How to Effectively Use Spring Data Mock DAO
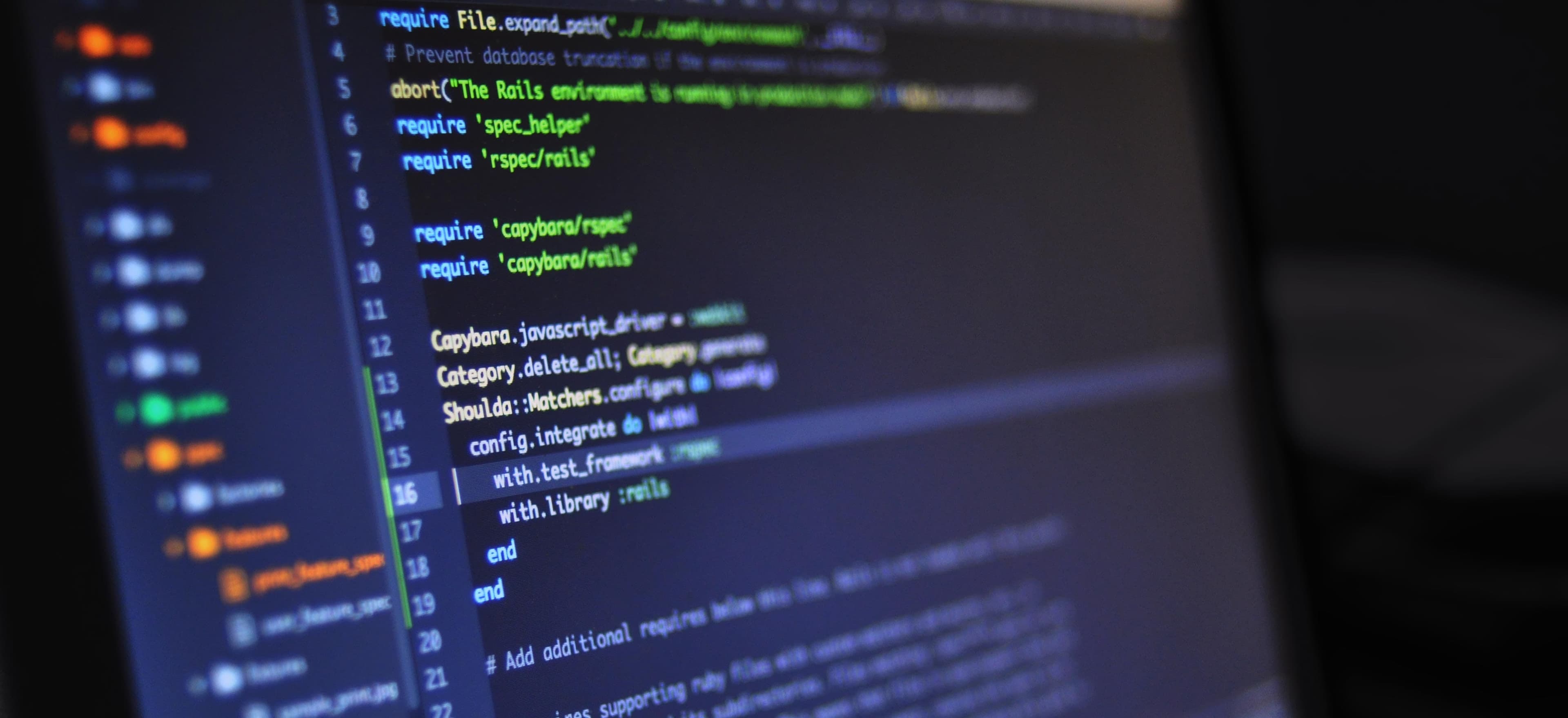
- Published on
How to Effectively Use Spring Data Mock DAO
When it comes to testing your data access layer in a Spring application, using a mock DAO can be a powerful tool. Mocking DAOs allows you to isolate the data access code and test it independently from the rest of your application. In this article, we'll explore how to effectively use Spring Data mock DAO for testing.
Why Use Spring Data Mock DAO?
Testing the data access layer is crucial to ensure that your application operates correctly and efficiently. By using mock DAOs, you can simulate the behavior of your data access code without actually interacting with the database. This allows for faster and more focused testing, as well as the ability to control the behavior of the DAO to test various scenarios.
Setting Up Your Project
Assuming you have a Spring application with Spring Data JPA, you can easily set up mock DAOs for testing using the following dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
These dependencies provide the necessary tools for setting up mock DAOs and writing test cases for your data access layer.
Creating a Mock DAO
To create a mock DAO for your Spring Data JPA repository, you can use the @MockBean
annotation provided by the Spring Test framework. This annotation allows you to mock a bean or a component within the Spring context. Here's an example of how to create a mock DAO for a repository called UserRepository
:
import org.springframework.boot.test.mock.mockito.MockBean;
@MockBean
private UserRepository userRepository;
By annotating a field with @MockBean
, Spring Boot will automatically create a mock instance of UserRepository
and inject it into the application context for testing.
Writing Test Cases
Once you have set up the mock DAO, you can start writing test cases for your data access layer. Here's an example of how to write a test case using JUnit and Mockito:
import static org.mockito.Mockito.when;
@Test
void testFindUserById() {
// Arrange
User expectedUser = new User(1L, "john.doe@example.com");
when(userRepository.findById(1L)).thenReturn(Optional.of(expectedUser));
// Act
Optional<User> actualUser = userRepository.findById(1L);
// Assert
assertTrue(actualUser.isPresent());
assertEquals(expectedUser.getEmail(), actualUser.get().getEmail());
}
In this example, we use Mockito to mock the behavior of the userRepository
and specify its behavior when the findById
method is called with a specific id. We then perform the method invocation and assert the expected outcome.
Benefits of Using Spring Data Mock DAO
Using Spring Data mock DAO offers several benefits:
- Isolation: Mocking DAOs allows you to isolate the data access layer from the rest of the application, making it easier to pinpoint issues and write focused test cases.
- Controlled Behavior: You can control the behavior of the mock DAO to simulate various scenarios, such as successful data retrieval, error handling, and edge cases.
- Speed: Testing with mock DAOs is generally faster than interacting with a real database, especially when dealing with large datasets or complex queries.
- Flexibility: You can easily modify the behavior of the mock DAO without changing the actual data in the database, making it ideal for iterative testing and development.
Closing Remarks
In conclusion, using Spring Data mock DAO for testing your data access layer in a Spring application offers a range of benefits, including isolation, controlled behavior, speed, and flexibility. By effectively leveraging mock DAOs, you can ensure the reliability and robustness of your data access code while streamlining the testing process.
By following the techniques outlined in this article, you can confidently test your data access layer using Spring Data mock DAO and write comprehensive test cases to validate the behavior of your DAO code.
Now that you understand the power of Spring Data mock DAO, it's time to apply these techniques in your own projects and elevate the quality and reliability of your data access layer testing. Happy coding!
Remember that for additional information on Spring Data and Mockito, you can refer to the official documentation provided by Spring Data and Mockito.
Checkout our other articles