Understanding the Role of Annotations in Java Programming
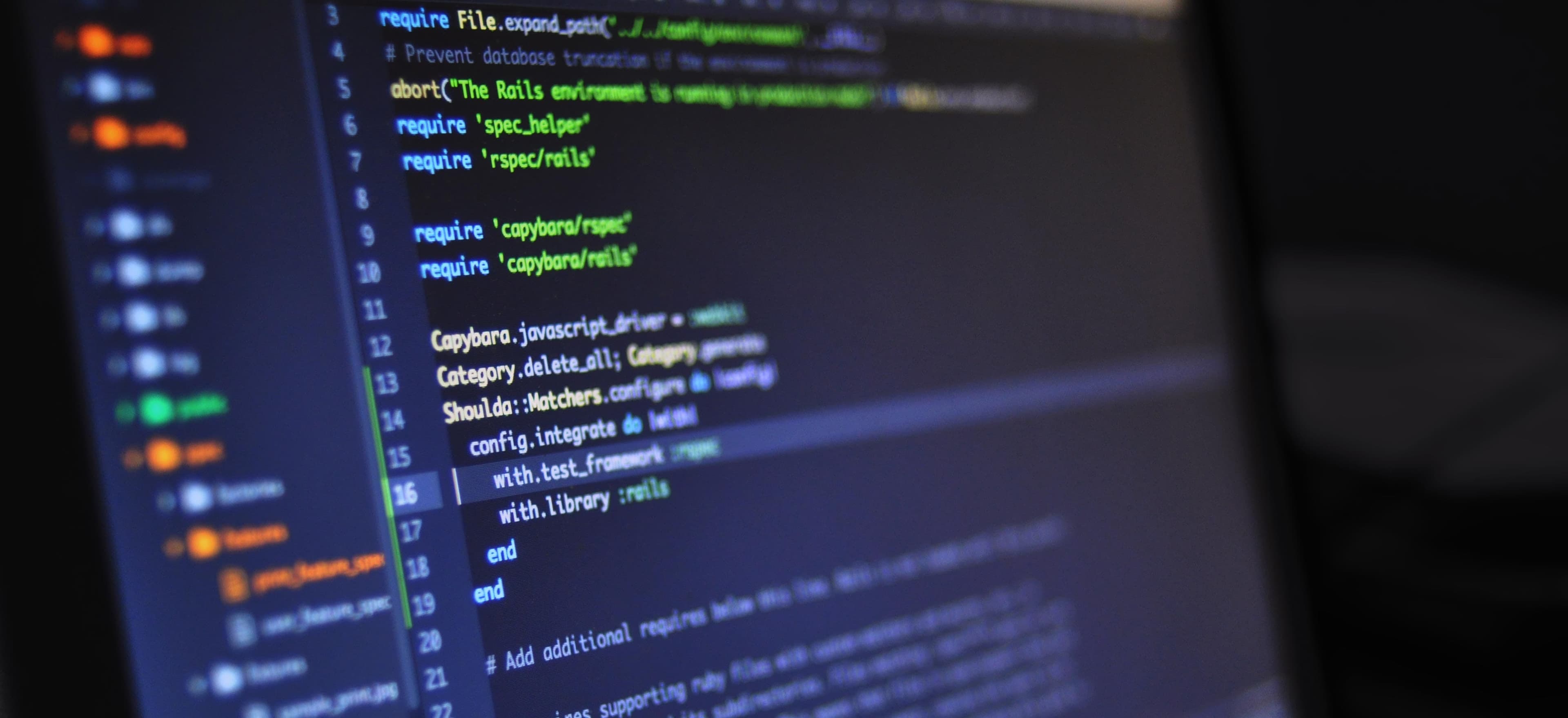
- Published on
In the world of Java programming, annotations play a crucial role in providing metadata about the code. They offer a way to add meta-information to your Java code, making it more understandable and efficient. In this blog post, we will delve into the concept of annotations in Java, examining their significance, how to use them, and their real-world applications.
What are Annotations?
Annotations, introduced in Java 5, are a form of metadata that provides information about the code. They do not directly affect the execution of the code, but they can be used by the compiler, development tools, or at runtime to generate or modify the code. Annotations are defined using the @
symbol, followed by the annotation interface.
Using Built-in Annotations
Java provides a set of built-in annotations that serve various purposes:
@Override
The @Override
annotation informs the compiler that the annotated method is intended to override a method in a superclass. It helps catch errors at compile time if the annotated method doesn't actually override a method in the superclass.
@Override
public void someMethod() {
// Method implementation
}
@Deprecated
The @Deprecated
annotation indicates that the annotated element is deprecated and should no longer be used. It serves as a warning to developers, encouraging them to use an alternative approach.
@Deprecated
public void oldMethod() {
// Deprecated method implementation
}
@SuppressWarnings
The @SuppressWarnings
annotation tells the compiler to suppress specific warnings that the annotated element might trigger. It is often used to maintain compatibility with older code.
@SuppressWarnings("unchecked")
List<String> items = new ArrayList();
Creating Custom Annotations
While using built-in annotations is valuable, creating custom annotations allows developers to define metadata specific to their applications or frameworks. Custom annotations are defined using the @interface
keyword.
Example: Creating a Custom Annotation
Let's create a custom annotation @Initiate
that marks a method to be initiated.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Initiate {
// Optional elements can be added here
}
In this example, the @Initiate
annotation is defined to be retained at runtime and can be applied only to methods.
Using the Custom Annotation
Next, let's apply the @Initiate
custom annotation to a method.
public class MyClass {
@Initiate
public void initialize() {
// Initialization code
}
}
Real-world Applications
Annotations find extensive use in frameworks and libraries to enhance the functionality and usability of code.
Spring Framework
In the Spring framework, annotations such as @Autowired
, @RestController
, and @Service
simplify configuration and dependency injection, reducing the boilerplate code.
@Service
public class MyService {
// Service implementation
}
Hibernate ORM
Hibernate ORM uses annotations like @Entity
, @Table
, and @Column
to map Java classes to database tables, making it easier to define the persistence layer.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue
@Column(name = "user_id")
private Long id;
// Other fields and methods
}
Best Practices for Using Annotations
When using annotations in Java, it's essential to adhere to best practices to maintain clean and readable code:
- Use built-in annotations whenever possible to leverage standard practices and improve code understanding.
- Document the custom annotations thoroughly to explain their purpose and usage.
- Avoid excessive or unnecessary use of annotations, as they can clutter the code and make it harder to maintain.
Final Considerations
Annotations in Java play a pivotal role in simplifying development tasks, enhancing code readability, and enabling powerful frameworks and libraries. By understanding the significance of built-in annotations, creating custom annotations, and applying them in real-world scenarios, Java developers can leverage the full potential of annotations to write efficient, maintainable, and scalable code.