField usernameField = new TextField();
TextField emailField = new TextField();
PasswordField passwordField = new PasswordField();
Button submitButton = new Button("Submit");
root.getChildren().addAll(
new Label("Username"),
usernameField,
new Label("Email"),
emailField,
new Label("Password"),
passwordField,
submitButton
);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("User Registration");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Applying Validation Constraints
Now that we have our form set up, let's apply validation constraints to the user input fields. We'll use the Java Bean Validation annotations to define the constraints. In this example, we'll ensure that the username is not empty, the email is a valid email address, and the password meets certain criteria.
import javax.validation.constraints.*;
public class User {
@NotBlank(message = "Username is required")
private String username;
@Email(message = "Invalid email address")
private String email;
@Size(min = 8, message = "Password must be at least 8 characters long")
private String password;
// Getters and setters
}
In the User
class, we've applied the @NotBlank
, @Email
, and @Size
annotations to the username
, email
, and password
fields, respectively. These annotations define the validation constraints for each field.
Integrating Validation into the JavaFX Application
To integrate the validation constraints into our JavaFX application, we'll need to handle the form submission and validate the user input. We can use the Validator
class from the Java Bean Validation API to perform the validation.
import javax.validation.*;
import javafx.scene.control.Alert;
public class UserRegistrationForm extends Application {
private Validator validator;
@Override
public void start(Stage primaryStage) {
// Form setup code (previous code snippet)
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
submitButton.setOnAction(event -> {
User user = new User();
user.setUsername(usernameField.getText());
user.setEmail(emailField.getText());
user.setPassword(passwordField.getText());
Set<ConstraintViolation<User>> violations = validator.validate(user);
if (!violations.isEmpty()) {
StringBuilder message = new StringBuilder();
for (ConstraintViolation<User> violation : violations) {
message.append(violation.getMessage()).append("\n");
}
showErrorAlert("Validation Error", message.toString());
} else {
// Process the validated user input
// ...
}
});
}
private void showErrorAlert(String title, String message) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setContentText(message);
alert.showAndWait();
}
// main method and other helper methods (previous code snippets)
}
In the start
method of the UserRegistrationForm
class, we initialize the Validator
using a ValidatorFactory
. When the user clicks the submit button, we create a User
object with the input data and validate it using the validate
method of the Validator
. If any validation constraints are violated, we display an error alert with the corresponding messages. Otherwise, we can proceed with processing the validated user input.
My Closing Thoughts on the Matter
In this blog post, we've explored the process of validating user input in a JavaFX application using validation constraints. By applying Java Bean Validation annotations and integrating the validation process into the application, we can ensure that the user input meets the specified criteria, leading to a more robust and reliable software system.
Validating user input is a critical aspect of application development, and JavaFX provides the necessary tools and frameworks, such as Java Bean Validation, to streamline the validation process and enhance the overall user experience.
Implementing validation constraints not only improves data integrity but also contributes to the creation of user-friendly and professional applications. By effectively validating user input, developers can mitigate potential errors and ensure that the software functions as intended, providing a seamless and reliable experience for the end users.
Incorporating validation constraints into JavaFX applications is a best practice that all developers should prioritize, as it ultimately leads to the development of high-quality, dependable software.
In conclusion, the integration of validation constraints in JavaFX applications is essential for maintaining data consistency and enhancing the overall user experience, ultimately contributing to the creation of robust and reliable software.
By following the guidelines and examples provided in this blog post, developers can effectively implement validation constraints in their JavaFX applications, thereby ensuring the integrity of user input and the overall quality of the software.