Handling Session Timeouts in JSF: Best Practices
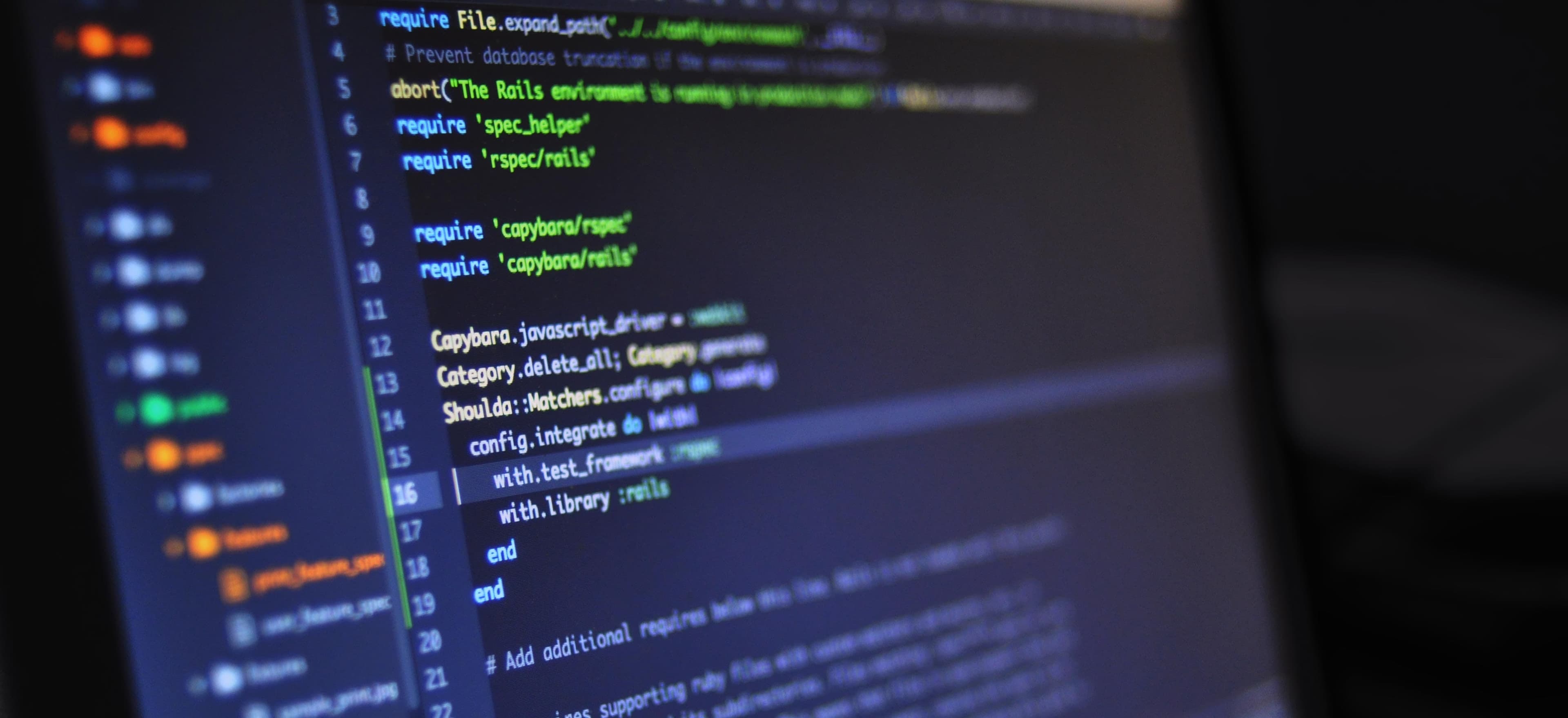
- Published on
Handling Session Timeouts in JavaServer Faces (JSF): Best Practices
When developing web applications with JavaServer Faces (JSF), managing session timeouts is a crucial aspect to consider. Session timeouts occur when a user's session on the server expires due to inactivity, which necessitates handling to provide a seamless user experience. In this article, we will explore the best practices for handling session timeouts in JSF applications.
Understanding Session Timeout
In a web application, a session is created when a user interacts with the server. This session has a predetermined lifespan, after which it expires due to inactivity, often causing loss of user data or disrupting the workflow. Therefore, it is essential to handle session timeouts effectively to maintain the application's usability and security.
Configuring Session Timeout in web.xml
In a JSF application, the session timeout can be configured in the web.xml
deployment descriptor. This configuration specifies the duration of inactivity after which a session will expire. Below is an example of how to set the session timeout to 30 minutes:
<session-config>
<session-timeout>30</session-timeout>
</session-config>
By setting the session timeout appropriately, you can ensure that sessions do not persist indefinitely, thereby enhancing the security of your application.
Notifying Users About Session Expiry
To provide a seamless user experience, it is imperative to notify users when their session is about to expire. This can be achieved by displaying a warning message or modal dialog before the session expires. Utilizing JavaScript, you can create timers that trigger notifications to users when their session is nearing expiration.
import javax.faces.application.FacesMessage;
import javax.faces.context.FacesContext;
public void checkSessionExpiry() {
// Code to check if the session is about to expire
if (sessionAboutToExpire) {
FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_WARN, "Warning", "Your session is about to expire. Please save your work."));
}
}
By implementing such a mechanism, you can effectively communicate with users before their sessions expire, allowing them to take appropriate action to prevent data loss.
Handling Session Timeout Redirects
When a session expires, it is advisable to redirect the user to a designated page, such as a login or session expired page, instead of leaving them on a potentially broken or insecure state within the application. To achieve this, you can employ the web.xml
configuration to specify the page to which the user should be redirected upon session expiration.
<error-page>
<exception-type>javax.faces.application.ViewExpiredException</exception-type>
<location>/sessionExpired.xhtml</location>
</error-page>
By defining the error page for ViewExpiredException
, you ensure that users are redirected to a specific page when their sessions expire, further enhancing the user experience and security of your application.
Handling Session Timeout Programmatically
In some scenarios, you may need to handle session timeouts programmatically, such as when invoking specific actions upon session expiration. JSF provides a convenient way to achieve this through the use of a PhaseListener
, which allows you to execute custom logic based on the JSF lifecycle phases.
import javax.faces.event.PhaseEvent;
import javax.faces.event.PhaseId;
import javax.faces.event.PhaseListener;
public class SessionTimeoutHandler implements PhaseListener {
@Override
public void afterPhase(PhaseEvent event) {
// Code to handle session timeout
}
@Override
public void beforePhase(PhaseEvent event) {
// Code to handle pre-phase actions
}
@Override
public PhaseId getPhaseId() {
return PhaseId.ANY;
}
}
By implementing a PhaseListener
, you can intercept the JSF lifecycle and execute custom logic to handle session timeouts as needed, providing greater control and flexibility in managing sessions within your application.
In Conclusion, Here is What Matters
In conclusion, effectively managing session timeouts in JSF applications is vital for ensuring a seamless user experience and maintaining the security of the application. By configuring session timeouts, notifying users about session expiry, handling redirects, and programmatically managing session expiration, you can enhance the usability and reliability of your JSF web application.
Incorporating these best practices into your JSF development workflow will not only benefit the end users but also contribute to the overall robustness of your web application.