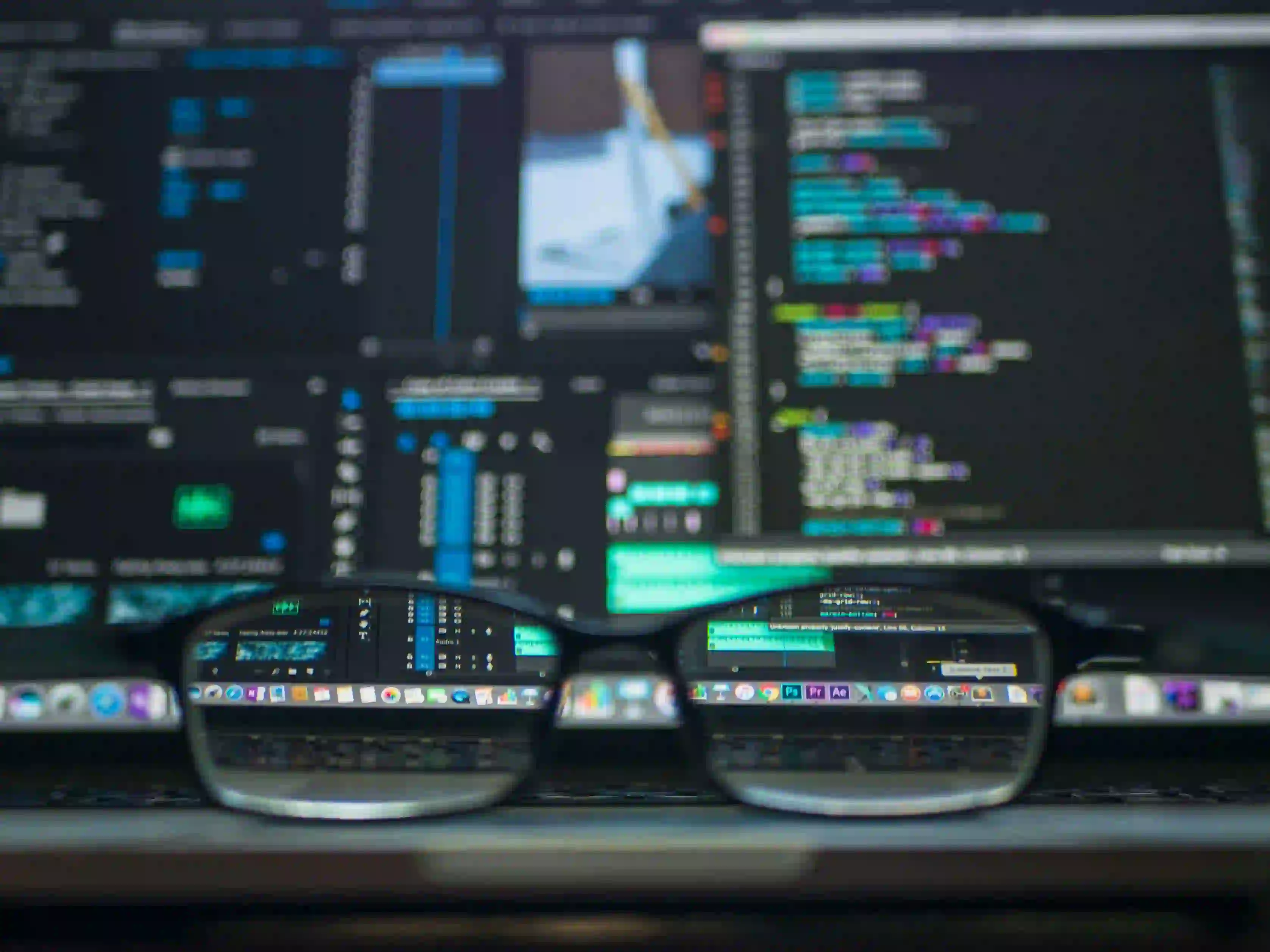
Implementing Template Method Pattern with Java Generics
In this post, we'll explore how to implement the Template Method Pattern in Java using Generics. The Template Method Pattern is a behavioral design pattern that defines the skeleton of an algorithm in a method, deferring some steps to subclasses. Generics allow us to create classes, interfaces, and methods that operate on objects of specified types, providing type safety and reducing the need for explicit type casting.
Understanding the Template Method Pattern
The Template Method Pattern is based on defining an algorithm in a superclass with some of the steps being deferred to subclasses. This pattern is useful when there are multiple similar algorithms with some varying steps. By providing a template method that outlines the algorithm's structure, we can let subclasses implement the varying steps, keeping the algorithm intact while allowing for customization.
Creating the Abstract Template Class
Let's begin by creating an abstract class that serves as the template for our algorithm, using Java generics to allow for flexibility in types. We will define the template method and leave some steps to be implemented by subclasses.
public abstract class DataProcessor<T> {
// Template method
public void process(T data) {
T preProcessedData = preprocess(data);
T processedData = processData(preProcessedData);
displayData(processedData);
}
// Abstract methods to be implemented by subclasses
protected abstract T preprocess(T data);
protected abstract T processData(T data);
protected abstract void displayData(T data);
}
In the above code, the DataProcessor
class is defined with a type parameter T
. The process
method outlines the algorithm's structure, while the preprocess
, processData
, and displayData
methods are left abstract for implementation by subclasses.
Implementing Concrete Subclasses
Now, let's create concrete subclasses that extend the DataProcessor
class and provide implementations for the abstract methods.
public class IntegerDataProcessor extends DataProcessor<Integer> {
@Override
protected Integer preprocess(Integer data) {
// Preprocessing logic for integer data
return data * 2;
}
@Override
protected Integer processData(Integer data) {
// Processing logic for integer data
return data + 5;
}
@Override
protected void displayData(Integer data) {
// Displaying integer data
System.out.println("Processed integer data: " + data);
}
}
In this example, we've created a IntegerDataProcessor
class that specializes the DataProcessor
for integer data. We have provided implementations for the preprocessing, processing, and displaying of integer data. This allows us to customize the steps of the algorithm while reusing the template method defined in the superclass.
Utilizing the Concrete Subclasses
We can now utilize the concrete subclasses to process data using the template method pattern.
public class Main {
public static void main(String[] args) {
DataProcessor<Integer> integerProcessor = new IntegerDataProcessor();
integerProcessor.process(10);
}
}
In this example, an instance of IntegerDataProcessor
is created and used to process integer data. The process
method from the superclass is called, which in turn executes the template method, including the customized steps implemented in the concrete subclass.
Benefits of Using Generics with Template Method Pattern
Using generics with the Template Method Pattern provides several benefits:
-
Increased Flexibility: Generics allow us to define the type of data to be processed, enabling flexibility in the types of data that can be handled by the template method pattern.
-
Type Safety: By using generics, we can ensure type safety throughout the implementation, reducing the risk of type-related errors at compile-time.
-
Code Reusability: The use of generics allows for the creation of a single template method that can be reused with different data types, promoting code reusability.
-
Clearer API: With generics, the template class provides a clearer API by specifying the expected data type, making the class more self-explanatory and easier to use.
Final Thoughts
In this post, we have explored how to implement the Template Method Pattern in Java using Generics. We created an abstract template class using generics and defined the template method, leaving some steps to be implemented by concrete subclasses. By utilizing Java generics, we were able to achieve flexibility, type safety, and code reusability when implementing the Template Method Pattern.
Implementing the Template Method Pattern with Java generics provides a powerful way to define algorithms with varying steps, while allowing for customization and reuse across different data types.
Check out the Oracle Java Generics documentation for a deeper understanding of Java generics and their usage.